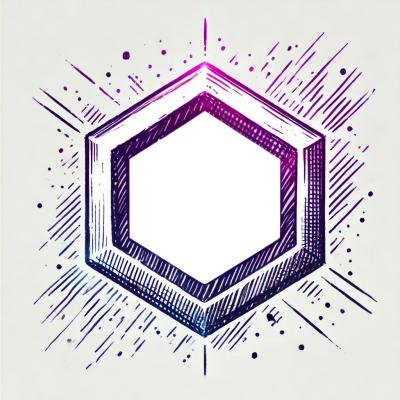
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
OryClient - the Ruby gem for the Ory APIs
Documentation for all public and administrative Ory APIs. Administrative APIs can only be accessed with a valid Personal Access Token. Public APIs are mostly used in browsers.
This document describes the APIs available in the Ory Network. The APIs are available as SDKs for the following languages:
Language | Download SDK | Documentation |
---|---|---|
Dart | pub.dev | README |
.NET | nuget.org | README |
Elixir | hex.pm | README |
Go | github.com | README |
Java | maven.org | README |
JavaScript | npmjs.com | README |
JavaScript (With fetch) | npmjs.com | README |
PHP | packagist.org | README |
Python | pypi.org | README |
Ruby | rubygems.org | README |
Rust | crates.io | README |
This SDK is automatically generated by the OpenAPI Generator project:
To build the Ruby code into a gem:
gem build ory-client.gemspec
Then either install the gem locally:
gem install ./ory-client-v1.16.6.gem
(for development, run gem install --dev ./ory-client-v1.16.6.gem
to install the development dependencies)
or publish the gem to a gem hosting service, e.g. RubyGems.
Finally add this to the Gemfile:
gem 'ory-client', '~> v1.16.6'
If the Ruby gem is hosted at a git repository: https://github.com/ory/sdk, then add the following in the Gemfile:
gem 'ory-client', :git => 'https://github.com/ory/sdk.git'
Include the Ruby code directly using -I
as follows:
ruby -Ilib script.rb
Please follow the installation procedure and then run the following code:
# Load the gem
require 'ory-client'
# Setup authorization
OryClient.configure do |config|
# Configure Bearer authorization: oryAccessToken
config.access_token = 'YOUR_BEARER_TOKEN'
# Configure a proc to get access tokens in lieu of the static access_token configuration
config.access_token_getter = -> { 'YOUR TOKEN GETTER PROC' }
end
api_instance = OryClient::CourierApi.new
id = 'id_example' # String | MessageID is the ID of the message.
begin
#Get a Message
result = api_instance.get_courier_message(id)
p result
rescue OryClient::ApiError => e
puts "Exception when calling CourierApi->get_courier_message: #{e}"
end
All URIs are relative to https://playground.projects.oryapis.com
Class | Method | HTTP request | Description |
---|---|---|---|
OryClient::CourierApi | get_courier_message | GET /admin/courier/messages/{id} | Get a Message |
OryClient::CourierApi | list_courier_messages | GET /admin/courier/messages | List Messages |
OryClient::EventsApi | create_event_stream | POST /projects/{project_id}/eventstreams | Create an event stream for your project. |
OryClient::EventsApi | delete_event_stream | DELETE /projects/{project_id}/eventstreams/{event_stream_id} | Remove an event stream from a project |
OryClient::EventsApi | list_event_streams | GET /projects/{project_id}/eventstreams | List all event streams for the project. This endpoint is not paginated. |
OryClient::EventsApi | set_event_stream | PUT /projects/{project_id}/eventstreams/{event_stream_id} | Update an event stream for a project. |
OryClient::FrontendApi | create_browser_login_flow | GET /self-service/login/browser | Create Login Flow for Browsers |
OryClient::FrontendApi | create_browser_logout_flow | GET /self-service/logout/browser | Create a Logout URL for Browsers |
OryClient::FrontendApi | create_browser_recovery_flow | GET /self-service/recovery/browser | Create Recovery Flow for Browsers |
OryClient::FrontendApi | create_browser_registration_flow | GET /self-service/registration/browser | Create Registration Flow for Browsers |
