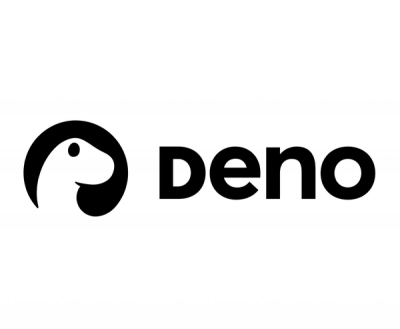
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
This is the official Storyblok ruby client for easy access of the management and content delivery api.
gem 'storyblok'
By default the client loads the "draft" version of the Story. Be sure to set the version to "published" to get the published content only.
# The draft mode is required for the preview
Storyblok::Client.new(version: 'draft')
# Requests only published stories
Storyblok::Client.new(version: 'published')
You should use the space access token AND api_region: 'us'
whenever your space was created under US
Server Location.
client = Storyblok::Client.new(token: 'YOUR_TOKEN', api_region: 'us')
# Without cache
client = Storyblok::Client.new(token: 'YOUR_TOKEN')
# Optionally set a cache client
redis = Redis.new(url: 'redis://localhost:6379')
cache = Storyblok::Cache::Redis.new(redis: redis)
client = Storyblok::Client.new(cache: cache, token: 'YOUR_TOKEN')
# Get a story
client.story('home')
# Get all Stories that start with news
client.stories({
:starts_with => 'news'
})
# Get all label datasource entries
client.datasource_entries({
:datasource => 'labels'
})
# Get all Tags that within the folder news
client.tags({
:starts_with => 'news'
})
client.links
tree = client.tree
puts '<ul>'
tree.each do |key, item|
puts '<li>' + item['item']['name']
if !item['children'].empty?
puts '<ul>'
item['children'].each do |key, inner_item|
puts '<li>' + inner_item['item']['name'] + '</li>'
end
puts '</ul>'
end
puts '</li>'
end
puts '</ul>'
client.space
Following an example of how to flush the client cache:
cache = Storyblok::Cache::Redis.new(redis: Redis.current)
client = Storyblok::Client.new(cache: cache, token: 'YOUR_TOKEN')
# Get a story and cache it
client.story('home')
# Flush the cache
client.flush
client = Storyblok::Client.new(oauth_token: 'YOUR_OAUTH_TOKEN')
# Get your spaces
client.get('spaces/')
client.post("spaces/{space_id}/stories", {story: {name: 'new', slug: "new"}})
client.put("spaces/{space_id}/stories/{story_id}", {story: {name: 'new', slug: "new"}})
client.delete("spaces/{space_id}/stories/{story_id}")
This SDK comes with a rendering service for richtext fields of Storyblok to get html output.
client.render(data.richtext_field)
Storyblok's richtext field also let's you insert content blocks. To render these blocks you can define a Lambda.
# Option 1: Define the resolver when initializing
client = Storyblok::Client.new(
component_resolver: ->(component, data) {
case component
when 'button'
"<button>#{data['text']}</button>"
when 'your_custom_component'
"<div class='welcome'>#{data['welcome_text']}</div>"
end
}
)
# Option 2: Define the resolver afterwards
client.set_component_resolver(->(component, data) {
"#{component}"
})
How to build a gem file.
gem build storyblok.gemspec
gem push storyblok-2.0.X.gem
We use RSpec for testing.
REDIS_URL
exported, the test suite will remove the keys with this pattern storyblok:*
from the redis database defined by REDIS_URL
export REDIS_URL="redis://localhost:6379"
Optionally you can generate the test report coverage by setting the environment variable
export COVERAGE=true
To run the whole test suite use the following command:
rspec
To run tests without redis cache tests (for when you don't have redis, or to avoid the test suite to remove the keys with this pattern storyblok:*
):
rspec --tag ~redis_cache:true
Bugs or Feature Requests? Submit an issue;
Do you have questions about Storyblok or you need help? Join our Discord Community.
Please see our contributing guidelines and our code of conduct. This project use semantic-release for generate new versions by using commit messages and we use the Angular Convention to naming the commits. Check this question about it in semantic-release FAQ.
FAQs
Unknown package
We found that storyblok demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.