Abacus
Shared front-end and mobile logic written in Kotlin Multiplatform (https://kotlinlang.org/docs/multiplatform.html).
The library generates Swift framework for iOS, JVM library for Android, and Javascript code for Web.
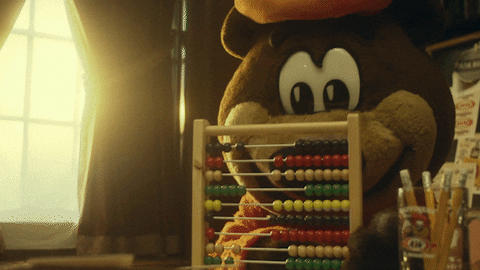
Install Java 11
https://www.oracle.com/java/technologies/downloads/#java11
iOS
Abacus uses Cocoapods to integrate with iOS project. The gradle configuration contains the steps needed to generate the .podspec file. Run
./gradlew podspec
to generate abacus.podspec. Configure your iOS project (https://github.com/dydxprotocol/native-ios) to import abacus.podspec.
You can also build the Abacus for iOS by running:
./gradlew assembleXCFramework
This generates the iOS framework in build/XCFrameworks folder.
Debugging on iOS directly from XCode is possible with a plugin (https://github.com/touchlab/xcode-kotlin)
Android
Abacus builds and pushes the JVM target to MavenLocal repo with the followinng command:
./gradlew publishToMavenLocal
The Android app (https://github.com/dydxprotocol/native-android) has the Gradle build step to pull the Abacus target from MavenLocal.
Web
Abacuas generates Javascript and Typescript files with the following command:
./gradlew assembleJsPackage
This outputs into build/distributions, and references the packages in the build/js directory.
Sample integration from a html page can be find in integration/Web.
Publishing to NPM
Abacus publishes using a library (https://github.com/mpetuska/npm-publish) with the following steps.
- replace the 'obfuscated' with an npm API_KEY (todo: automate this / hide key) and bump version
-
./gradlew assembleJsPackage
-
./gradlew packJsPackage
-
./gradlew publishJsPackageToNpmjsRegistry
- Check published version (https://www.npmjs.com/package/@dydxprotocol/abacus)
Unit Tests
Shared code should have unit tests written in Kotlin residing in the src/CommonTest directory. Run the tests with the following command
./gradlew test
Integration Tests
Integration tests can be written to call Abacus from non-Kotlin code (i.e., Swift, JS). Sample integration projects can be found in the integration directory.
Version Bump
- Update the version in build.gradle.kts
- Update the version in abacus.podspec
- Make sure you have Cocoapod installed. In Terminal, go the {project}/integration/iOS, run "pod update".
- This will update the pod version for iOS Integration app
- in Xcode, open {project}/integraiton/iOS/abacus.ios.xcworkspace. Build and run.
- The integration app doesn't have any UI to indicate the connections. Use Charles to check network traffic. It should contain the standard initial connections to
- wss://api.dydx.exchange/v3/ws, with subscriptions to "v3_markets", "v3_trades", and "v3_orderbook"
- https://api.dydx.exchange/v3/config
How to use
// create a state machine
val stateMachine = PerpTradingStateMachine()
// send socket payload to the state machine and get the state
// the param is the complete socket text
val state = stateMachine.socket(payloadText)
// See src/commonTest/kotlin/exchange.dydx.abacus/PerpV3Tests.kt for testing code