OryClient::FrontendApi | create_browser_settings_flow | GET /self-service/settings/browser | Create Settings Flow for Browsers |
OryClient::FrontendApi | create_browser_verification_flow | GET /self-service/verification/browser | Create Verification Flow for Browser Clients |
OryClient::FrontendApi | create_fedcm_flow | GET /self-service/fed-cm/parameters | Get FedCM Parameters |
OryClient::FrontendApi | create_native_login_flow | GET /self-service/login/api | Create Login Flow for Native Apps |
OryClient::FrontendApi | create_native_recovery_flow | GET /self-service/recovery/api | Create Recovery Flow for Native Apps |
OryClient::FrontendApi | create_native_registration_flow | GET /self-service/registration/api | Create Registration Flow for Native Apps |
OryClient::FrontendApi | create_native_settings_flow | GET /self-service/settings/api | Create Settings Flow for Native Apps |
OryClient::FrontendApi | create_native_verification_flow | GET /self-service/verification/api | Create Verification Flow for Native Apps |
OryClient::FrontendApi | disable_my_other_sessions | DELETE /sessions | Disable my other sessions |
OryClient::FrontendApi | disable_my_session | DELETE /sessions/{id} | Disable one of my sessions |
OryClient::FrontendApi | exchange_session_token | GET /sessions/token-exchange | Exchange Session Token |
OryClient::FrontendApi | get_flow_error | GET /self-service/errors | Get User-Flow Errors |
OryClient::FrontendApi | get_login_flow | GET /self-service/login/flows | Get Login Flow |
OryClient::FrontendApi | get_recovery_flow | GET /self-service/recovery/flows | Get Recovery Flow |
OryClient::FrontendApi | get_registration_flow | GET /self-service/registration/flows | Get Registration Flow |
OryClient::FrontendApi | get_settings_flow | GET /self-service/settings/flows | Get Settings Flow |
OryClient::FrontendApi | get_verification_flow | GET /self-service/verification/flows | Get Verification Flow |
OryClient::FrontendApi | get_web_authn_java_script | GET /.well-known/ory/webauthn.js | Get WebAuthn JavaScript |
OryClient::FrontendApi | list_my_sessions | GET /sessions | Get My Active Sessions |
OryClient::FrontendApi | perform_native_logout | DELETE /self-service/logout/api | Perform Logout for Native Apps |
OryClient::FrontendApi | to_session | GET /sessions/whoami | Check Who the Current HTTP Session Belongs To |
OryClient::FrontendApi | update_fedcm_flow | POST /self-service/fed-cm/token | Submit a FedCM token |
OryClient::FrontendApi | update_login_flow | POST /self-service/login | Submit a Login Flow |
OryClient::FrontendApi | update_logout_flow | GET /self-service/logout | Update Logout Flow |
OryClient::FrontendApi | update_recovery_flow | POST /self-service/recovery | Update Recovery Flow |
OryClient::FrontendApi | update_registration_flow | POST /self-service/registration | Update Registration Flow |
OryClient::FrontendApi | update_settings_flow | POST /self-service/settings | Complete Settings Flow |
OryClient::FrontendApi | update_verification_flow | POST /self-service/verification | Complete Verification Flow |
OryClient::IdentityApi | batch_patch_identities | PATCH /admin/identities | Create multiple identities |
OryClient::IdentityApi | create_identity | POST /admin/identities | Create an Identity |
OryClient::IdentityApi | create_recovery_code_for_identity | POST /admin/recovery/code | Create a Recovery Code |
OryClient::IdentityApi | create_recovery_link_for_identity | POST /admin/recovery/link | Create a Recovery Link |
OryClient::IdentityApi | delete_identity | DELETE /admin/identities/{id} | Delete an Identity |
OryClient::IdentityApi | delete_identity_credentials | DELETE /admin/identities/{id}/credentials/{type} | Delete a credential for a specific identity |
