KazeJS
A flexible Node.js web framework built with TypeScript, focusing on dependency injection, routing, and middleware management. This package allows easy integration of external dependencies, such as a database, into your application. It supports dynamic route groups, global middleware, schema validation with error handling, and static file serving. With customizable error handlers for general and validation errors, it ensures a smooth development experience for building scalable web applications with type safety and clean architecture.
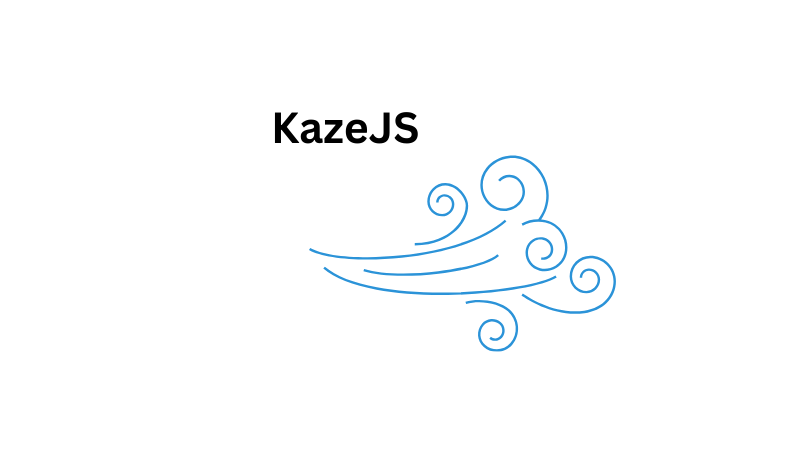
1. Routing [ Static | Dynamic ]
Static Route Example:
app.get("/api/users", async (ctx: KazeContext<Dep>) => {
const users = await ctx.dependencies?.db.query("SELECT * FROM users");
ctx.res.json({ message: "List of users", users });
});
Dynamic Route Example:
app.get("/api/users/:id", async (ctx: KazeContext<Dep, { id: string }>) => {
const userId = ctx.req.params?.id;
const user = await ctx.dependencies?.db.query(`SELECT * FROM users WHERE id=${userId}`);
ctx.res.json({ user });
});
2. Route Grouping [ Static | Dynamic ]
Route Group Example:
const userRouter = Kaze.Router();
userRouter.get("/profile", (ctx: KazeContext<Dep>) => {
ctx.res.json({ message: "User profile" });
});
userRouter.get("/:id", (ctx: KazeContext<Dep, { id: string }>) => {
const userId = ctx.req.params?.id;
ctx.res.json({ userId, message: "User details" });
});
app.routeGrp("/users", userRouter);
3. Middleware Support
Middleware Example:
function authMiddleware(ctx: KazeContext, next: KazeNextFunction) {
console.log("Authentication check...");
next();
}
app.get("/api/protected", authMiddleware, (ctx: KazeContext<Dep>) => {
ctx.res.json({ message: "Access granted" });
});
4. Dependency Injection
Dependency Injection Example:
class UserService {
async findUserById(id: string) {
console.log(`Fetching user with ID: ${id}`);
return { id, name: "John Doe" };
}
}
const dependencies = {
userService: new UserService(),
};
const app = new Kaze({
dependencies,
});
app.get("/api/users/:id", async (ctx: KazeContext<typeof dependencies, { id: string }>) => {
const userId = ctx.req.params?.id;
const user = await ctx.dependencies?.userService.findUserById(userId);
ctx.res.json(user);
});
5. Schema Validation [ Query | Params | JSON Body ]
Query Validation Example:
const ageSchema = Validator.object({
age: Validator.number()
.greaterThanOrEqual(18, "Age must be greater than 18.")
.parse()
});
app.get("/api/validate", queryValidate(ageSchema), (ctx: KazeContext<any, { age: number }>) => {
const age = ctx.req.query?.age;
ctx.res.json({ message: `Age is valid: ${age}` });
});
Params Validation Example:
const ageSchema = Validator.object({
age: Validator.number()
.greaterThanOrEqual(18, "Age must be greater than 18.")
.parse()
});
app.get("/api/:age", paramsValidate(ageSchema), (ctx: KazeContext<any, any, { age: number }>) => {
const age = ctx.req.params?.age;
ctx.res.json({ message: `Age is valid: ${age}` });
});
JSON Body Validation Example:
const ageSchema = Validator.object({
age: Validator.number()
.greaterThanOrEqual(18, "Age must be greater than 18.")
