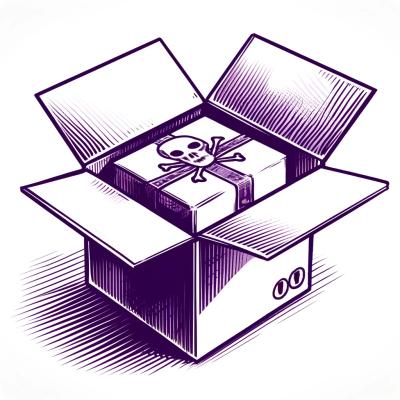
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
@datastructures-js/queue
Advanced tools
A performant queue implementation in javascript.
npm install --save @datastructures-js/queue
const { Queue } = require('@datastructures-js/queue');
import { Queue } from '@datastructures-js/queue';
// empty queue
const queue = new Queue();
// from an array
const queue = new Queue([1, 2, 3]);
// empty queue
const queue = new Queue<number>();
// from an array
const queue = new Queue<number>([1, 2, 3]);
// empty queue
const queue = Queue.fromArray([]);
// with elements
const list = [10, 3, 8, 40, 1];
const queue = Queue.fromArray(list);
// If the list should not be mutated, use a copy of it.
const queue = Queue.fromArray(list.slice());
// empty queue
const queue = Queue.fromArray<number>([]);
// with elements
const list = [10, 3, 8, 40, 1];
const queue = Queue.fromArray<number>(list);
adds an element to the back of the queue.
queue.enqueue(10).enqueue(20); // or queue.push(123)
peeks on the front element of the queue.
console.log(queue.front()); // 10
peeks on the back element in the queue.
console.log(queue.back()); // 20
removes and returns the front element of the queue in O(1) runtime.
console.log(queue.dequeue()); // 10 // or queue.pop()
console.log(queue.front()); // 20
Dequeuing all elements takes O(n) instead of O(n2) when using shift/unshift with arrays.
Explanation by @alexypdu:
Internally, when half the elements have been dequeued, we will resize the dynamic array using Array.slice()
which runs in $O(n)$. Since dequeuing all $n$ elements will resize the array $\log_2n$ times, the complexity is $$1 + 2 + 4 + \cdots + 2^{\log_2 n - 1} = 2 ^ {(\log_2 n - 1) + 1} - 1 = n - 1 = O(n)$$ Hence the overall complexity of dequeuing all elements is $O(n + n) = O(n)$, and the amortized complexity of dequeue()
is thus $O(1)$.
benchmark:
dequeuing 1 million elements in Node v14
Queue.dequeue | Array.shift |
~27 ms | ~4 mins 31 secs |
checks if the queue is empty.
console.log(queue.isEmpty()); // false
returns the number of elements in the queue.
console.log(queue.size()); // 1
creates a shallow copy of the queue.
const queue = Queue.fromArray([{ id: 2 }, { id: 4 } , { id: 8 }]);
const clone = queue.clone();
clone.dequeue();
console.log(queue.front()); // { id: 2 }
console.log(clone.front()); // { id: 4 }
returns a copy of the remaining elements as an array.
queue.enqueue(4).enqueue(2);
console.log(queue.toArray()); // [20, 4, 2]
clears all elements from the queue.
queue.clear();
queue.size(); // 0
grunt build
The MIT License. Full License is here
FAQs
a performant queue implementation in javascript
The npm package @datastructures-js/queue receives a total of 14,973 weekly downloads. As such, @datastructures-js/queue popularity was classified as popular.
We found that @datastructures-js/queue demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.