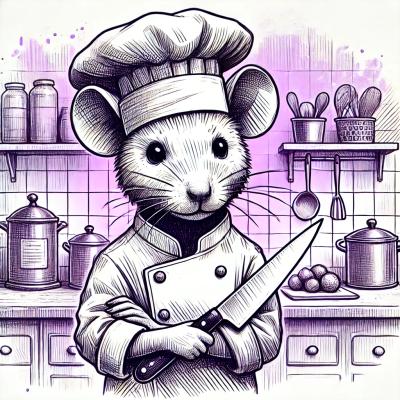
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@fingerprintjs/fingerprintjs
Advanced tools
Browser fingerprinting library with the highest accuracy and stability
@fingerprintjs/fingerprintjs is a JavaScript library that provides a way to uniquely identify users based on their browser and device characteristics. It is commonly used for fraud prevention, user authentication, and personalization.
Generate a Visitor Identifier
This feature allows you to generate a unique identifier for a visitor based on their browser and device characteristics. The code sample demonstrates how to load the FingerprintJS library, generate a visitor identifier, and log it to the console.
const FingerprintJS = require('@fingerprintjs/fingerprintjs');
FingerprintJS.load().then(fp => {
fp.get().then(result => {
const visitorId = result.visitorId;
console.log(visitorId);
});
});
Get Detailed Visitor Data
This feature provides detailed information about the visitor's browser and device characteristics. The code sample shows how to access and log the detailed components of the visitor's fingerprint.
const FingerprintJS = require('@fingerprintjs/fingerprintjs');
FingerprintJS.load().then(fp => {
fp.get().then(result => {
const components = result.components;
console.log(components);
});
});
ClientJS is a JavaScript library for generating a client-side fingerprint based on browser and device characteristics. It offers similar functionality to @fingerprintjs/fingerprintjs but may not be as comprehensive in terms of the data it collects and analyzes.
FingerprintJS2 is an older version of the FingerprintJS library. It provides similar functionality for generating unique visitor identifiers but lacks some of the newer features and improvements found in @fingerprintjs/fingerprintjs.
UA-Parser-JS is a JavaScript library for parsing user-agent strings to identify browser, engine, OS, CPU, and device type/model. While it does not generate a unique visitor identifier, it provides detailed information about the user's environment, which can be used in conjunction with other libraries for fingerprinting.
FingerprintJS is a browser fingerprinting library that queries browser attributes and computes a hashed visitor identifier from them. Unlike cookies and local storage, a fingerprint stays the same in incognito/private mode and even when browser data is purged.
<script>
function initFingerprintJS() {
// Initialize an agent at application startup.
const fpPromise = FingerprintJS.load();
// Get the visitor identifier when you need it.
fpPromise
.then(fp => fp.get())
.then(result => {
// This is the visitor identifier:
const visitorId = result.visitorId;
console.log(visitorId);
});
}
</script>
<script
async
src="//cdn.jsdelivr.net/npm/@fingerprintjs/fingerprintjs@3/dist/fp.min.js"
onload="initFingerprintJS()"
></script>
npm i @fingerprintjs/fingerprintjs
# or
yarn add @fingerprintjs/fingerprintjs
import FingerprintJS from '@fingerprintjs/fingerprintjs';
(async () => {
// Initialize an agent at application startup.
const fpPromise = FingerprintJS.load();
// Get the visitor identifier when you need it.
const fp = await fpPromise
const result = await fp.get();
// This is the visitor identifier:
const visitorId = result.visitorId;
console.log(visitorId);
})();
FingerprintJS Pro is a professional visitor identification service that processes all information server-side and transmits it securely to your servers using server-to-server APIs. Pro combines browser fingerprinting with vast amounts of auxiliary data (IP addresses, time of visit patterns, URL changes and more) to be able to reliably deduplicate different users that have identical devices, resulting in 99.5% identification accuracy.
See our full product comparison for more information on the differences between open source and Pro.
