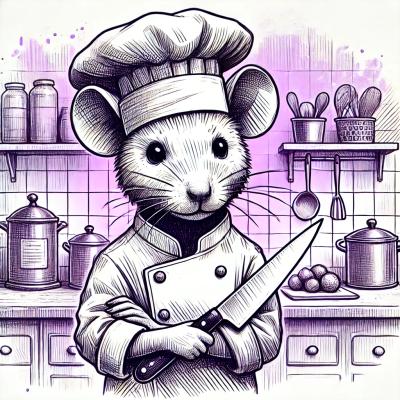
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@imgix/gatsby
Advanced tools
@imgix/gatsby
is a multi-faceted plugin to help the developer use imgix with Gatsby.
ixlib
Param on Every Request?Before you get started with this library, it's highly recommended that you read Eric Portis' seminal article on srcset
and sizes
. This article explains the history of responsive images in responsive design, why they're necessary, and how all these technologies work together to save bandwidth and provide a better experience for users. The primary goal of this library is to make these tools easier for developers to implement, so having an understanding of how they work will significantly improve your experience with this library.
Below are some other articles that help explain responsive imagery, and how it can work alongside imgix:
Integrating imgix with Gatsby provides a few key advantages over the core image experience in Gatsby:
Firstly, this library requires an imgix account, so please follow this quick start guide if you don't have an account.
Then, install this library with the following commands, depending on your package manager.
npm install @imgix/gatsby
yarn add @imgix/gatsby
Finally, check out the section in the usage guide below that most suits your needs.
To find what part of this usage guide you should read, select the use case below that best matches your use case:
<img>
element, with blur-up support 👉 graphql imgixImage
apiimgixImage
APIThis feature can be best thought about as a part-replacement for gatsby-image-sharp, and allows imgix URLs to be used with gatsby-image through the Gatsby GraphQL API. This feature transforms imgix URLs into a format that is compatible with gatsby-image. This can generate either fluid or fixed images. With this feature you can either display images that already exist on imgix, or proxy other images through imgix.
This feature supports many of the existing gatsby-image GraphQL that you know and love, and also supports most of the features of gatsby-image, such as blur-up and lazy loading. It also brings all of the great features of imgix, including the extensive image transformations and optimisations, as well as the excellent imgix CDN.
This source must be configured in your gatsby-config
file as follows:
module.exports = {
//...
plugins: [
// your other plugins here
{
resolve: `@imgix/gatsby`,
options: {
domain: '<your imgix domain, e.g. acme.imgix.net>',
defaultImgixParams: ['auto', 'format'],
},
},
],
};
Setting auto: ['format', 'compress']
is highly recommended. This will re-format the image to the format that is best optimized for your browser, such as WebP. It will also reduce unnecessary wasted file size, such as transparency on a non-transparent image. More information about the auto parameter can be found here.
The following code will render a fluid image with gatsby-image. This code should already be familiar to you if you've used gatsby-image in the past.
import gql from 'graphql-tag';
import Img from 'gatsby-image';
export default ({ data }) => {
return <Img fluid={{ ...data.imgixImage.fluid, sizes: '100vw' }} />;
};
export const query = gql`
{
imgixImage(url: "/image.jpg") {
fluid(imgixParams: {
// pass any imgix parameters you want to here
}) {
...GatsbySourceImgixFluid
}
}
}
`;
A full list of imgix parameters can be found here.
Although sizes
is optional, it is highly recommended. It has a default of (max-width: 8192px) 100vw, 8192px
, which means that it is most likely loading an image too large for your users. Some examples of what you can set sizes as are:
500px
- the image is a fixed width. In this case, you should use fixed mode, described in the next section.(min-width: 1140px) 1140px, 100vw
- under 1140px, the image is as wide as the viewport. Above 1140px, it is fixed to 1140px.The following code will render a fixed image with gatsby-image. This code should already be familiar to you if you've used gatsby-image in the past.
import gql from 'graphql-tag';
import Img from 'gatsby-image';
export default ({ data }) => {
return <Img fixed={data.imgixImage.fixed} />;
};
export const query = gql`
{
imgixImage(url: "/image.jpg") {
fixed(
width: 960 # Width (in px) is required
imgixParams: {}
) {
...GatsbySourceImgixFixed
}
}
}
`;
A full list of imgix parameters can be found here.
