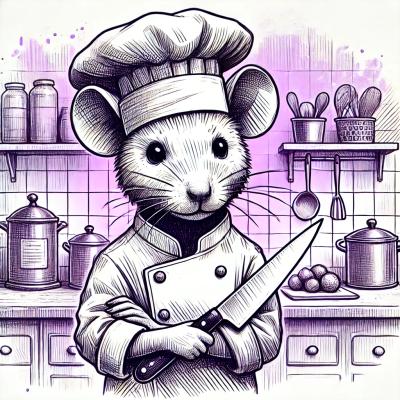
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@cypress/code-coverage
Advanced tools
@cypress/code-coverage is a plugin for Cypress that allows you to measure the code coverage of your application while running end-to-end tests. It integrates with popular code coverage libraries like Istanbul to provide detailed reports on which parts of your codebase are being exercised by your tests.
Setup Code Coverage
This code snippet shows how to set up the @cypress/code-coverage plugin in your Cypress configuration file. It requires the plugin and initializes it with the Cypress event handlers.
module.exports = (on, config) => {
require('@cypress/code-coverage/task')(on, config);
return config;
};
Instrument Application Code
This code demonstrates how to instrument your application code using Istanbul, which is a necessary step for collecting code coverage data. The instrumented code is then used in your application.
const istanbul = require('istanbul-lib-instrument');
const instrumenter = istanbul.createInstrumenter();
const instrumentedCode = instrumenter.instrumentSync(originalCode, filename);
Generate Coverage Reports
This code snippet shows how to generate HTML coverage reports using Istanbul. The coverage data collected during the tests is used to create a coverage map, which is then used to generate the report.
const { createCoverageMap } = require('istanbul-lib-coverage');
const coverageMap = createCoverageMap(global.__coverage__);
const report = require('istanbul-reports').create('html');
const context = require('istanbul-lib-report').createContext({ dir: './coverage' });
report.execute(context);
nyc is a command-line interface for Istanbul, a popular JavaScript code coverage tool. It provides a simple way to instrument your code and generate coverage reports. Unlike @cypress/code-coverage, nyc is not specifically designed for Cypress and is more commonly used for unit testing.
Jest is a JavaScript testing framework that comes with built-in code coverage support. It uses Istanbul under the hood to generate coverage reports. Jest is primarily used for unit and integration testing, whereas @cypress/code-coverage is focused on end-to-end testing with Cypress.
karma-coverage is a Karma plugin that uses Istanbul to generate code coverage reports for your tests. It is designed to work with the Karma test runner, which is often used for unit testing in Angular projects. @cypress/code-coverage, on the other hand, is tailored for use with Cypress.
Saves the code coverage collected during Cypress tests
⚠️ Performance Warning This plugin will slow down your tests. There will be more web application JavaScript code to execute due to instrumentation, and there will be code coverage information to merge and save after each test. Track issue #26 for current progress.
npm install -D @cypress/code-coverage
and its peer dependencies
npm install -D nyc istanbul-lib-coverage cypress
Add to your cypress/support/index.js
file
import '@cypress/code-coverage/support'
Register tasks in your cypress/plugins/index.js
file
module.exports = (on, config) => {
on('task', require('@cypress/code-coverage/task'))
}
If your application is loaded Istanbul-instrumented source code, then the coverage information will be automatically saved into .nyc_output
folder and a report will be generated after the tests finish (even in the interactive mode). Find the LCOV and HTML report in the coverage/lcov-report
folder.
That should be it!
If you test your application code directly from specs
you might want to instrument them and combine unit test code coverage with any end-to-end code coverage (from iframe). You can easily instrument spec files using babel-plugin-istanbul for example.
Install the plugin
npm i -D babel-plugin-istanbul
Set your .babelrc
file
{
"plugins": ["istanbul"]
}
Put the following in cypress/plugins/index.js
file to use .babelrc
file
module.exports = (on, config) => {
on('task', require('@cypress/code-coverage/task'))
on('file:preprocessor', require('@cypress/code-coverage/use-babelrc'))
}
Now the code coverage from spec files will be combined with end-to-end coverage.
