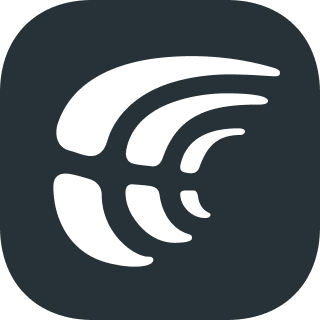
Crowdin JavaScript client
The Crowdin JavaScript client is a lightweight interface to the Crowdin API v2 that works in any JavaScript environment, including web browsers, workers in web browsers, extensions in web browsers or desktop applications, Node.js etc. It provides common services for making API requests.
Our API is a full-featured RESTful API that helps you to integrate localization into your development process. The endpoints that we use allow you to easily make calls to retrieve information and to execute actions needed.
For more about Crowdin API v2 see the documentation.


Build Status
Azure CI (Linux) | Azure CI (Windows) | Azure CI (MacOS) |
---|
?branchName=master) | ?branchName=master) | ?branchName=master) |
 |  |  |
Table of Contents
Installation
npm
npm i @crowdin/crowdin-api-client
yarn
yarn add @crowdin/crowdin-api-client
Quick Start
Typescript
import crowdin, { Credentials } from '@crowdin/crowdin-api-client';
const credentials: Credentials = {
token: 'personalAccessToken',
organization: 'organizationName'
};
const { projectsGroupsApi } = new crowdin(credentials);
projectsGroupsApi.listProjects()
.then(projects => console.log(projects))
.catch(error => console.error(error));
async function getProjects() {
try {
const projects = await projectsGroupsApi.listProjects();
console.log(projects);
} catch (error) {
console.error(error);
}
}
Or specific API instances:
import { Credentials, ProjectsGroups } from '@crowdin/crowdin-api-client';
const credentials: Credentials = {
token: 'personalAccessToken',
organization: 'organizationName'
};
const projectsGroupsApi = new ProjectsGroups(credentials);
projectsGroupsApi.listProjects()
.then(projects => console.log(projects))
.catch(error => console.error(error));
Javascript
import crowdin from '@crowdin/crowdin-api-client';
const { projectsGroupsApi } = new crowdin({
token: 'personalAccessToken',
organization: 'organizationName'
});
projectsGroupsApi.listProjects()
.then(projects => console.log(projects))
.catch(error => console.error(error));
async function getProjects() {
try {
const projects = await projectsGroupsApi.listProjects();
console.log(projects);
} catch (error) {
console.error(error);
}
}
Or specific API instances:
import { ProjectsGroups } from '@crowdin/crowdin-api-client';
const projectsGroupsApi = new ProjectsGroups({
token: 'personalAccessToken',
organization: 'organizationName'
});
projectsGroupsApi.listProjects()
.then(projects => console.log(projects))
.catch(error => console.error(error));
You can generate Personal Access Token in your Crowdin Account Settings.
List of projects with Fetch API
In addition if you use client in non-Node.js environment you might have a troubles with http calls.
This client uses axios which internally uses http
and https
Node modules.
So there is an option to use http client based on Fetch API.
import { ProjectsGroups, HttpClientType } from '@crowdin/crowdin-api-client';
const projectsGroupsApi = new ProjectsGroups(credentials, {
httpClientType: HttpClientType.FETCH
});
Or even pass your own http client as httpClient
property which should implement HttpClient
interface.
Retry configuration
There is a possibility to configure client invoke http calls with retry mechanism.
import { ProjectsGroups, HttpClientType } from '@crowdin/crowdin-api-client';
const projectsGroupsApi = new ProjectsGroups(credentials, {
retryConfig: {
retries: 2,
waitInterval: 100,
conditions: [
{
test(error) {
return error.code === 40
}
}
]
}
});
Contribution
We are happy to accept contributions to the Crowdin JavaScript client. To contribute please do the following:
- Fork the repository on GitHub.
- Decide which code you want to submit. Commit your changes and push to the new branch.
- Ensure that your code adheres to standard conventions, as used in the rest of the library.
- Ensure that there are unit tests for your code.
- Submit a pull request with your patch on Github.
Seeking Assistance
If you find any problems or would like to suggest a feature, please feel free to file an issue on Github at Issues Page.
If you've found an error in these samples, please contact our Support Team.
License
Copyright © 2020 Crowdin
The Crowdin JavaScript client is licensed under the MIT License.
See the LICENSE.md file distributed with this work for additional
information regarding copyright ownership.
Except as contained in the LICENSE file, the name(s) of the above copyright
holders shall not be used in advertising or otherwise to promote the sale,
use or other dealings in this Software without prior written authorization.