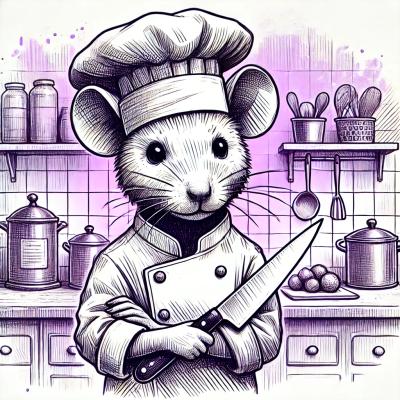
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@codewarriorr/hw-app-btcv
Advanced tools
Ledger Hardware Wallet BTC JavaScript bindings. Also supports many altcoins.
Bitcoin API.
transport
Transport<any>scrambleKey
string (optional, default "BTC"
)import Btc from "@ledgerhq/hw-app-btc";
const btc = new Btc(transport)
path
string a BIP 32 path
opts
(boolean | {verify: boolean?, format: AddressFormat?})?
options
an object with optional these fields:- verify (boolean) will ask user to confirm the address on the device
format ("legacy" | "p2sh" | "bech32" | "cashaddr") to use different bitcoin address formatter.NB The normal usage is to use:- legacy format with 44' paths
p2sh format with 49' paths
bech32 format with 173' paths
cashaddr in case of Bitcoin Cash
btc.getWalletPublicKey("44'/0'/0'/0/0").then(o => o.bitcoinAddress)
btc.getWalletPublicKey("49'/0'/0'/0/0", { format: "p2sh" }).then(o => o.bitcoinAddress)
Returns Promise<{publicKey: string, bitcoinAddress: string, chainCode: string}>
You can sign a message according to the Bitcoin Signature format and retrieve v, r, s given the message and the BIP 32 path of the account to sign.
btc.signMessageNew_async("44'/60'/0'/0'/0", Buffer.from("test").toString("hex")).then(function(result) {
var v = result['v'] + 27 + 4;
var signature = Buffer.from(v.toString(16) + result['r'] + result['s'], 'hex').toString('base64');
console.log("Signature : " + signature);
}).catch(function(ex) {console.log(ex);});
Returns Promise<{v: number, r: string, s: string}>
To sign a transaction involving standard (P2PKH) inputs, call createTransaction with the following parameters
arg
CreateTransactionArginputs
is an array of [ transaction, output_index, optional redeem script, optional sequence ] where- transaction is the previously computed transaction object for this UTXO
associatedKeysets
is an array of BIP 32 paths pointing to the path to the private key used for each UTXOchangePath
is an optional BIP 32 path pointing to the path to the public key used to compute the change addressoutputScriptHex
is the hexadecimal serialized outputs of the transaction to signlockTime
is the optional lockTime of the transaction to sign, or default (0)sigHashType
is the hash type of the transaction to sign, or default (all)segwit
is an optional boolean indicating wether to use segwit or notinitialTimestamp
is an optional timestamp of the function call to use for coins that necessitate timestamps only, (not the one that the tx will include)additionals
list of additionnal options- "bech32" for spending native segwit outputs
expiryHeight
is an optional Buffer for zec overwinter / sapling TxsuseTrustedInputForSegwit
trust inputs for segwit transactionsbtc.createTransaction({
inputs: [ [tx1, 1] ],
associatedKeysets: ["0'/0/0"],
outputScriptHex: "01905f0100000000001976a91472a5d75c8d2d0565b656a5232703b167d50d5a2b88ac"
}).then(res => ...);
Returns any the signed transaction ready to be broadcast
To obtain the signature of multisignature (P2SH) inputs, call signP2SHTransaction_async with the folowing parameters
arg
SignP2SHTransactionArginputs
is an array of [ transaction, output_index, redeem script, optional sequence ] where- transaction is the previously computed transaction object for this UTXO
associatedKeysets
is an array of BIP 32 paths pointing to the path to the private key used for each UTXOoutputScriptHex
is the hexadecimal serialized outputs of the transaction to signlockTime
is the optional lockTime of the transaction to sign, or default (0)sigHashType
is the hash type of the transaction to sign, or default (all)btc.signP2SHTransaction({
inputs: [ [tx, 1, "52210289b4a3ad52a919abd2bdd6920d8a6879b1e788c38aa76f0440a6f32a9f1996d02103a3393b1439d1693b063482c04bd40142db97bdf139eedd1b51ffb7070a37eac321030b9a409a1e476b0d5d17b804fcdb81cf30f9b99c6f3ae1178206e08bc500639853ae"] ],
associatedKeysets: ["0'/0/0"],
outputScriptHex: "01905f0100000000001976a91472a5d75c8d2d0565b656a5232703b167d50d5a2b88ac"
}).then(result => ...);
Returns any the signed transaction ready to be broadcast
For each UTXO included in your transaction, create a transaction object from the raw serialized version of the transaction used in this UTXO.