OryClient::IdentityApi | delete_identity_sessions | DELETE /admin/identities/{id}/sessions | Delete & Invalidate an Identity's Sessions |
OryClient::IdentityApi | disable_session | DELETE /admin/sessions/{id} | Deactivate a Session |
OryClient::IdentityApi | extend_session | PATCH /admin/sessions/{id}/extend | Extend a Session |
OryClient::IdentityApi | get_identity | GET /admin/identities/{id} | Get an Identity |
OryClient::IdentityApi | get_identity_schema | GET /schemas/{id} | Get Identity JSON Schema |
OryClient::IdentityApi | get_session | GET /admin/sessions/{id} | Get Session |
OryClient::IdentityApi | list_identities | GET /admin/identities | List Identities |
OryClient::IdentityApi | list_identity_schemas | GET /schemas | Get all Identity Schemas |
OryClient::IdentityApi | list_identity_sessions | GET /admin/identities/{id}/sessions | List an Identity's Sessions |
OryClient::IdentityApi | list_sessions | GET /admin/sessions | List All Sessions |
OryClient::IdentityApi | patch_identity | PATCH /admin/identities/{id} | Patch an Identity |
OryClient::IdentityApi | update_identity | PUT /admin/identities/{id} | Update an Identity |
OryClient::JwkApi | create_json_web_key_set | POST /admin/keys/{set} | Create JSON Web Key |
OryClient::JwkApi | delete_json_web_key | DELETE /admin/keys/{set}/{kid} | Delete JSON Web Key |
OryClient::JwkApi | delete_json_web_key_set | DELETE /admin/keys/{set} | Delete JSON Web Key Set |
OryClient::JwkApi | get_json_web_key | GET /admin/keys/{set}/{kid} | Get JSON Web Key |
OryClient::JwkApi | get_json_web_key_set | GET /admin/keys/{set} | Retrieve a JSON Web Key Set |
OryClient::JwkApi | set_json_web_key | PUT /admin/keys/{set}/{kid} | Set JSON Web Key |
OryClient::JwkApi | set_json_web_key_set | PUT /admin/keys/{set} | Update a JSON Web Key Set |
OryClient::MetadataApi | get_version | GET /version | Return Running Software Version. |
OryClient::OAuth2Api | accept_o_auth2_consent_request | PUT /admin/oauth2/auth/requests/consent/accept | Accept OAuth 2.0 Consent Request |
OryClient::OAuth2Api | accept_o_auth2_login_request | PUT /admin/oauth2/auth/requests/login/accept | Accept OAuth 2.0 Login Request |
OryClient::OAuth2Api | accept_o_auth2_logout_request | PUT /admin/oauth2/auth/requests/logout/accept | Accept OAuth 2.0 Session Logout Request |
OryClient::OAuth2Api | create_o_auth2_client | POST /admin/clients | Create OAuth 2.0 Client |
OryClient::OAuth2Api | delete_o_auth2_client | DELETE /admin/clients/{id} | Delete OAuth 2.0 Client |
OryClient::OAuth2Api | delete_o_auth2_token | DELETE /admin/oauth2/tokens | Delete OAuth 2.0 Access Tokens from specific OAuth 2.0 Client |
OryClient::OAuth2Api | delete_trusted_o_auth2_jwt_grant_issuer | DELETE /admin/trust/grants/jwt-bearer/issuers/{id} | Delete Trusted OAuth2 JWT Bearer Grant Type Issuer |
OryClient::OAuth2Api | get_o_auth2_client | GET /admin/clients/{id} | Get an OAuth 2.0 Client |
OryClient::OAuth2Api | get_o_auth2_consent_request | GET /admin/oauth2/auth/requests/consent | Get OAuth 2.0 Consent Request |
OryClient::OAuth2Api | get_o_auth2_login_request | GET /admin/oauth2/auth/requests/login | Get OAuth 2.0 Login Request |
OryClient::OAuth2Api | get_o_auth2_logout_request | GET /admin/oauth2/auth/requests/logout | Get OAuth 2.0 Session Logout Request |
OryClient::OAuth2Api | get_trusted_o_auth2_jwt_grant_issuer | GET /admin/trust/grants/jwt-bearer/issuers/{id} | Get Trusted OAuth2 JWT Bearer Grant Type Issuer |
OryClient::OAuth2Api | introspect_o_auth2_token | POST /admin/oauth2/introspect | Introspect OAuth2 Access and Refresh Tokens |