Open Source version | Pro version | |
---|---|---|
Identification accuracy | 60% | 99.5% |
Incognito / Private mode detection | ❌ | ✅ |
Geolocation | ❌ | ✅ |
Security | ❌ | ✅ |
Server API | ❌ | ✅ |
Webhooks | ❌ | ✅ |
Stable identifier between versions | ❌ | ✅ |
Pro result example:
{
"requestId": "HFMlljrzKEiZmhUNDx7Z",
"visitorId": "kHqPGWS1Mj18sZFsP8Wl",
"visitorFound": true,
"incognito": false,
"browserName": "Chrome",
"browserVersion": "85.0.4183",
"os": "Mac OS X",
"osVersion": "10.15.6",
"device": "Other",
"ip": "192.65.67.131",
"ipLocation": {
"accuracyRadius": 100,
"latitude": 37.409657,
"longitude": -121.965467
// ...
}
}
⏱ How to upgrade from Open Source to Pro in 30 seconds
📕 FingerprintJS Pro documentation
▶️ Video: use FingerprintJS Pro to prevent multiple signups
The library is shipped in various formats:
<script>
function initFingerprintJS() {
// Start loading FingerprintJS here
}
</script>
<script
async
src="//cdn.jsdelivr.net/npm/@fingerprintjs/fingerprintjs@3/dist/fp.min.js"
onload="initFingerprintJS()"
></script>
require(
['//cdn.jsdelivr.net/npm/@fingerprintjs/fingerprintjs@3/dist/fp.umd.min.js'],
(FingerprintJS) => {
// Start loading FingerprintJS here
}
);
# Install the package first:
npm i @fingerprintjs/fingerprintjs
# or
yarn add @fingerprintjs/fingerprintjs
import FingerprintJS from '@fingerprintjs/fingerprintjs';
// Start loading FingerprintJS here
# Install the package first:
npm i @fingerprintjs/fingerprintjs
# or
yarn add @fingerprintjs/fingerprintjs
const FingerprintJS = require('@fingerprintjs/fingerprintjs');
// Start loading FingerprintJS here
FingerprintJS.load({ delayFallback?: number }): Promise<Agent>
Builds an instance of Agent and waits a delay required for a proper operation.
We recommend calling it as soon as possible.
delayFallback
is an optional parameter that sets duration (milliseconds) of the fallback for browsers that don't support requestIdleCallback;
it has a good default value which we don't recommend to change.
agent.get({ debug?: boolean }): Promise<{ visitorId: string, components: object }>
Gets the visitor identifier.
We recommend calling it later, when you really need the identifier, to increase the chance of getting an accurate identifier.
debug: true
prints debug messages to the console.
visitorId
is the visitor identifier.
components
is a dictionary of components that have formed the identifier;
each value is an object like { value: any, duration: number }
in case of success
and { error: object, duration: number }
in case of an unexpected error during getting the component.
See the extending guide to learn how to remove and add entropy components.
FingerprintJS.hashComponents(components: object): string
Converts a dictionary of components (described above) into a short hash string a.k.a. a visitor identifier. Designed for extending the library with your own components.
FingerprintJS.componentsToDebugString(components: object): string
Converts a dictionary of components (described above) into human-friendly format.
The OSS version doesn't guarantee the same visitor identifier between versions, but will try to keep them the same as much as possible. To get identifiers that remain stable up to 1 year, please consider upgrading to pro.
The documented JS API follows Semantic Versioning. Use undocumented features at your own risk.
npx browserslist "cover 95% in us, not IE < 10"
See more details and learn how to run the library in old browsers in the documentation article.
See the contributing guidelines to learn how to start a playground, test and build.
FAQs
Browser fingerprinting library with the highest accuracy and stability
The npm package @fingerprintjs/fingerprintjs receives a total of 257,799 weekly downloads. As such, @fingerprintjs/fingerprintjs popularity was classified as popular.
We found that @fingerprintjs/fingerprintjs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.