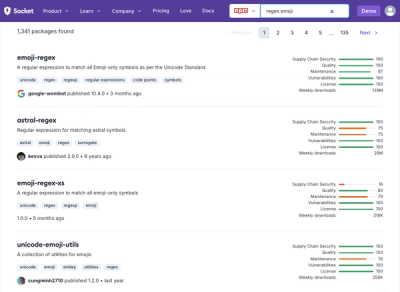
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
@denq/iron-tree
Advanced tools
This package builds a tree and gives a lot of useful methods for managing a tree and its nodes
npm install @denq/iron-tree --save
// create tree
const object = { id: 1, title: 'Root' };
const tree = new Tree(object);
// add nodes
const regularObject = { id:2, title: 'Node 2'}
tree.add((parentNode) => {
return parentNode.get('id') === 1;
}, regularObject);
// contains node
const targetNode = tree.contains((currentNode) => {
return currentNode.get('id') === 2;
});
// remove node
const result = tree.remove((currentNode) => {
return currentNode.get('id') === 2;
});
// traversal
const criteria = (currentNode) => currentNode.get('id') === 1;
tree.traversal(criteria, (currentNode) => {
currentNode.set('some', true);
});
// getPath
const criteria = (currentNode) => currentNode.get('id') === 6;
const targetNode = tree.contains(criteria);
const path = targetNode.getPath();
const pathString = path
.map((item) => item.get('id'))
.join(',');
function compareById(vector) {
return (a, b) => {
const aid = Number(a.get('id'));
const bid = Number(b.get('id'));
if (aid > bid) {
return vector ? 1 : -1;
} else if (aid < bid) {
return vector ? -1 : 1;
} else {
return 0
}
};
}
tree.sort(compareById(false)); // desc
The following are the other methods available.
This is the class of tree management.
Node
contstructor(object)
object
. OptionalIronTree
const object = { id: 1, title: 'Root' };
const tree = new Tree(object);
.add(criteria, object) Adds a node to the tree if the criterion is true.
function
or string
. If string
then criteria is "root"IronTree
const object = { id: 1, title: 'Root' };
const tree = new Tree();
const resultTree = tree.add('root', object);
const regularObject = { id:2, title: 'Node 2'}
const resultTree = tree.add((parentNode) => {
return parentNode.get('id') === 1;
}, regularObject);
.remove(criteria) Removes a node from a tree if the criterion is true.
boolean
boolean
const result = tree.remove((currentNode) => {
return currentNode.get('id') === 7;
});
.contains(criteria) Searches for a node in a tree according to the criterion.
boolean
Node
const targetNode = tree.contains((currentNode) => {
return currentNode.get('id') === 7;
});
.sort(compare) Sorts a tree.
null
function compareById(vector) {
return (a, b) => {
const aid = Number(a.get('id'));
const bid = Number(b.get('id'));
if (aid > bid) {
return vector ? 1 : -1;
} else if (aid < bid) {
return vector ? -1 : 1;
} else {
return 0
}
};
}
tree.sort(compareById(false)); //Desc
.move(criteria, destination) Moves the desired branch or node to the node or branch of the destination, according to the criteria.
boolean
const search = (currentNode) => currentNode.get('id') === 7;
const destination = (currentNode) => currentNode.get('id') === 3;
const result = tree.move(search, destination);
.traversal(criteria, callback) Bypasses the tree and, according to the criterion, calls a function for each node.
boolean
null
const criteria = (currentNode) => currentNode.get('id') === 7;
tree.traversal(criteria, (currentNode) => {
currentNode.set('some', true);
});
tree.traversal(null, (currentNode) => {
if (currentNode.get('id')%2 === 0) {
currentNode.set('some', true);
}
});
.toJson(options) Represents a tree in the form of a json format.
object
. Optional
boolean
. Allow empty children. Default true
string
. Field name for children. Default children
object
const json = tree.toJson();
This is the node management class.
object
array
number
constructor(json)
json
objectconst rootContent = {
id: 1,
name: 'Root',
}
let node = new Node(rootContent);
.add(child) Adding a child to the node.
Node
- created nodeobject
/jsonconst rootContent = {
id: 1,
name: 'Root',
}
let node = new Node(rootContent);
const childNode = node.add({ id: 2, name: 'Two node'});
.remove(criteria) Removing a child node according to the criterion.
Node
const removedNodes = node.remove((itemNode) => {
return itemNode.get('id') === 3;
})
.get(path) Access to node content by field name.
mixed
id
or fullname
, etc... node.get('id'); // 1
node.get('name') // "Some name"
.set(path, value) Setting a value or creating a new field in the contents of a node.
boolean
String
field namemixed
node.set('id', 100)); // returned `true`. Node.content.id = 100
node.get('id'); // 100
.sort(compare) Sorting child nodes
null
function compareById(vector) {
return (a, b) => {
const aid = Number(a.get('id'));
const bid = Number(b.get('id'));
if (aid > bid) {
return vector ? 1 : -1;
} else if (aid < bid) {
return vector ? -1 : 1;
} else {
return 0
}
};
}
node.sort(compareById(false));
.traversal(criteria, callback) Bypassing child nodes according to the criterion and applying function to them.
null
function
criteria each nodesfunction
fire when criteria is true for node// for all nodes
node.traversal(null, (currentNode) => {
const name = currentNode.get('name');
currentNode.set('name', `${name}!`); // Last symbol "!"
});
// only for node.id == 3
node.traversal((currentNode) => currentNode.get('id') === 3, (currentNode) => {
const name = currentNode.get('name');
currentNode.set('name', `${name}!`); // Last symbol "!"
});
.getPath() This method return array Nodes from root node
to current node
. It maybe helpful for breadcrumbs.
Array
const path = targetNode.getPath();
const pathString = path
.map((item) => item.get('id'))
.join(','); // 1,3,4,5,6
npm test
FAQs
Build tree and many method for manipulation
The npm package @denq/iron-tree receives a total of 506 weekly downloads. As such, @denq/iron-tree popularity was classified as not popular.
We found that @denq/iron-tree demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.