OryClient::OAuth2Api | list_o_auth2_clients | GET /admin/clients | List OAuth 2.0 Clients |
OryClient::OAuth2Api | list_o_auth2_consent_sessions | GET /admin/oauth2/auth/sessions/consent | List OAuth 2.0 Consent Sessions of a Subject |
OryClient::OAuth2Api | list_trusted_o_auth2_jwt_grant_issuers | GET /admin/trust/grants/jwt-bearer/issuers | List Trusted OAuth2 JWT Bearer Grant Type Issuers |
OryClient::OAuth2Api | o_auth2_authorize | GET /oauth2/auth | OAuth 2.0 Authorize Endpoint |
OryClient::OAuth2Api | oauth2_token_exchange | POST /oauth2/token | The OAuth 2.0 Token Endpoint |
OryClient::OAuth2Api | patch_o_auth2_client | PATCH /admin/clients/{id} | Patch OAuth 2.0 Client |
OryClient::OAuth2Api | reject_o_auth2_consent_request | PUT /admin/oauth2/auth/requests/consent/reject | Reject OAuth 2.0 Consent Request |
OryClient::OAuth2Api | reject_o_auth2_login_request | PUT /admin/oauth2/auth/requests/login/reject | Reject OAuth 2.0 Login Request |
OryClient::OAuth2Api | reject_o_auth2_logout_request | PUT /admin/oauth2/auth/requests/logout/reject | Reject OAuth 2.0 Session Logout Request |
OryClient::OAuth2Api | revoke_o_auth2_consent_sessions | DELETE /admin/oauth2/auth/sessions/consent | Revoke OAuth 2.0 Consent Sessions of a Subject |
OryClient::OAuth2Api | revoke_o_auth2_login_sessions | DELETE /admin/oauth2/auth/sessions/login | Revokes OAuth 2.0 Login Sessions by either a Subject or a SessionID |
OryClient::OAuth2Api | revoke_o_auth2_token | POST /oauth2/revoke | Revoke OAuth 2.0 Access or Refresh Token |
OryClient::OAuth2Api | set_o_auth2_client | PUT /admin/clients/{id} | Set OAuth 2.0 Client |
OryClient::OAuth2Api | set_o_auth2_client_lifespans | PUT /admin/clients/{id}/lifespans | Set OAuth2 Client Token Lifespans |
OryClient::OAuth2Api | trust_o_auth2_jwt_grant_issuer | POST /admin/trust/grants/jwt-bearer/issuers | Trust OAuth2 JWT Bearer Grant Type Issuer |
OryClient::OidcApi | create_oidc_dynamic_client | POST /oauth2/register | Register OAuth2 Client using OpenID Dynamic Client Registration |
OryClient::OidcApi | create_verifiable_credential | POST /credentials | Issues a Verifiable Credential |
OryClient::OidcApi | delete_oidc_dynamic_client | DELETE /oauth2/register/{id} | Delete OAuth 2.0 Client using the OpenID Dynamic Client Registration Management Protocol |
OryClient::OidcApi | discover_oidc_configuration | GET /.well-known/openid-configuration | OpenID Connect Discovery |
OryClient::OidcApi | get_oidc_dynamic_client | GET /oauth2/register/{id} | Get OAuth2 Client using OpenID Dynamic Client Registration |
OryClient::OidcApi | get_oidc_user_info | GET /userinfo | OpenID Connect Userinfo |
OryClient::OidcApi | revoke_oidc_session | GET /oauth2/sessions/logout | OpenID Connect Front- and Back-channel Enabled Logout |
OryClient::OidcApi | set_oidc_dynamic_client | PUT /oauth2/register/{id} | Set OAuth2 Client using OpenID Dynamic Client Registration |
OryClient::PermissionApi | batch_check_permission | POST /relation-tuples/batch/check | Batch check permissions |
OryClient::PermissionApi | check_permission | GET /relation-tuples/check/openapi | Check a permission |
OryClient::PermissionApi | check_permission_or_error | GET /relation-tuples/check | Check a permission |
OryClient::PermissionApi | expand_permissions | GET /relation-tuples/expand | Expand a Relationship into permissions. |
OryClient::PermissionApi | post_check_permission | POST /relation-tuples/check/openapi | Check a permission |
OryClient::PermissionApi | post_check_permission_or_error | POST /relation-tuples/check | Check a permission |
OryClient::ProjectApi | create_organization | POST /projects/{project_id}/organizations | Create an Enterprise SSO Organization |
