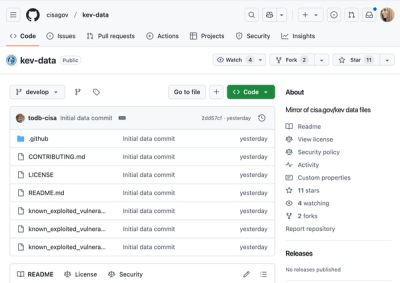
Security News
CISA Brings KEV Data to GitHub
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.
@chakra-ui/system
Advanced tools
@chakra-ui/system is a foundational package for building custom components in Chakra UI. It provides a set of utilities and hooks to create theme-aware, responsive, and accessible components with ease.
Theming
The `useTheme` hook allows you to access the theme object, enabling you to create components that are consistent with your application's design system.
import { useTheme } from '@chakra-ui/system';
function ThemedComponent() {
const theme = useTheme();
return <div style={{ color: theme.colors.primary }}>Hello, Theme!</div>;
}
Responsive Styles
The `Box` component and responsive style props allow you to create components that adapt to different screen sizes easily.
import { Box } from '@chakra-ui/system';
function ResponsiveComponent() {
return (
<Box
width={{ base: '100%', md: '50%' }}
padding={{ base: '4', md: '8' }}
>
Responsive Box
</Box>
);
}
Style Props
Style props enable you to apply CSS styles directly to components using a prop-based syntax, making it easier to style components without writing separate CSS files.
import { Box } from '@chakra-ui/system';
function StyledComponent() {
return <Box bg='tomato' w='100%' p={4} color='white'>Styled Box</Box>;
}
Custom Components
The `chakra` function allows you to create custom components with base styles and variants, making it easy to build reusable and consistent UI elements.
import { chakra } from '@chakra-ui/system';
const CustomButton = chakra('button', {
baseStyle: {
bg: 'blue.500',
color: 'white',
padding: '8px 16px',
borderRadius: '4px',
},
});
function App() {
return <CustomButton>Click Me</CustomButton>;
}
Styled-components is a popular library for writing CSS-in-JS. It allows you to create styled React components with tagged template literals. Compared to @chakra-ui/system, styled-components offers more flexibility in defining styles but lacks built-in theming and responsive design utilities.
Emotion is another CSS-in-JS library that provides powerful and flexible styling capabilities. It offers both a styled API and a css function for writing styles. Emotion is similar to @chakra-ui/system in terms of flexibility but does not come with the same level of built-in theming and responsive design support.
Theme UI is a library for building themeable user interfaces based on constraint-based design principles. It provides a similar theming and styling system to @chakra-ui/system but is more focused on design constraints and scales. Theme UI is a good alternative if you prefer a more design-system-oriented approach.
Styled API for creating atomic, theme-aware component styling.
yarn add @chakra-ui/system
# or
npm i @chakra-ui/system
In modern web development, we have lots of solutions and architectures that have tried to unify how components are styled. We've seen CSS architectures like BEM, SMACSS, etc, and frameworks like theme-ui, and Tailwind CSS.
While these solutions work great, we still think there is a sheer amount of work required to create a fully customizable, theme-aware component.
Chakra provides enhanced JSX elements that can be styled directly via props, or
can accept the common sx
prop for custom styles.
We'll provide a chakra function, just like styled-components. Users can create
any component using the chakra.[element]
. The resulting component will be a
styled component and have all system props.
<chakra.button bg="green.200" _hover={{ bg: "green.300" }}>
Click me
</chakra.button>
<chakra.h1 fontSize="lg"> Heading </chakra.h1>
// create your own box
const Box = chakra.div
// you can still use the `as` prop
<Box as="h1">This is my box</Box>
// for custom components
const ChakraPowered = chakra(YourComponent)
// TS: chakra will infer the types of Link and
// make it available in props
<chakra.a as={Link} to="/home"> Click me</chakra.a>
A way to define themeable components in chakra. We believe most re-usable, atomic components have the following modifiers:
Our goal with this component API is to design a common interface to style any component given these characteristics. Here's how it works:
// 1. create a component schema in your theme
const theme = {
colors: {
green: {
light: "#dfdfdf",
normal: "#dfdfdf",
dark: "#d566Df",
darker: "#dfd56f"
},
blue: {}
},
components: {
Button: {
defaultProps: {
variant: "solid",
size: "md",
colorScheme: "blue"
},
variants: {
// props has colorScheme, colorMode (light mode or dark mode)
solid: props => ({
bg: `${props.colorScheme}.normal`,
color: "white",
}),
outline: {
border: "2px",
borderColor: "green.normal"
}
},
sizes: {
sm: {
padding: 20,
fontSize: 12
},
md: {
padding: 40,
fontSize: 15
}
}
}
}
};
// 2. create or import Button from chakra-ui
import { Button } from "@chakra-ui/react"
// or your own button
const Button = chakra("button", { themeKey: "Button" })
// 3. use the button. It'll have the visual props defined in defaultProps
<Button>Click me</Button>
// 4. override the defaultProps
<Button variant="outline" colorScheme="green">Click me</Button>
FAQs
Chakra UI system primitives
The npm package @chakra-ui/system receives a total of 389,669 weekly downloads. As such, @chakra-ui/system popularity was classified as popular.
We found that @chakra-ui/system demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.