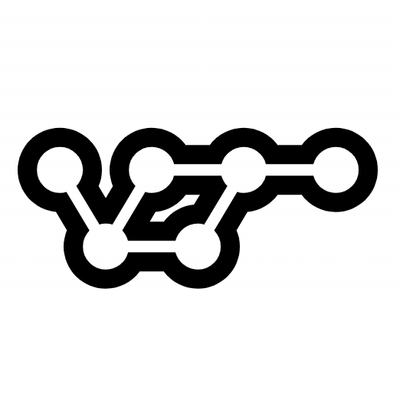
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Tree Structure allows the records of a ActiveRecord model to be organized in a tree structure.
Add this line to your application's Gemfile:
gem 'tree_structure'
And then execute:
$ bundle install
Or install it yourself as:
$ gem install tree_structure
After installing the tree_structure
gem in your Gemfile, follow these steps to set up the tree structure:
Add a parent_id
column to the table where you want to organize the tree structure. You can do this by creating a migration:
class AddParentIdToYourModel < ActiveRecord::Migration[6.0]
def change
add_column :your_model_name, :parent_id, :integer
add_index :your_model_name, :parent_id
end
end
Include the TreeStructure module in the model that will be organized in a tree structure. For example:
class YourModelName < ApplicationRecord
include TreeStructure
end
Run your migrations to update the database schema:
rails db:migrate
Once you have set up your model, you can start using the tree structure features. For example:
root1 = YourModelName.create(name: 'Root 1')
root2 = YourModelName.create(name: 'Root 2')
child1 = YourModelName.create(name: 'Child 1', parent: root1)
child2 = YourModelName.create(name: 'Child 2', parent: root1)
child3 = YourModelName.create(name: 'Child 3', parent: root2)
root1.root? #true
child1.root? #false
root1.leaf? #false
child1.leaf? #true
child1.ancestors
root1.descendants
root1.subtree
child1.siblings
child1.path_to_root
child2.depth
root1.leaves
child2.move_to_child_of root1
Bug reports and pull requests are welcome on GitHub at https://github.com/[USERNAME]/tree_structure.
The gem is available as open source under the terms of the MIT License.
FAQs
Unknown package
We found that tree_structure demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.