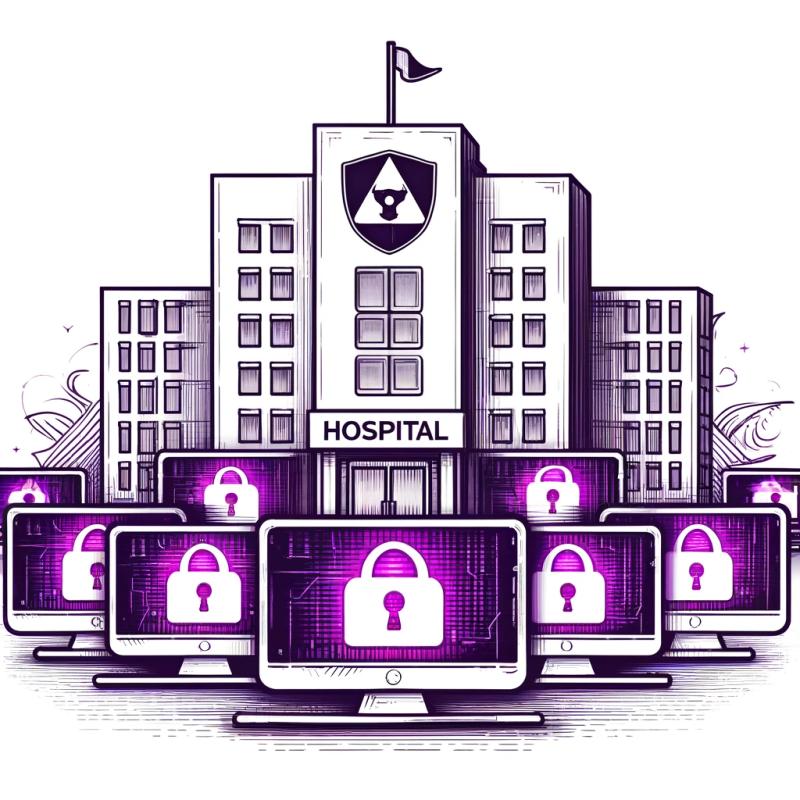
Security News
Mobile, Alabama Hospital Refuses to Pay Settlement in Landmark Ransomware Death Lawsuit
A hospital in Mobile, Alabama, agreed to a settlement in a landmark ransomware death lawsuit, but is now reportedly reconsidering the agreement and refusing to pay.