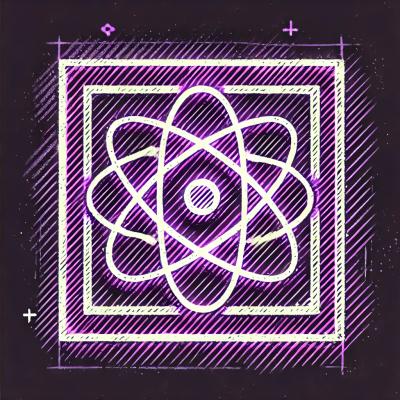
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
@chakra-ui/color-mode
Advanced tools
React component and hooks for handling light and dark mode.
@chakra-ui/color-mode is a package that provides utilities for managing color modes (e.g., light and dark themes) in Chakra UI applications. It allows developers to easily toggle between different color modes and persist the user's preference.
Color Mode Provider
The Color Mode Provider is used to wrap your application and provide the color mode context to all components within the app.
import { ChakraProvider, ColorModeProvider } from '@chakra-ui/react';
function App() {
return (
<ChakraProvider>
<ColorModeProvider>
<YourComponent />
</ColorModeProvider>
</ChakraProvider>
);
}
useColorMode Hook
The useColorMode hook allows you to access the current color mode and a function to toggle between light and dark modes.
import { useColorMode } from '@chakra-ui/react';
function ToggleButton() {
const { colorMode, toggleColorMode } = useColorMode();
return (
<button onClick={toggleColorMode}>
Toggle {colorMode === 'light' ? 'Dark' : 'Light'}
</button>
);
}
Color Mode Script
The Color Mode Script is used to ensure that the initial color mode is correctly set on the server-side and during hydration.
import { ColorModeScript } from '@chakra-ui/react';
function App() {
return (
<html>
<head>
<ColorModeScript />
</head>
<body>
<YourComponent />
</body>
</html>
);
}
styled-components is a library for React and React Native that allows you to use component-level styles in your application. It supports theming, including light and dark modes, but requires more manual setup compared to @chakra-ui/color-mode.
Emotion is a library designed for writing CSS styles with JavaScript. It provides powerful and flexible theming capabilities, including support for color modes. However, it is more general-purpose and not as tightly integrated with a UI component library as @chakra-ui/color-mode.
theme-ui is a library for building themeable user interfaces based on constraint-based design principles. It includes built-in support for color modes and theming, similar to @chakra-ui/color-mode, but is part of a different ecosystem.
React component that adds support for light mode and dark mode using
localStorage
and matchMedia
.
yarn add @chakra-ui/color-mode
# or
npm i @chakra-ui/color-mode
To enable this behavior within your apps, wrap your application in a
ColorModeProvider
below the ThemeProvider
import * as React from "react"
import { ColorModeProvider } from "@chakra-ui/color-mode"
import theme from "./theme"
function App({ children }) {
return (
<ThemeProvider theme={theme}>
<ColorModeProvider>{children}</ColorModeProvider>
</ThemeProvider>
)
}
Then you can use the hook useColorMode
within your application.
function Example() {
const { colorMode, toggleColorMode } = useColorMode()
return (
<header>
<Button onClick={toggleColorMode}>
Toggle {colorMode === "light" ? "Dark" : "Light"}
</Button>
</header>
)
}
FAQs
React component and hooks for handling light and dark mode.
The npm package @chakra-ui/color-mode receives a total of 329,492 weekly downloads. As such, @chakra-ui/color-mode popularity was classified as popular.
We found that @chakra-ui/color-mode demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.