.parse()
});
app.get("/api/user", jsonValidate(ageSchema), (ctx: KazeContext<any, any, any, { age: number }>) => {
const age = ctx.req.body?.age;
ctx.res.json({ message: `Age is valid: ${age}` });
});
6. Static File Serving
Static File Example:
app.static("public");
7. Global Middleware Support
Global Middleware Example:
app.addGlobalMiddleware([
Kaze.parseCookies(),
Kaze.parseBody()
]);
app.addGlobalMiddleware(singleGlobalMiddleware);
app.addGlobalMiddleware(anotherGlobalMiddleware);
8. Global Error Handling Support [ Handler Errors | Schema Validation Errors ]
Global Error Handler Example:
app.globalErrorHandler((ctx: KazeContext, err: unknown) => {
if(err instanceof YourCustomThrownError) {
} else {
ctx.res.status(500).send({ error: "Internal Server Error" });
}
});
app.globalVErrorHandler((ctx: KazeContext, err: KazeValidationError) => {
console.log(err.vErrors);
ctx.res.status(400).json({ error: err.message });
});
9. Async Request Handling
Async Request Example:
const sleep = (ms: number) => new Promise(resolve => setTimeout(resolve, ms));
app.get("/api/delay", async (ctx: KazeContext) => {
await sleep(2000);
ctx.res.json({ message: "Response after delay" });
});
async function anyMiddleware(ctx: KazeContext, next: KazeNextFunction) {
await sleep(2000);
next();
}
app.get("/middleware", anyMiddleware, async(ctx: KazeContext) => {
ctx.res.send("works");
});
10. Jwt Support
Jwt Example:
type CustomClaimType = {
role: "admin" | "user"
}
app.get("/login", async (ctx: KazeContext) => {
const jwt = await signJwt({
aud: "http://localhost:4000",
iat: createIssueAt(new Date()),
exp: createExpiry("1h"),
iss: "server-x",
sub: "user"
},
{ role: "admin" },
"itsasecret",
{ alg: "HS512" }
);
ctx.res.json({ token: jwt });
ctx.res.setCookie("token", jwt, {
path: "/",
expires: new Date(Date.now() + 60 * 60 * 1000),
httpOnly: true,
sameSite: "Lax"
})
});
async function auth(ctx: KazeContext, next: KazeNextFunction) {
const token = ctx.req.cookies?.get("token");
try {
const verifiedPayload = await verifyJwt<CustomClaimType>(token,"itsasecret");
next();
} catch {
ctx.res.send("Invalid token");
}
}
app.get("/protected-route", auth, async(ctx: KazeContext) => {
ctx.res.send("works");
});
Error Handling
The verifyJwt
function may throw the following errors:
- DirtyJwtSignature: If the JWT signature doesn't match or is invalid.
- ExpiredJwt: If the token has expired (based on the
exp
claim). - InvalidJwt: If the token is malformed or cannot be decoded properly.
async function auth(ctx: KazeContext, next: KazeNextFunction) {
const jwt = "your.jwt.token";
const secret = "itsasecret";
try {
const verifiedClaims = await verifyJwt(jwt, secret);
console.log(verifiedClaims);
} catch (error) {
if (error instanceof DirtyJwtSignature) {
console.error("Error: JWT signature is invalid or has been tampered with.");
} else if (error instanceof ExpiredJwt) {
console.error("Error: JWT has expired.");
} else if (error instanceof InvalidJwt) {
console.error("Error: JWT is malformed or cannot be decoded.");
} else {
console.error("Unexpected error:", error);
}
}
}
11. CORS Handling
Cors Example:
app.addGlobalMiddleware(cors({
origin: "http://localhost:3000",
allowMethods: ["GET", "POST"],
}));
app.get("/api/endpoint", (ctx: KazeContext) => {
ctx.res.send("hello");
});
12. File Upload
File upload Example:
app.addGlobalMiddleware([
Kaze.fileUpload({
limit: 100,
});
]);
app.post("/submit", (ctx: KazeContext) => {
if(ctx.req.files && ctx.req.files?.length > 0) {
console.log(ctx.req.files?.[0].fileName);
console.log(ctx.req.files?.[0].fileSize);
}
console.log(ctx.req.body)
ctx.res.send("works");
});