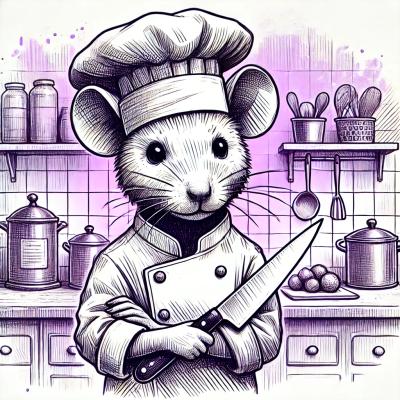
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@azure/core-lro
Advanced tools
The @azure/core-lro package provides a framework for building and working with long-running operations (LROs) in Azure services. It offers a standardized way to poll for the status of these operations and retrieve the final results, abstracting the complexity involved in handling LROs.
Creating and managing long-running operations
This feature allows developers to implement custom pollers for managing long-running operations. The code sample demonstrates how to extend the Poller class to create a custom LRO poller.
const { Poller } = require('@azure/core-lro');
class MyLroPoller extends Poller {
async cancelOperation() {
// Cancel the operation if supported
}
async delay() {
// Implement delay between polls
}
}
const myPoller = new MyLroPoller();
await myPoller.pollUntilDone();
Polling for operation status
This feature enables polling for the status of a long-running operation until it is completed. The code sample shows how to use the Poller class to poll an operation until it's done.
const { Poller } = require('@azure/core-lro');
async function pollOperation(operation) {
const poller = new Poller({
operation,
});
return await poller.pollUntilDone();
}
The 'async' package provides utilities for working with asynchronous JavaScript, including control flow, iteration, and utilities functions. While it doesn't specifically target long-running operations, it offers tools that could be used to manage asynchronous tasks and operations, which could indirectly support LRO scenarios. However, it lacks the direct LRO management and polling capabilities of @azure/core-lro.
RxJS is a library for reactive programming using Observables, to make it easier to compose asynchronous or callback-based code. This can be particularly useful for managing streams of events or asynchronous tasks, which could include long-running operations. However, RxJS does not provide specific abstractions for LROs like @azure/core-lro does, focusing instead on a broader set of reactive programming tools.
Azure's Core LRO is a JavaScript library that provides an API that aims to allow the azure-sdk-for-js public libraries to implement fully featured pollers to manage long running operations with the Azure services.
core-lro is made following our guidelines, here: https://azure.github.io/azure-sdk/typescript_design.html#ts-lro
Since this package is intended to be consumed by developers of the azure-sdk-for-js repository, you'll need to manually add the following entry to your package.json's dependencies:
{
// ...
"dependencies": {
// ...
"@azure/core-lro": "1.0.0-preview.1",
// ...
}
}
TypeScript users need to have Node type definitions installed:
npm install @types/node
You also need to enable compilerOptions.allowSyntheticDefaultImports
in your
tsconfig.json. Note that if you have enabled compilerOptions.esModuleInterop
,
allowSyntheticDefaultImports
is enabled by default. See TypeScript's
compiler options
handbook
for more information.
Now we'll examine how to set up an implementation of an operation and a poller.
You will be able to find some working examples of an implementation of an operation and a poller both in our test files: https://github.com/Azure/azure-sdk-for-js/tree/master/sdk/core/core-lro/test as well as in the azure-sdk-for-js libraries that might be currently using core-lro, which can be found here: https://github.com/Azure/azure-sdk-for-js/search?q="from+%40azure%5C%2Fcore-lro"&unscoped_q="from+%40azure%5C%2Fcore-lro"
First, you'll need to import the definitions of a poll operation and its state, as follows:
import { PollOperationState, PollOperation } from "@azure/core-lro";
A PollOperation requires implementing its state. We recommend extending the PollOperationState we provide. An example can be:
export interface MyOperationState extends PollOperationState<string> {
serviceClient: any; // You define all of these properties
myCustomString: string;
startedAt: Date;
finishedAt: Date;
// ... and so on
}
Now, to be able to create your custom operations, you'll need to extend the PollOperation class with both your operation's state and the final result value. For this example, we'll think on the final result value to be a string, but it can be any type.
export interface MyPollOperation extends PollOperation<MyOperationState, string> {}
Now you can make a utility function to create new instances of your operation, as follows:
function makeOperation(
state: MyOperationState,
): MyOperation {
return {
// We recommend you to create copies of the given state,
// just to make sure that no references are left or manipulated by mistake.
state: {
...state,
},
update,
cancel,
toString
};
}
Keep in mind that you'll need to have implemented these three functions: update
, cancel
and toString
on order to successfully create an operation. Ideas of how you might do so follow:
async function update(
this: MyOperation,
options: {
abortSignal?: AbortSignalLike;
fireProgress?: (state: MyOperationState) => void;
} = {}
): Promise<MyOperation> {
let isDone: boolean = false;
let doFireProgress: boolean = false;
// Asyncrhonously call your service client...
// You might also update the operation's state
if (isDone) {
this.state.completed = true;
this.state.result = "Done";
}
// You can also arbitrarily report progress
if (doFireProgress) {
options.fireProgress(state);
}
return makeOperation(this.state);
}
async function cancel(
this: MyOperation,
options: { abortSignal?: AbortSignal } = {}
): Promise<MyOperation> {
// Reach out to your service to trigger the cancellation of the operation
return makeOperation(
{
...this.state,
cancelled: true
}
);
}
function toString(this: MyOperation): string {
return JSON.stringify({
state: {
...this.state,
// Only the plain text properties, for examle
}
});
}
To implement a poller, you must pull in the definitions of your operation and extend core-lro's poller, as follows:
import { Poller } from "@azure/core-lro";
import { makeOperation, MyOperation, MyOperationState } from "./myOperation";
// See that "string" here is the type of the result
export class MyPoller extends Poller<MyOperationState, string> {
constructor(
baseOperation?: MyOperation,
onProgress?: (state: MyOperationState) => void
) {
let state: MyOperationState = {};
if (baseOperation) {
state = baseOperation.state;
}
const operation = makeOperation(state);
super(operation);
// Setting up the poller to call progress when the operation decides.
// This ties to the operation's update method, which receives a
// fireProgress method in the optional properties.
if (onProgress) {
this.onProgress(onProgress);
}
}
async delay(): Promise<void> {
// Your own implementation of a delay
}
}
Here's one simple examle of your poller in action. More examples can be found in the test folder near this README.
const poller = new MyPoller();
// Waiting until the operation completes
const result = await poller.pollUntilDone();
const state = poller.getOperationState();
console.log(state.completed);
TODO.
TODO.
This project welcomes contributions and suggestions. Please read the contributing guidelines for detailed information about how to contribute and what to expect while contributing.
To run our tests, first install the dependencies (with npm install
or rush install
),
then run the unit tests with: npm run unit-test
.
This project has adopted the Microsoft Open Source Code of Conduct. For more information see the Code of Conduct FAQ or contact opencode@microsoft.com with any additional questions or comments.
FAQs
Isomorphic client library for supporting long-running operations in node.js and browser.
The npm package @azure/core-lro receives a total of 1,550,753 weekly downloads. As such, @azure/core-lro popularity was classified as popular.
We found that @azure/core-lro demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.