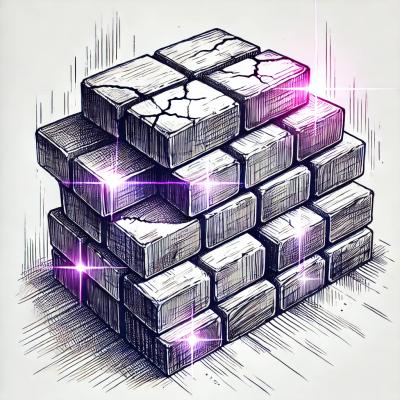
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
@dfinity/utils
Advanced tools
A collection of utilities and constants for NNS/SNS projects.
A collection of utilities and constants for NNS/SNS projects.
You can use utils-js
by installing it in your project.
npm i @dfinity/utils
The bundle needs peer dependencies, be sure that following resources are available in your project as well.
npm i @dfinity/agent @dfinity/candid @dfinity/principal
utils-js
implements following features:
Receives a string representing a number and returns the big int or error.
Function | Type |
---|---|
convertStringToE8s | (value: string) => bigint or FromStringToTokenError |
Parameters:
amount
: - in string formatIs null or undefined
Function | Type |
---|---|
isNullish | <T>(argument: T or null or undefined) => argument is null or undefined |
Not null and not undefined
Function | Type |
---|---|
nonNullish | <T>(argument: T or null or undefined) => argument is NonNullable<T> |
Not null and not undefined and not empty
Function | Type |
---|---|
notEmptyString | (value: string or null or undefined) => boolean |
Get a default agent that connects to mainnet with the anonymous identity.
Function | Type |
---|---|
defaultAgent | () => Agent |
Create an agent for a given identity
Function | Type |
---|---|
createAgent | ({ identity, host, fetchRootKey, verifyQuerySignatures, retryTimes, }: { identity: Identity; host?: string or undefined; fetchRootKey?: boolean or undefined; verifyQuerySignatures?: boolean or undefined; retryTimes?: number or undefined; }) => Promise<...> |
Parameters:
identity
: A mandatory identity to use for the agenthost
: An optional host to connect tofetchRootKey
: Fetch root key for certificate validation during local development or on testnetverifyQuerySignatures
: Check for signatures in the state tree signed by the node that replies to queries - i.e. certify responses.retryTimes
: Set the number of retries the agent should perform before errorring.Function | Type |
---|---|
createServices | <T>({ options: { canisterId, serviceOverride, certifiedServiceOverride, agent: agentOption, callTransform, queryTransform, }, idlFactory, certifiedIdlFactory, }: { options: Required<Pick<CanisterOptions<T>, "canisterId">> and Omit<CanisterOptions<T>, "canisterId"> and Pick<...>; idlFactory: InterfaceFactory; certifiedId... |
Function | Type |
---|---|
assertNonNullish | <T>(value: T, message?: string or undefined) => asserts value is NonNullable<T> |
Function | Type |
---|---|
asNonNullish | <T>(value: T, message?: string or undefined) => NonNullable<T> |
Function | Type |
---|---|
assertPercentageNumber | (percentage: number) => void |
Function | Type |
---|---|
uint8ArrayToBigInt | (array: Uint8Array) => bigint |
Function | Type |
---|---|
bigIntToUint8Array | (value: bigint) => Uint8Array |
Function | Type |
---|---|
numberToUint8Array | (value: number) => Uint8Array |
Function | Type |
---|---|
arrayBufferToUint8Array | (buffer: ArrayBuffer) => Uint8Array |
Function | Type |
---|---|
uint8ArrayToArrayOfNumber | (array: Uint8Array) => number[] |
Function | Type |
---|---|
arrayOfNumberToUint8Array | (numbers: number[]) => Uint8Array |
Function | Type |
---|---|
asciiStringToByteArray | (text: string) => number[] |
Function | Type |
---|---|
hexStringToUint8Array | (hexString: string) => Uint8Array |
Function | Type |
---|---|
uint8ArrayToHexString | (bytes: Uint8Array or number[]) => string |
Function | Type |
---|---|
candidNumberArrayToBigInt | (array: number[]) => bigint |
Encode an Uint8Array to a base32 string.
Function | Type |
---|---|
encodeBase32 | (input: Uint8Array) => string |
Parameters:
input
: The input array to encode.Decode a base32 string to Uint8Array.
