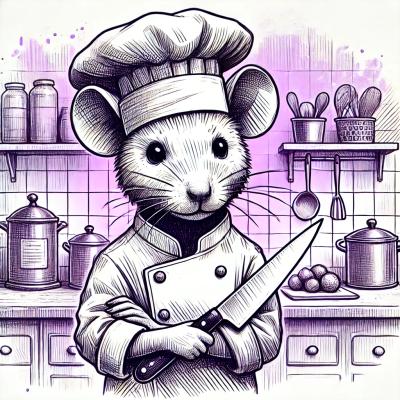
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@0xsequence/kit
Advanced tools
Sequence Kit 🧰 is a library enabling developers to easily integrate web3 wallets in their app. It is based on wagmi and supports all wagmi features.
View the demo! 👀
@0xsequence/kit
is the core package. Any extra modules require this package to be installed first.
To install this package:
npm install @0xsequence/kit wagmi ethers@6.13.0 viem 0xsequence @tanstack/react-query
# or
pnpm install @0xsequence/kit wagmi ethers@6.13.0 viem 0xsequence @tanstack/react-query
# or
yarn add @0xsequence/kit wagmi ethers@6.13.0 viem 0xsequence @tanstack/react-query
createConfig(walletType, options)
method is used to create your initial config and prepare sensible defaults that can be overriddenwalletType
is either 'waas' or 'universal'
interface CreateConfigOptions {
appName: string
projectAccessKey: string
chainIds?: number[]
defaultChainId?: number
disableAnalytics?: boolean
defaultTheme?: Theme
position?: ModalPosition
signIn?: {
logoUrl?: string
projectName?: string
useMock?: boolean
}
displayedAssets?: Array<{
contractAddress: string
chainId: number
}>
ethAuth?: EthAuthSettings
isDev?: boolean
wagmiConfig?: WagmiConfig // optional wagmiConfig overrides
waasConfigKey: string
enableConfirmationModal?: boolean
walletConnect?:
| boolean
| {
projectId: string
}
google?:
| boolean
| {
clientId: string
}
apple?:
| boolean
| {
clientId: string
rediretURI: string
}
email?:
| boolean
| {
legacyEmailAuth?: boolean
}
}
import { SequenceKit, createConfig } from '@0xsequence/kit'
import Content from './components/Content'
const config = createConfig('waas', {
projectAccessKey: '<your-project-access-key>',
chainIds: [1, 137]
defaultChainId: 1
appName: 'Demo Dapp',
waasConfigKey: '<your-waas-config-key>',
google: {
clientId: '<your-google-client-id>'
},
apple: {
clientId: '<your-apple-client-id>',
redirectUrl: '...'
},
walletConnect: {
projectId: '<your-wallet-connect-id>'
}
})
function App() {
return (
<SequenceKit config={config}>
<Content />
</SequenceKit>
)
}
React apps must be wrapped by a Wagmi client and the KitWalletProvider components. It is important that the Wagmi wrapper comes before the Sequence Kit wrapper.
import Content from './components/Content'
import { KitProvider, getDefaultConnectors, getDefaultChains } from '@0xsequence/kit'
import { QueryClient, QueryClientProvider } from '@tanstack/react-query'
import { createConfig, http, WagmiProvider } from 'wagmi'
import { mainnet, polygon, Chain } from 'wagmi/chains'
import '@0xsequence/kit/styles.css'
const projectAccessKey = 'xyz'
const chains = getDefaultChains(/* optional array of chain ids to filter */)
const transports = {}
chains.forEach(chain => {
transports[chain.id] = http()
})
const connectors = getDefaultConnectors('universal', {
projectAccessKey,
appName: 'demo app',
defaultChainId: 137,
walletConnect: {
projectId: '<your-wallet-connect-project-id>'
}
})
const config = createConfig({
chains,
transports,
connectors
})
const queryClient = new QueryClient()
function App() {
return (
<WagmiProvider config={config}>
<QueryClientProvider client={queryClient}>
<KitProvider>
<Content />
</KitProvider>
</QueryClientProvider>
</WagmiProvider>
)
}
Wallet selection is done through a modal which can be called programmatically.
import { useOpenConnectModal } from '@0xsequence/kit'
import { useDisconnect, useAccount } from 'wagmi'
const MyReactComponent = () => {
const { setOpenConnectModal } = useOpenConnectModal()
const { isConnected } = useAccount()
const onClick = () => {
setOpenConnectModal(true)
}
return <>{!isConnected && <button onClick={onClick}>Sign in</button>}</>
}
Use the useOpenConnectModal
to change to open or close the connection modal.
import { useOpenConnectModal } from '@0xsequence/kit'
// ...
const { setOpenConnectModal } = useOpenConnectModal()
setOpenConnectModal(true)
Use the useTheme
hook to get information about the current theme, such as light or dark, or set it to something else.
import { useTheme } from '@0xsequence/kit'
const { theme, setTheme } = useTheme()
setTheme('light')
The KitProvider
wrapper can accept an optional config object.
The settings are described in more detailed in the Sequence Kit documentation.
const kitConfig = {
defaultTheme: 'light',
position: 'top-left',
signIn: {
logoUrl: 'https://logo-dark-mode.svg',
projectName: 'my app',
},
// limits the digital assets displayed on the assets summary screen
displayedAssets: [
{
contractAddress: ethers.ZeroAddress,
chainId: 137,
},
{
contractAddress: '0x631998e91476da5b870d741192fc5cbc55f5a52e',
chainId: 137
}
],
}
<KitProvider config={kitConfig}>
<App />
<KitProvider>
Package | Description | Docs |
---|---|---|
@0xsequence/kit | Core package for Sequence Kit | Read more |
@0xsequence/kit-wallet | Embedded wallets for viewing and sending coins and collectibles | Read more |
@0xsequence/kit-checkout | Checkout modal with fiat onramp | Read more |
@0xsequence/kit-example-react | Example application showing sign in, wallet and checkout | Read more |
The React example can be used to test the library locally.
pnpm install
pnpm build
to build the packages.pnpm dev:react
or pnpm dev:next
to run the examples.Now that the core package is installed, you can install the embedded wallet or take a look at the checkout.
Apache-2.0
Copyright (c) 2017-present Horizon Blockchain Games Inc. / https://horizon.io
FAQs
Core package for Sequence Kit
The npm package @0xsequence/kit receives a total of 238 weekly downloads. As such, @0xsequence/kit popularity was classified as not popular.
We found that @0xsequence/kit demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.