OryClient::ProjectApi | create_project | POST /projects | Create a Project |
OryClient::ProjectApi | create_project_api_key | POST /projects/{project}/tokens | Create project API key |
OryClient::ProjectApi | delete_organization | DELETE /projects/{project_id}/organizations/{organization_id} | Delete Enterprise SSO Organization |
OryClient::ProjectApi | delete_project_api_key | DELETE /projects/{project}/tokens/{token_id} | Delete project API key |
OryClient::ProjectApi | get_organization | GET /projects/{project_id}/organizations/{organization_id} | Get Enterprise SSO Organization by ID |
OryClient::ProjectApi | get_project | GET /projects/{project_id} | Get a Project |
OryClient::ProjectApi | get_project_members | GET /projects/{project}/members | Get all members associated with this project |
OryClient::ProjectApi | list_organizations | GET /projects/{project_id}/organizations | List all Enterprise SSO organizations |
OryClient::ProjectApi | list_project_api_keys | GET /projects/{project}/tokens | List a project's API keys |
OryClient::ProjectApi | list_projects | GET /projects | List All Projects |
OryClient::ProjectApi | patch_project | PATCH /projects/{project_id} | Patch an Ory Network Project Configuration |
OryClient::ProjectApi | patch_project_with_revision | PATCH /projects/{project_id}/revision/{revision_id} | Patch an Ory Network Project Configuration based on a revision ID |
OryClient::ProjectApi | purge_project | DELETE /projects/{project_id} | Irrecoverably purge a project |
OryClient::ProjectApi | remove_project_member | DELETE /projects/{project}/members/{member} | Remove a member associated with this project |
OryClient::ProjectApi | set_project | PUT /projects/{project_id} | Update an Ory Network Project Configuration |
OryClient::ProjectApi | update_organization | PUT /projects/{project_id}/organizations/{organization_id} | Update an Enterprise SSO Organization |
OryClient::RelationshipApi | check_opl_syntax | POST /opl/syntax/check | Check the syntax of an OPL file |
OryClient::RelationshipApi | create_relationship | PUT /admin/relation-tuples | Create a Relationship |
OryClient::RelationshipApi | delete_relationships | DELETE /admin/relation-tuples | Delete Relationships |
OryClient::RelationshipApi | get_relationships | GET /relation-tuples | Query relationships |
OryClient::RelationshipApi | list_relationship_namespaces | GET /namespaces | Query namespaces |
OryClient::RelationshipApi | patch_relationships | PATCH /admin/relation-tuples | Patch Multiple Relationships |
OryClient::WellknownApi | discover_json_web_keys | GET /.well-known/jwks.json | Discover Well-Known JSON Web Keys |
OryClient::WorkspaceApi | create_workspace | POST /workspaces | Create a new workspace |
OryClient::WorkspaceApi | create_workspace_api_key | POST /workspaces/{workspace}/tokens | Create workspace API key |
OryClient::WorkspaceApi | delete_workspace_api_key | DELETE /workspaces/{workspace}/tokens/{token_id} | Delete workspace API key |
OryClient::WorkspaceApi | get_workspace | GET /workspaces/{workspace} | Get a workspace |
OryClient::WorkspaceApi | list_workspace_api_keys | GET /workspaces/{workspace}/tokens | List a workspace's API keys |
OryClient::WorkspaceApi | list_workspace_projects | GET /workspaces/{workspace}/projects | List all projects of a workspace |
OryClient::WorkspaceApi | list_workspaces | GET /workspaces | List workspaces the user is a member of |
OryClient::WorkspaceApi | update_workspace | PUT /workspaces/{workspace} | Update an workspace |
Authentication schemes defined for the API:
FAQs
Unknown package
We found that ory-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.