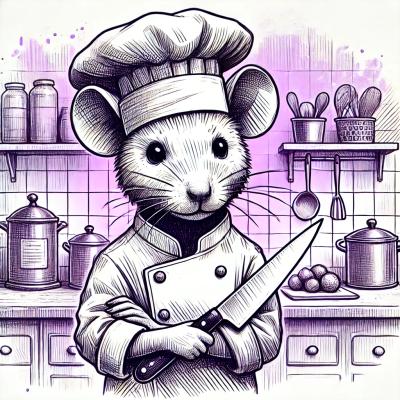
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@infra-design/infra-view
Advanced tools
Work in progress. Not yet ready for public use.
Create your UI in a simple way.
npm install infra-view
yarn add infra-view
pnpm add infra-view
Try to use full spellings instead of abbreviations, which will reduce the mental burden when developing. And we provide intelligent completions, so the time spent using abbreviations and full spellings is not too different.
import { Div } from 'infra-view'
export const App = () => {
return (
<Div
display='block'
margin={100}
width={20}
height={20}
background='black'
hoverStyle={{
background: 'red',
}}
md={{
margin: 200,
width: 30,
height: 30,
}}
lg={{
margin: 300,
width: 30,
height: 30,
}}>
UI
</Div>
)
}
import { Div } from 'infra-view'
const Component = (props) => {
return <Div margin={20} md={{ margin: 30 }} externalProps={props} />
}
export const App = () => {
return (
<Component padding={10} md={{ margin: 20 }}>
UI
</Component>
)
}
import { Div } from 'infra-view'
export const App = () => {
return (
<Div
margin={1}
padding={1}
md={{ margin: 2, padding: 2 }}
lg={{ margin: 3, padding: 3 }}
xl={{ margin: 4, padding: 4 }}
/>
)
}
import { Div } from 'infra-view'
export const App = () => {
return <Div displayName='test'></Div>
}
import { Button } from 'infra-view'
export const App = (props) => {
return (
<Button
color='gray'
background='red'
hoverStyle={{ background: 'blue' }}
activeStyle={{ background: 'yellow' }}
focusStyle={{ borderColor: 'green' }}>
{props.children}
</Button>
)
}
Easy switch between components. Defaults to div
.
import { Div } from 'infra-view'
export const App = () => {
return (
<Div as='div'>
<Div as='button'>Button</Div>
</Div>
)
}
import { P, Span } from 'infra-view'
export const App = () => {
return (
<P>
Same as use <Span>Div</Span> as p.
</P>
)
}
import { Div } from 'infra-view'
export const App = () => {
return (
<>
<Div padding={[1, 2, 3, 4]}></Div>
<Div
text={{
family: 'sans-serif',
size: 16,
align: 'left',
color: 'red',
}}>
Text
</Div>
<Div
background={{
color: 'gray',
image: 'http://www.demo.com/demo.jpg',
repeat: 'no-repeat',
position: 'center',
size: 'cover',
}}>
Background
</Div>
<Div
border={{
color: 'red',
width: 1,
style: 'solid',
}}>
Border
</Div>
</>
)
}
import { Div } from 'infra-view'
export const App = () => {
return (
<>
{/* same as { isMounted && <Div /> } */}
<Div isMounted={true}>isMounted</Div>
{/* same as <div display='none' /> */}
<Div isHidden={true}>isHidden</Div>
</>
)
}
import { Div } from 'infra-view'
export const App = () => {
return (
<>
<Div
border={{
color: 'red',
width: 1,
style: 'solid',
}}
lastChildStyle={{
border: {
color: 'blue',
width: 2,
style: 'dashed',
},
}}>
Last child
</Div>
</>
)
}
We recommend to use Layout
components to create your layout without direct use of Div
component.
import { Flex } from 'infra-view'
export const App = () => {
return (
<Flex
alignItems='center'
justifyContent='space-between'
flexDirection='row'
md={{
flexDirection: 'column',
}}>
<div>1</div>
<div>2</div>
</Flex>
)
}
import { Grid } from 'infra-view'
export const App = () => {
return (
<Grid
columns={1}
gap={20}
md={{ columns: 2, gap: 30 }}
lg={{ columns: 3, gap: 40 }}
xm={{ columns: 4, gap: 50 }}>
<div>1</div>
<div>2</div>
<div>3</div>
<div>4</div>
</Grid>
)
}
import { Center } from 'infra-view'
export const App = () => {
return (
<Center width={20} height={20}>
<div>UI</div>
</Center>
)
}
import { Absolute } from 'infra-view'
export const App = () => {
return (
<Absolute top={10} left={10}>
<div>UI</div>
</Absolute>
)
}
import { Fixed } from 'infra-view'
export const App = () => {
return (
<Fixed top={0} left={0} right={0} height={200}>
<div>UI</div>
</Fixed>
)
}
import { Container, Div } from 'infra-view'
export const App = () => {
return (
<Container
padding={10}
margin={10}
md={{
padding: 20,
margin: 20,
}}
lg={{
padding: 30,
margin: 30,
}}>
<Div>UI</Div>
</Container>
)
}
import { Div, useTheme } from 'infra-view'
export const App = () => {
const { fontSize, lineHeight } = useTheme()
return (
<Div
fontSize={fontSize[0]}
lineHeight={lineHeight[1]}
md={{
fontSize: fontSize[2],
lineHeight: lineHeight[3],
}}>
Theme
</Div>
)
}
import { createTheme, baseTheme } from 'infra-view'
export const theme = createTheme(baseTheme, {
margin: [2, 10, 20, 30],
padding: [10, 20, 30, 40],
fontSize: [12, 14, 16, 18],
lineHeight: [1.5, 1.7, 1.9, 2.1],
breakpoints: [0, 768, 1024, 1440],
})
import theme from 'your-theme'
theme.update({
margin: [1, 2, 3, 4],
})
FAQs
Create your UI in a simple way.
We found that @infra-design/infra-view demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.