Redis client for Go

go-redis is brought to you by :star: uptrace/uptrace.
Uptrace is an open source and blazingly fast
distributed tracing tool powered
by OpenTelemetry and ClickHouse. Give it a star as well!
Upstash: Serverless Database for Redis
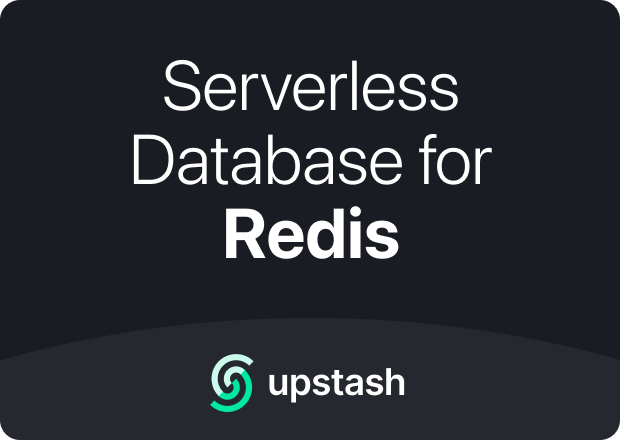
Upstash is a Serverless Database with Redis/REST API and durable storage. It is the perfect database
for your applications thanks to its per request pricing and low latency data.
Start for free in 30 seconds!
Resources
Ecosystem
This client also works with kvrocks, a distributed key
value NoSQL database that uses RocksDB as storage engine and is compatible with Redis protocol.
Features
Installation
go-redis supports 2 last Go versions and requires a Go version with
modules support. So make sure to initialize a Go
module:
go mod init github.com/my/repo
If you are using Redis 6, install go-redis/v8:
go get github.com/APITeamLimited/redis/v8
If you are using Redis 7, install go-redis/v9:
go get github.com/APITeamLimited/redis/v9
Quickstart
import (
"context"
"github.com/APITeamLimited/redis/v8"
"fmt"
)
var ctx = context.Background()
func ExampleClient() {
rdb := redis.NewClient(&redis.Options{
Addr: "localhost:6379",
Password: "",
DB: 0,
})
err := rdb.Set(ctx, "key", "value", 0).Err()
if err != nil {
panic(err)
}
val, err := rdb.Get(ctx, "key").Result()
if err != nil {
panic(err)
}
fmt.Println("key", val)
val2, err := rdb.Get(ctx, "key2").Result()
if err == redis.Nil {
fmt.Println("key2 does not exist")
} else if err != nil {
panic(err)
} else {
fmt.Println("key2", val2)
}
}
Look and feel
Some corner cases:
set, err := rdb.SetNX(ctx, "key", "value", 10*time.Second).Result()
set, err := rdb.SetNX(ctx, "key", "value", redis.KeepTTL).Result()
vals, err := rdb.Sort(ctx, "list", &redis.Sort{Offset: 0, Count: 2, Order: "ASC"}).Result()
vals, err := rdb.ZRangeByScoreWithScores(ctx, "zset", &redis.ZRangeBy{
Min: "-inf",
Max: "+inf",
Offset: 0,
Count: 2,
}).Result()
vals, err := rdb.ZInterStore(ctx, "out", &redis.ZStore{
Keys: []string{"zset1", "zset2"},
Weights: []int64{2, 3}
}).Result()
vals, err := rdb.Eval(ctx, "return {KEYS[1],ARGV[1]}", []string{"key"}, "hello").Result()
res, err := rdb.Do(ctx, "set", "key", "value").Result()
Run the test
go-redis will start a redis-server and run the test cases.
The paths of redis-server bin file and redis config file are defined in main_test.go
:
var (
redisServerBin, _ = filepath.Abs(filepath.Join("testdata", "redis", "src", "redis-server"))
redisServerConf, _ = filepath.Abs(filepath.Join("testdata", "redis", "redis.conf"))
)
For local testing, you can change the variables to refer to your local files, or create a soft link
to the corresponding folder for redis-server and copy the config file to testdata/redis/
:
ln -s /usr/bin/redis-server ./go-redis/testdata/redis/src
cp ./go-redis/testdata/redis.conf ./go-redis/testdata/redis/
Lastly, run:
go test
See also
Contributors
Thanks to all the people who already contributed!