If you cannot use .babelrc
for some reason (maybe it is used by other tools?), try pushing babel-plugin-istanbul
directory to browserify plugins list.
module.exports = (on, config) => {
on('task', require('@cypress/code-coverage/task'))
on('file:preprocessor', require('@cypress/code-coverage/use-browserify-istanbul'))
}
You can also instrument your server-side code and produce combined coverage report that covers both the backend and frontend code.
node src/server
then to run instrumented version you can do nyc --silent node src/server
.const express = require('express')
const app = express()
require('@cypress/code-coverage/middleware/express')(app)
Tip: you can register the endpoint only if there is global code coverage object, and you can exclude the middleware code from the coverage numbers
// https://github.com/gotwarlost/istanbul/blob/master/ignoring-code-for-coverage.md
/* istanbul ignore next */
if (global.__coverage__) {
require('@cypress/code-coverage/middleware/express')(app)
}
If you use Hapi server, define the endpoint yourself and return the object
if (global.__coverage__) {
require('@cypress/code-coverage/middleware/hapi')(server)
}
For any other server, define the endpoint yourself and return the coverage object:
if (global.__coverage__) {
// add method "GET /__coverage__" and response with JSON
onRequest = (response) =>
response.sendJSON({coverage: global.__coverage__ })
}
cypress.json
file to let the plugin know where to call to receive the code coverage data from the server. Place it in env.codeCoverage
object:{
"env": {
"codeCoverage": {
"url": "http://localhost:3000/__coverage__"
}
}
}
That should be enough - the code coverage from the server will be requested at the end of the test run and merged with the client-side code coverage, producing a combined report
You can specify custom report folder by adding nyc
object to the package.json
file. For example to save reports to cypress-coverage
folder, use:
{
"nyc": {
"report-dir": "cypress-coverage"
}
}
You can specify custom coverage reporter(s) to use. For example to output text summary and save JSON report in cypress-coverage
folder set in your package.json
folder:
{
"nyc": {
"report-dir": "cypress-coverage",
"reporter": [
"text",
"json"
]
}
}
Tip: find list of reporters here
You can exclude parts of the code or entire files from the code coverage report. See Istanbul guide. Common cases:
When running code only during Cypress tests, the "else" branch will never be hit. Thus we should exclude it from the branch coverage computation:
// expose "store" reference during tests
/* istanbul ignore else */
if (window.Cypress) {
window.store = store
}
Often needed to skip a statement
/* istanbul ignore next */
if (global.__coverage__) {
require('@cypress/code-coverage/middleware/express')(app)
}
Or a particular switch
case
switch (foo) {
case 1: /* some code */; break;
/* istanbul ignore next */
case 2: // really difficult to hit from tests
someCode();
}
See nyc
configuration and include and exclude options. You can include and exclude files using minimatch
patterns in .nycrc
file or using "nyc" object inside your package.json
file.
For example, if you want to only include files in the app
folder, but exclude app/util.js
file, you can set in your package.json
{
"nyc": {
"include": [
"app/**/*.js"
],
"exclude": [
"app/util.js"
]
}
}
babel-plugin-istanbul
during tests.react-scripts
.This plugin uses debug module to output additional logging messages from its task.js file. This can help with debugging errors while saving code coverage or reporting. In order to see these messages, run Cypress from the terminal with environment variable DEBUG=code-coverage
. Example using Unix syntax to set the variable:
$ DEBUG=code-coverage npm run dev
...
code-coverage reset code coverage in interactive mode +0ms
code-coverage wrote coverage file /code-coverage/.nyc_output/out.json +28ms
code-coverage saving coverage report using command: "nyc report --report-dir ./coverage --reporter=lcov --reporter=clover --reporter=json" +3ms
This project is licensed under the terms of the MIT license.
FAQs
Saves the code coverage collected during Cypress tests
The npm package @cypress/code-coverage receives a total of 401,005 weekly downloads. As such, @cypress/code-coverage popularity was classified as popular.
We found that @cypress/code-coverage demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.