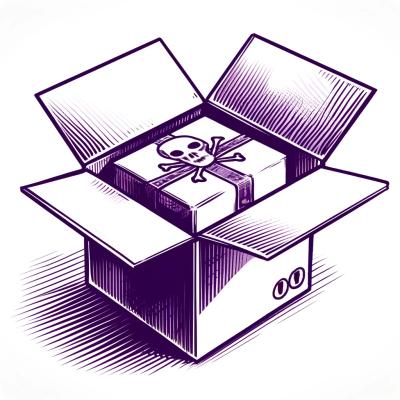
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Welcome to BinarySearch, a gem that brings the power of Red-Black Trees to your Ruby projects! 🚀
BinarySearch is a Ruby gem that implements a self-balancing binary search tree using the Red-Black Tree algorithm. It provides a list-like interface with blazing-fast search, insertion, and deletion operations. 💨
Add this line to your application's Gemfile:
gem 'binary_search'
And then execute:
bundle install
Here's a quick example of how to use BinarySearch:
require 'binary_search'
# Create a new list
list = BinarySearch::List.new([3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5])
# Get the sorted array
puts list.to_a # Output: [1, 1, 2, 3, 3, 4, 5, 5, 5, 6, 9]
# Check if a value exists
puts list.include?(4) # Output: true
# Remove all instances of a value
list.delete(1)
puts list.to_a # Output: [2, 3, 3, 4, 5, 5, 5, 6, 9]
# Add a new value
list.insert(7)
puts list.to_a # Output: [2, 3, 3, 4, 5, 5, 5, 6, 7, 9]
# Get the minimum and maximum values
puts list.min # Output: 2
puts list.max # Output: 9
Custom objects
require 'binary_search'
class Person
attr_accessor :name, :age
def initialize(name, age)
@name = name
@age = age
end
def <=>(other)
@age <=> other.age
end
end
list = BinarySearch::List.new([
Person.new('Alice', 25),
Person.new('Bob', 30),
Person.new('Charlie', 20),
Person.new('David', 35)
])
puts list.to_a.map(&:name) # Output: ["Charlie", "Alice", "Bob", "David"]
After checking out the repo, run bin/setup
to install dependencies. Then, run rake spec
to run the tests. You can also run bin/console
for an interactive prompt that will allow you to experiment.
To install this gem onto your local machine, run bundle exec rake install
.
Bug reports and pull requests are welcome on GitHub at https://github.com/sebyx07/binary_search. This project is intended to be a safe, welcoming space for collaboration, and contributors are expected to adhere to the code of conduct.
The gem is available as open source under the terms of the MIT License.
Everyone interacting in the BinarySearch project's codebases, issue trackers, chat rooms and mailing lists is expected to follow the code of conduct.
FAQs
Unknown package
We found that ruby_binary_search demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.