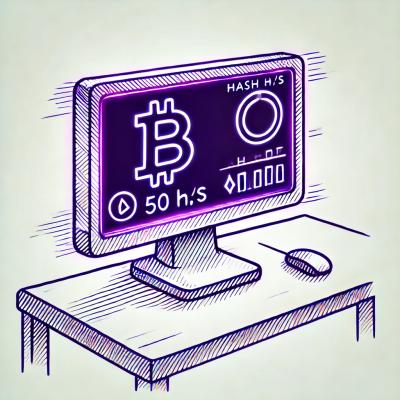
Security News
Research
Supply Chain Attack on Rspack npm Packages Injects Cryptojacking Malware
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
@depay/web3-payments
Advanced tools
JavaScript library to scan crypto wallets for liquefiable assets and calculate most cost-effective payment routing.
yarn add @depay/web3-payments
or
npm install --save @depay/web3-payments
import { route } from '@depay/web3-payments'
let paymentRoutes = await route({
accept: [{
blockchain: 'ethereum',
token: '0xa0bEd124a09ac2Bd941b10349d8d224fe3c955eb',
amount: 20,
toAddress: '0xb0252f13850a4823706607524de0b146820F2240'
}],
from: {
ethereum: '0x317D875cA3B9f8d14f960486C0d1D1913be74e90',
}
})
Routes payment and returns payment routes:
import { route } from '@depay/web3-payments'
let paymentRoutes = await route({
accept: [{
blockchain: 'ethereum',
token: '0xa0bEd124a09ac2Bd941b10349d8d224fe3c955eb',
amount: 20,
toAddress: '0xb0252f13850a4823706607524de0b146820F2240'
}],
from: {
ethereum: '0x5Af489c8786A018EC4814194dC8048be1007e390',
}
})
Also allows to pass in multiple accepted means of payment:
import { route } from '@depay/web3-payments'
let paymentRoutes = await route({
accept: [
{
blockchain: 'ethereum',
token: '0xa0bEd124a09ac2Bd941b10349d8d224fe3c955eb',
amount: 20,
toAddress: '0xb0252f13850a4823706607524de0b146820F2240'
},{
blockchain: 'bsc',
token: '0xa0bEd124a09ac2Bd941b10349d8d224fe3c955eb',
amount: 20,
toAddress: '0xb0252f13850a4823706607524de0b146820F2240'
}
],
from: {
ethereum: '0x5Af489c8786A018EC4814194dC8048be1007e390',
bsc: '0x5Af489c8786A018EC4814194dC8048be1007e390'
}
})
If you want to work with intermediate routing results over waiting for all routes to be calculated, you can use the update
option:
import { route } from '@depay/web3-payments'
let paymentRoutes = await route({
update: {
every: 500,
callback: (currentRoutes){
// yields the current routes every 500ms
}
}
})
In cases where you want to set the fromToken
and fromAmount
(instead of the target token and the target amount) when calculating payment routes you can pass fromToken
, fromAmount
+ toToken
.
Make sure to NOT pass token
nor amount
if you use that option!
import { route } from '@depay/web3-payments'
let paymentRoutes = await route({
accept: [
{
blockchain: 'bsc',
fromAmount: 1,
fromToken: '0xe9e7cea3dedca5984780bafc599bd69add087d56',
toToken: '0xa0bEd124a09ac2Bd941b10349d8d224fe3c955eb',
toAddress: '0xb0252f13850a4823706607524de0b146820F2240'
}
],
from: { bsc: '0x5Af489c8786A018EC4814194dC8048be1007e390' }
})
In case you want to pay into smart contract (calling a smart contract method), you will need to pass toContract
in addition to toAddress
:
import { route } from '@depay/web3-payments'
let paymentRoutes = await route({
accept: [
{
blockchain: 'ethereum',
token: '0xa0bEd124a09ac2Bd941b10349d8d224fe3c955eb',
amount: 20,
toAddress: '0xb0252f13850a4823706607524de0b146820F2240',
toContract: {
signature: 'claim(address,uint256,bool)',
params: ['true']
}
}
],
from: { bsc: '0x5Af489c8786A018EC4814194dC8048be1007e390' }
})
To contract needs to contain at lest the signature
field. Depending on the signature
field params
also need to be provided.
The previous example after swapping payment tokens, will call the contract at 0xb0252f13850a4823706607524de0b146820F2240
calling method claim
passing address
from the payment sender
and amounts from the final token amounts also forwarding params[0]
to pass the value for bool
.
If you want to know more about paying into smart contracts, checkout the depay-evm-router.