If you would rather not use gatsby-image and would instead prefer just a plain imgix URL, you can use the url
field to generate one. For instance, you could generate a URL and use it for the background image of an element:
import gql from 'graphql-tag';
export default ({ data }) => {
return (
<div
style={{
backgroundImage: `url(${data.imgixImage.url})`,
backgroundSize: 'contain',
width: '100vw',
height: 'calc(100vh - 64px)',
}}
>
<h1>Blog Title</h1>
</div>
);
};
export const query = gql`
{
imgixImage(url: "/image.jpg") {
url(imgixParams: { w: 1200, h: 800 })
}
}
`;
If you would like to proxy images from another domain, you should set up a Web Proxy Source. After doing this, you can use this source with this plugin as follows:
gatsby-config.js
to the following:module.exports = {
//...
plugins: [
// your other plugins here
{
resolve: `@imgix/gatsby-source-url`,
options: {
domain: '<your proxy source domain, e.g. my-proxy-source.imgix.net>',
secureURLToken: '...', // <-- now required, your "Token" from your source page
defaultImgixParams: ['auto', 'format'],
},
},
],
};
url
parameter in the GraphQL API. For example, to render a fixed image, a page would look like this:import gql from 'graphql-tag';
import Img from 'gatsby-image';
export default ({ data }) => {
return <Img fixed={data.imgixImage.fixed} />;
};
export const query = gql`
{
imgixImage(url: "https://acme.com/my-image-to-proxy.jpg") {
# Now this is a full URL
fixed(
width: 960 # Width (in px) is required
) {
...GatsbySourceImgixFixed
}
}
}
`;
This features allows imgix urls to be used with gatsby-image. This feature transforms imgix urls into a format that is compatible with gatsby-image. This can generate either fluid or fixed images. With this feature you can either display images that already exist on imgix, or proxy other images through imgix.
Unfortunately, due to limitations of Gatsby, this feature does not support the placeholder/blur-up feature yet. When Gatsby removes this limitation, we plan to implement this for this feature. In the meantime, our other Gatsby plugins will support blur-up/placeholder images, so if this feature is critical to you, please consider using one of those.
The following code will render a fluid image with gatsby-image. This code should already be familiar to you if you've used gatsby-image in the past.
import Img from 'gatsby-image';
import { buildFluidImageData } from '@imgix/gatsby';
// Later, in a gatsby page/component.
<Img
fluid={buildFluidImageData(
'https://assets.imgix.net/examples/pione.jpg',
{
// imgix parameters
ar: 1.61, // required
auto: ['format', 'compress'], // recommended for all images
// pass other imgix parameters here, as needed
},
{
sizes: '50vw', // optional, but highly recommended
},
)}
/>;
ar
is required, since gatsby-image requires this to generate placeholders. This ar
will also crop the rendered photo from imgix to the same aspect ratio. If you don't know the aspect ratio of your image beforehand, it is virtually impossible to use gatsby-image in this format, so we either recommend using another of our plugins, or using an img
directly.
Setting auto: ['format', 'compress']
is highly recommended. This will re-format the image to the format that is best optimized for your browser, such as WebP. It will also reduce unnecessary wasted file size, such as transparency on a non-transparent image. More information about the auto parameter can be found here.
A full list of imgix parameters can be found here.
Although sizes
is optional, it is highly recommended. It has a default of 100vw
, which means that it might be loading an image too large for your users. Some examples of what you can set sizes as are:
500px
- the image is a fixed width. In this case, you should use fixed mode, described in the next section.(min-width: 1140px) 1140px, 100vw
- under 1140px, the image is as wide as the viewport. Above 1140px, it is fixed to 1140px.A full example of a fluid image in a working Gatsby repo can be found on CodeSandbox.
The following code will render a fixed image with gatsby-image. This code should already be familiar to you if you've used gatsby-image in the past.
import Img from 'gatsby-image';
import { buildFixedImageData } from '@imgix/gatsby';
// Later, in a gatsby page/component.
<Img
fluid={buildFixedImageData('https://assets.imgix.net/examples/pione.jpg', {
// imgix parameters
w: 960, // required
h: 540, // required
auto: ['format', 'compress'], // recommended for all images
// pass other imgix parameters here, as needed
})}
/>;
The imgix parameters w
and h
are required, since these are used by gatsby-image to display a placeholder while the image is loading. Other imgix parameters can be added below the width and height.
Setting auto: ['format', 'compress']
is highly recommended. This will re-format the image to the format that is best optimized for your browser, such as WebP. It will also reduce unnecessary wasted file size, such as transparency on a non-transparent image. More information about the auto parameter can be found here.
A full list of imgix parameters can be found here.
An example of this mode in a full working Gatsby repo can be found on CodeSandbox.