transactionHex
stringisSegwitSupported
boolean? (optional, default false
)hasTimestamp
boolean (optional, default false
)hasExtraData
boolean (optional, default false
)additionals
Array<string> (optional, default []
)const tx1 = btc.splitTransaction("01000000014ea60aeac5252c14291d428915bd7ccd1bfc4af009f4d4dc57ae597ed0420b71010000008a47304402201f36a12c240dbf9e566bc04321050b1984cd6eaf6caee8f02bb0bfec08e3354b022012ee2aeadcbbfd1e92959f57c15c1c6debb757b798451b104665aa3010569b49014104090b15bde569386734abf2a2b99f9ca6a50656627e77de663ca7325702769986cf26cc9dd7fdea0af432c8e2becc867c932e1b9dd742f2a108997c2252e2bdebffffffff0281b72e00000000001976a91472a5d75c8d2d0565b656a5232703b167d50d5a2b88aca0860100000000001976a9144533f5fb9b4817f713c48f0bfe96b9f50c476c9b88ac00000000");
Returns Transaction
const tx1 = btc.splitTransaction("01000000014ea60aeac5252c14291d428915bd7ccd1bfc4af009f4d4dc57ae597ed0420b71010000008a47304402201f36a12c240dbf9e566bc04321050b1984cd6eaf6caee8f02bb0bfec08e3354b022012ee2aeadcbbfd1e92959f57c15c1c6debb757b798451b104665aa3010569b49014104090b15bde569386734abf2a2b99f9ca6a50656627e77de663ca7325702769986cf26cc9dd7fdea0af432c8e2becc867c932e1b9dd742f2a108997c2252e2bdebffffffff0281b72e00000000001976a91472a5d75c8d2d0565b656a5232703b167d50d5a2b88aca0860100000000001976a9144533f5fb9b4817f713c48f0bfe96b9f50c476c9b88ac00000000");
const outputScript = btc.serializeTransactionOutputs(tx1).toString('hex');
Returns Buffer
inputs
Array<[Transaction, number, string?, number?]>associatedKeysets
Array<string>changePath
string?outputScriptHex
stringlockTime
number?sigHashType
number?segwit
boolean?initialTimestamp
number?additionals
Array<string>expiryHeight
Buffer?useTrustedInputForSegwit
boolean?onDeviceStreaming
function ({progress: number, total: number, index: number}): void?onDeviceSignatureRequested
function (): void?onDeviceSignatureGranted
function (): void?address format is one of legacy | p2sh | bech32 | cashaddr
Type: ("legacy"
| "p2sh"
| "bech32"
| "cashaddr"
)
$0
Transaction
$0.outputs
const tx1 = btc.splitTransaction("01000000014ea60aeac5252c14291d428915bd7ccd1bfc4af009f4d4dc57ae597ed0420b71010000008a47304402201f36a12c240dbf9e566bc04321050b1984cd6eaf6caee8f02bb0bfec08e3354b022012ee2aeadcbbfd1e92959f57c15c1c6debb757b798451b104665aa3010569b49014104090b15bde569386734abf2a2b99f9ca6a50656627e77de663ca7325702769986cf26cc9dd7fdea0af432c8e2becc867c932e1b9dd742f2a108997c2252e2bdebffffffff0281b72e00000000001976a91472a5d75c8d2d0565b656a5232703b167d50d5a2b88aca0860100000000001976a9144533f5fb9b4817f713c48f0bfe96b9f50c476c9b88ac00000000");
const outputScript = btc.serializeTransactionOutputs(tx1).toString('hex');
Returns Buffer
inputs
Array<[Transaction, number, string?, number?]>associatedKeysets
Array<string>outputScriptHex
stringlockTime
number?sigHashType
number?segwit
boolean?transactionVersion
number?FAQs
Ledger Hardware Wallet Bitcoin Vault Application API
The npm package @codewarriorr/hw-app-btcv receives a total of 7 weekly downloads. As such, @codewarriorr/hw-app-btcv popularity was classified as not popular.
We found that @codewarriorr/hw-app-btcv demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.