Function | Type |
---|---|
decodeBase32 | (input: string) => Uint8Array |
Parameters:
input
: The input string to decode.input
: The base32 encoded string to decode.Function | Type |
---|---|
bigEndianCrc32 | (bytes: Uint8Array) => Uint8Array |
Convert seconds to a human-readable duration, such as "6 days, 10 hours."
Function | Type |
---|---|
secondsToDuration | ({ seconds, i18n, }: { seconds: bigint; i18n?: I18nSecondsToDuration or undefined; }) => string |
Parameters:
options
: - The options object.options.seconds
: - The number of seconds to convert.options.i18n
: - The i18n object for customizing language and units. Defaults to English.Function | Type |
---|---|
debounce | (func: Function, timeout?: number or undefined) => (...args: unknown[]) => void |
Function | Type |
---|---|
toNullable | <T>(value?: T or null or undefined) => [] or [T] |
Function | Type |
---|---|
fromNullable | <T>(value: [] or [T]) => T or undefined |
Function | Type |
---|---|
fromDefinedNullable | <T>(value: [] or [T]) => T |
A parser that interprets revived BigInt, Principal, and Uint8Array when constructing JavaScript values or objects.
Function | Type |
---|---|
jsonReplacer | (_key: string, value: unknown) => unknown |
A function that alters the behavior of the stringification process for BigInt, Principal and Uint8Array.
Function | Type |
---|---|
jsonReviver | (_key: string, value: unknown) => unknown |
Convert a principal to a Uint8Array 32 length. e.g. Useful to convert a canister ID when topping up cycles with the Cmc canister
Function | Type |
---|---|
principalToSubAccount | (principal: Principal) => Uint8Array |
Parameters:
principal
: The principal that needs to be converted to SubaccountReturns true if the current version is smaller than the minVersion, false if equal or bigger. Tags after patch version are ignored, e.g. 1.0.0-beta.1 is considered equal to 1.0.0.
Function | Type |
---|---|
smallerVersion | ({ minVersion, currentVersion, }: { minVersion: string; currentVersion: string; }) => boolean |
Parameters:
params.minVersion
: Ex: "1.0.0"params.currentVersion
: Ex: "2.0.0"Constant | Type |
---|---|
E8S_PER_TOKEN | bigint |
Constant | Type |
---|---|
ICPToken | Token |
Deprecated. Use TokenAmountV2 instead which supports decimals !== 8.
Represents an amount of tokens.
Initialize from a bigint. Bigint are considered e8s.
Method | Type |
---|---|
fromE8s | ({ amount, token, }: { amount: bigint; token: Token; }) => TokenAmount |
Parameters:
params.amount
: The amount in bigint format.params.token
: The token type.Initialize from a string. Accepted formats:
1234567.8901 1'234'567.8901 1,234,567.8901
Method | Type |
---|---|
fromString | ({ amount, token, }: { amount: string; token: Token; }) => FromStringToTokenError or TokenAmount |
Parameters:
params.amount
: The amount in string format.params.token
: The token type.Initialize from a number.
1 integer is considered E8S_PER_TOKEN
Method | Type |
---|---|
fromNumber | ({ amount, token, }: { amount: number; token: Token; }) => TokenAmount |
Parameters:
params.amount
: The amount in number format.params.token
: The token type.Method | Type |
---|---|
toE8s | () => bigint |
Represents an amount of tokens.
Initialize from a bigint. Bigint are considered ulps.
Method | Type |
---|---|
fromUlps | ({ amount, token, }: { amount: bigint; token: Token; }) => TokenAmountV2 |
Parameters:
params.amount
: The amount in bigint format.params.token
: The token type.Initialize from a string. Accepted formats:
1234567.8901 1'234'567.8901 1,234,567.8901
Method | Type |
---|---|
fromString | ({ amount, token, }: { amount: string; token: Token; }) => FromStringToTokenError or TokenAmountV2 |
Parameters:
params.amount
: The amount in string format.params.token
: The token type.Initialize from a number.
1 integer is considered 10^{token.decimals} ulps
Method | Type |
---|---|
fromNumber | ({ amount, token, }: { amount: number; token: Token; }) => TokenAmountV2 |
Parameters:
params.amount
: The amount in number format.params.token
: The token type.Method | Type |
---|---|
toUlps | () => bigint |
Method | Type |
---|---|
toE8s | () => bigint |
FAQs
A collection of utilities and constants for NNS/SNS projects.
The npm package @dfinity/utils receives a total of 3,195 weekly downloads. As such, @dfinity/utils popularity was classified as popular.
We found that @dfinity/utils demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.