The majority of the API for this library can be found by using the GraphiQL inspector (usually at https://localhost:8000/__graphql
).
This library also provides some GraphQL fragments, such as GatsbySourceImgixFluid
, and GatsbySourceImgixFluid_noBase64
. The values of these fragments can be found at fragments.js
The plugin options that can be specified in gatsby-config.js
are:
Name | Type | Required | Description |
---|---|---|---|
domain | String | ✔️ | The imgix domain to use for the image URLs. Usually in the format .imgix.net |
defaultImgixParams | Object | Imgix parameters to use by default for every image. Recommended to set to { auto: ['compress', 'format'] } . | |
disableIxlibParam | Boolean | Set to true to remove the ixlib param from every request. See this section for more information. | |
secureURLToken | String | When specified, this token will be used to sign images. Read more about securing images on the imgix Docs site. |
buildFixedImageData
function buildFixedImageData(
/**
* An imgix url to transform, e.g. https://yourdomain.imgix.net/your-image.jpg
*/
url: string,
/**
* A set of imgix parameters to apply to the image.
* Parameters ending in 64 will be base64 encoded.
* A full list of imgix parameters can be found here: https://docs.imgix.com/apis/url
* Width (w) and height (h) are required.
*/
imgixParams: { w: number; h: number } & IImgixParams,
/**
* Options that are not imgix parameters.
* Optional.
*/
options?: {
/**
* Disable the ixlib diagnostic param that is added to every url.
*/
includeLibraryParam?: boolean;
},
): {
width: number;
height: number;
src: string;
srcSet: string;
srcWebp: string;
srcSetWebp: string;
};
buildFluidImageData
export function buildFluidImageData(
/**
* An imgix url to transform, e.g. https://yourdomain.imgix.net/your-image.jpg
*/
url: string,
/**
* A set of imgix parameters to apply to the image.
* Parameters ending in 64 will be base64 encoded.
* A full list of imgix parameters can be found here: https://docs.imgix.com/apis/url
* The aspect ratio (ar) as a float is required.
*/
imgixParams: {
/**
* The aspect ratio to set for the rendered image and the placeholder.
* Format: float or string. For float, it can be calculated with ar = width/height. For a string, it should be in the format w:h.
*/
ar: number | string;
} & IImgixParams,
/**
* Options that are not imgix parameters.
* Optional.
*/
options?: {
/**
* Disable the ixlib diagnostic param that is added to every url.
*/
includeLibraryParam?: boolean;
/**
* The sizes attribute to set on the underlying image.
*/
sizes?: string;
},
): {
aspectRatio: number;
src: string;
srcSet: string;
srcWebp: string;
srcSetWebp: string;
sizes: string;
};
ixlib
Param on Every Request?For security and diagnostic purposes, we tag all requests with the language and version of library used to generate the URL.
To disable this, set includeLibraryParam
in the third parameter to false
when calling one of the two functions this library exports. For example, for buildFluidImageData
:
<Img
fluid={buildFixedImageData(
'https://assets.imgix.net/examples/pione.jpg',
{
w: 960,
h: 540,
},
{
includeLibraryParam: false, // this disables the ixlib parameter
},
)}
/>
📣 Have your say on our roadmap below!
Hey there! Thanks for checking out this repository. We are currently deciding on the roadmap for this library, and we'd love your help in showing us what to prioritize, and what you'd like us to build. If you're interested, read on!
Below is a list of issues that contain all the high-level use-cases that we thought apply to Gatbsy developers using imgix. Please check out any issues that are interesting, and help us decide what to build first by voting on those issues that best fit your use case.
Other features:
Contributions are a vital part of this library and imgix's commitment to open-source. We welcome all contributions which align with this project's goals. More information can be found in the contributing documentation.
imgix would like to make a special announcement about the prior work of Angelo Ashmore from Wall-to-Wall Studios on his gatsby plugin for imgix. The code and API from his plugin has made a significant contribution to the codebase and API for imgix's official plugins, and imgix is very grateful that he agreed to collaborate with us.
Thanks goes to these wonderful people (emoji key):
Frederick Fogerty 💻 📖 🚧 | Angelo Ashmore 💻 |
This project follows the all-contributors specification.
FAQs
The official imgix plugin to apply imgix transformations and optimisations to images in Gatsby at build-time or request-time
The npm package @imgix/gatsby receives a total of 3,441 weekly downloads. As such, @imgix/gatsby popularity was classified as popular.
We found that @imgix/gatsby demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 11 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.