Read following the currently supported contract call signatures:
import { route } from '@depay/web3-payments'
let paymentRoutes = await route({
accept: [
{
blockchain: 'ethereum',
token: '0xa0bEd124a09ac2Bd941b10349d8d224fe3c955eb',
amount: 20,
toAddress: '0xb0252f13850a4823706607524de0b146820F2240',
toContract: {
signature: 'claim(address,uint256,bool)',
params: ['true']
}
}
],
from: { bsc: '0x5Af489c8786A018EC4814194dC8048be1007e390' }
})
import { route } from '@depay/web3-payments'
let paymentRoutes = await route({
accept: [
{
blockchain: 'ethereum',
token: '0xa0bEd124a09ac2Bd941b10349d8d224fe3c955eb',
amount: 20,
toAddress: '0xb0252f13850a4823706607524de0b146820F2240',
toContract: {
signature: 'claim(address,uint256,bool)',
params: ['40000000000000000000', 'true']
}
}
],
from: { bsc: '0x5Af489c8786A018EC4814194dC8048be1007e390' }
})
Allows only fromTokens (from the sender) that are part of the whitelist:
let paymentRoutes = await route({
whitelist: {
ethereum: [
'0xEeeeeEeeeEeEeeEeEeEeeEEEeeeeEeeeeeeeEEeE', // ETH
'0xdac17f958d2ee523a2206206994597c13d831ec7', // USDT
'0x6b175474e89094c44da98b954eedeac495271d0f' // DAI
],
bsc: [
'0xEeeeeEeeeEeEeeEeEeEeeEEEeeeeEeeeeeeeEEeE', // BNB
'0xe9e7cea3dedca5984780bafc599bd69add087d56', // BUSD
'0x55d398326f99059ff775485246999027b3197955' // BSC-USD
]
}
})
Filters fromTokens to not be used for payment routing:
let paymentRoutes = await route({
blacklist: {
ethereum: [
'0x6b175474e89094c44da98b954eedeac495271d0f' // DAI
],
bsc: [
'0x55d398326f99059ff775485246999027b3197955' // BSC-USD
]
}
})
Allows to emit events as part of the payment transaction.
Possible values:
ifSwapped
: Only emits an event if payment requires swap, otherwise no dedicated payment event is emited. Use classic transfer event in case of a direct payment (does not go through the DePay router).
let paymentRoutes = await route({
accept: [
{
blockchain: 'ethereum',
token: '0xa0bEd124a09ac2Bd941b10349d8d224fe3c955eb',
amount: 20,
toAddress: '0xb0252f13850a4823706607524de0b146820F2240'
},{
blockchain: 'bsc',
token: '0xa0bEd124a09ac2Bd941b10349d8d224fe3c955eb',
amount: 20,
toAddress: '0xb0252f13850a4823706607524de0b146820F2240'
}
]
event: 'ifSwapped',
from: {
ethereum: '0x5Af489c8786A018EC4814194dC8048be1007e390',
bsc: '0x5Af489c8786A018EC4814194dC8048be1007e390'
}
})
Events are not emitted if payment receiver is a smart contract. Make sure your smart contract emits events in that case!
route
allows you also to configure a fee
that is taken from the payment amount and is sent to another receiver (the fee receiver):
let paymentRoutes = await route({
accept: [...],
fee: {
amount: '3%',
receiver: '0xAb5801a7D398351b8bE11C439e05C5B3259aeC9B'
}
})
// splits 0.3 of the amount paid and sends it to the feeReceiver
fee.amount
can be passed as percentage (String with ending %) or as a BigNumber string or as a pure number/decimal
let paymentRoutes = await route({
accept: [...],
fee: {
amount: '300000000000000000',
receiver: '0xAb5801a7D398351b8bE11C439e05C5B3259aeC9B'
}
})
// splits 0.3 of the amount paid and sends it to the feeReceiver
let paymentRoutes = await route({
accept: [...],
fee: {
amount: 0.3,
receiver: '0xAb5801a7D398351b8bE11C439e05C5B3259aeC9B'
}
})
// splits 0.3 of the amount paid and sends it to the feeReceiver
Exports basic router information (address and api):
import { routers } from '@depay/web3-payments'
routers.ethereum.address // 0xae60aC8e69414C2Dc362D0e6a03af643d1D85b92
Exports plugin addresses:
import { plugins } from '@depay/web3-payments'
plugins.ethereum.payment // 0x99F3F4685a7178F26EB4F4Ca8B75a1724F1577B9
If user has request token in his wallet, direct token transfer to receiver will be prioritized
Any token that can be liquidated on decentralized exchange and has been already been approved for the DePay router will be second priority
All other liquefiable tokens that still require token approval will be prioritized last
Payment routes are provided in the following structure:
{
blockchain: String (e.g. ethereum)
fromToken: Token (see @depay/web3-tokens)
fromBalance: BigNumber (e.g. <BigNumber '10000000000000000000'>)
fromAddress: String (e.g. '0xd8da6bf26964af9d7eed9e03e53415d37aa96045')
fromAmount: BigNumber (e.g. <BigNumber '31000000000000000000'>)
fromDecimals: number (e.g. 18)
toToken: Token (see @depay/web3-tokens)
toAmount: BigNumber (e.g. <BigNumber '21000000000000000000'>)
toDecimals: number (e.g. 18)
toAddress: String (e.g. '0x65aBbdEd9B937E38480A50eca85A8E4D2c8350E4')
exchangeRoutes: Array (list of exchange routes offering to convert )
transaction: Transaction (see @depay/web3-wallets for details)
approvalRequired: Boolean (e.g. true)
approvalTransaction: Transaction (to approve the fromToken being used from the payment router to perform the payment)
directTransfer: Boolean (e.g. true)
}
approvalRequired
: indicates if a upfront token approval is required in order to perform the payment, make sure you execute approve
before executing the payment transaction itself.
directTransfer
: indicates if the payment does not require any swapping/exchanging.
See @depay/web3-wallets for details about the transaction format.
This library supports the following blockchains:
This library supports the following decentralized exchanges:
Soon:
yarn install
yarn dev
npm publish
FAQs
JavaScript library to scan crypto wallets for liquefiable assets and perform cost-effective, auto-converted payments on-chain.
The npm package @depay/web3-payments receives a total of 238 weekly downloads. As such, @depay/web3-payments popularity was classified as not popular.
We found that @depay/web3-payments demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.
Security News
Sonar’s acquisition of Tidelift highlights a growing industry shift toward sustainable open source funding, addressing maintainer burnout and critical software dependencies.