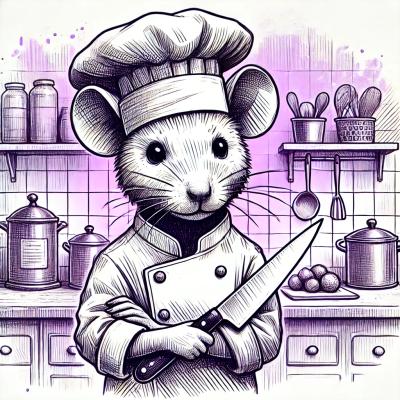
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@alttiri/base85
Advanced tools
Pretty fast base85 JavaScript library (with TS support).
It is designed to encode binary data (Uint8Array
) into a "base85" text string and vice versa.
npm install @alttiri/base85
function encode(ui8a: Uint8Array, charset?: "ascii85" | "z85" | string): string
encode
encodes the input Uint8Array
into base85 string
.
function decode(base85: string, charset?: "ascii85" | "z85" | string): Uint8Array
decode
decodes the input base85 string
into Uint8Array
.
charset
is "z85" by default.
Also, there are encodeBase85
and decodeBase85
aliases for encode
and decode
functions.
Binary data encoding example:
import {encodeBase85} from "@alttiri/base85";
// png image, 169 bytes
const imageBytes = new Uint8Array([137,80,78,71,13,10,26,10,0,0,0,13,73,72,68,82,0,0,0,32,0,0,0,32,8,2,0,0,0,252,24,237,163,0,0,0,1,115,82,71,66,0,174,206,28,233,0,0,0,4,103,65,77,65,0,0,177,143,11,252,97,5,0,0,0,9,112,72,89,115,0,0,14,195,0,0,14,195,1,199,111,168,100,0,0,0,62,73,68,65,84,72,75,237,210,49,10,0,48,8,197,80,239,127,105,187,252,161,208,150,32,93,243,86,149,44,86,191,213,33,131,9,3,200,0,50,128,12,160,92,74,63,242,77,55,217,216,100,48,97,0,25,64,6,144,1,208,189,0,183,189,228,126,66,93,37,1,0,0,0,0,73,69,78,68,174,66,96,130]);
const base85 = encodeBase85(imageBytes);
console.log(base85);
console.log(base85.length);
// "Ibl@q4gj0X0000dnK#lE0000w0000w2M:#a0q)Y3Qw$n]0DRdeliagl9o^C600D}2o*F:VV5Yp<vfDSh010Qns-TMy4-nnD4-ns/z(vgD002hOl{T^yoypdZ3ih:=-zD2Mx$Kqp^3t!W]h.bcr>)fdG9.U305x6kPJ>8N[>z6@/KMWA02X3aKo9.w0jPV5ENmr^0rr9107/QOm6n<:F="
// 212
If you need to encode a text (are you really need it?) use TextEncoder
/TextDecoder
.
const input = "Man is distinguished";
const inputBytes = utf8StringToArrayBuffer(input);
console.log(encode(inputBytes, "ascii85")); // "9jqo^BlbD-BleB1DJ+*+F(f,q"
console.log(encode(inputBytes, "z85")); // "o<}]Zx(+zcx(!xgzFa9aB7/b}"
const outputBytes = decode("9jqo^BlbD-BleB1DJ+*+F(f,q", "ascii85");
const output = arrayBufferToUtf8String(outputBytes);
console.log(output); // "Man is distinguished"
For more examples see the tests.
You can test the lib online in the browser's console: https://alttiri.github.io/base85/online
All required things are already in the global scope. (encode
, decode
; util functions: utf8StringToArrayBuffer
and others.)
Note.
The optimisation for "ascii85"
by replacing "\0\0\0\0"
by "z"
instead of "!!!!!"
and " "
(4 spaces) by "y"
instead of "+<VdL"
is not supported.
Anyway nobody forbids you to do something like base85.replaceAll("!!!!!", "z")
as well as to add "<~"
, "~>"
manually if you need it.
/** 85 unique characters string */
type CharSet = string;
/**
* Returns Base85 string.
* @param {Uint8Array} ui8a - input data to encode
* @param {"ascii85" | "z85" | string} [charset="z85"] - 85 unique characters string
* @return {string}
* */
export declare function encode(ui8a: Uint8Array, charset?: "ascii85" | "z85" | CharSet): string;
/**
* Decodes Base85 string.
* @param {string} base85 - base85 encoded string
* @param {"ascii85" | "z85" | string} [charset="z85"] - 85 unique characters string
* @return {Uint8Array}
* */
export declare function decode(base85: string, charset?: "ascii85" | "z85" | CharSet): Uint8Array;
/** Alias to `encode`. */
export declare const encodeBase85: typeof encode;
/** Alias to `decode`. */
export declare const decodeBase85: typeof decode;
declare const base85: {
encode: typeof encode;
decode: typeof decode;
encodeBase85: typeof encode;
decodeBase85: typeof decode;
};
export default base85;
npm install @alttiri/base85
npm install git+https://github.com/alttiri/base85.git
Based on SemVer:
npm install git+https://github.com/alttiri/base85.git#semver:1.5.1
Or add
"@alttiri/base85": "github:alttiri/base85#semver:1.5.1"
as dependencies
in package.json
file.
See available tags.
Based on a commit hash:
npm install git+https://git@github.com/alttiri/base85.git#ca3217f3d1075b8ff0966e24437326a6c2537bbc
Or add
"@alttiri/base85": "github:alttiri/base85#ca3217f3d1075b8ff0966e24437326a6c2537bbc"
as dependencies
in package.json
file.
See available commits hashes.
To install you need first to create .npmrc
file with @alttiri:registry=https://npm.pkg.github.com
content:
echo @alttiri:registry=https://npm.pkg.github.com >> .npmrc
only then run
npm install @alttiri/base85
Note, that GitHub Packages requires to have also ~/.npmrc
file (.npmrc
in your home dir) with //npm.pkg.github.com/:_authToken=TOKEN
content, where TOKEN
is a token with the read:packages
permission, take it here https://github.com/settings/tokens/new.
FAQs
Pretty fast base85 JavaScript library
The npm package @alttiri/base85 receives a total of 28 weekly downloads. As such, @alttiri/base85 popularity was classified as not popular.
We found that @alttiri/base85 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.