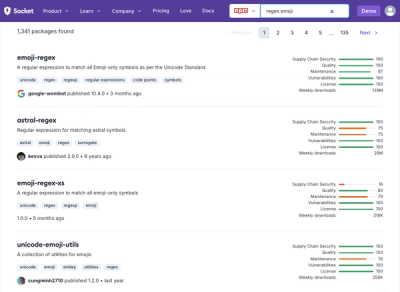
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
@kentico/kontent-management
Advanced tools
Javascript SDK for the Kontent Management. Helps you manage content in your Kentico Kontent projects. Supports both
node.js
andbrowsers
.
To get started, you'll first need to have access to your Kentico Kontent project where you need
to enable Content management API and generate access token
that will be used to authenticate all requests made by this
library.
npm i @kentico/kontent-management --save
If you'd like to use this library directly in browser, place following script tags to your html page. You may of course download it and refer to local versions of scripts.
<script src="https://cdn.jsdelivr.net/npm/@kentico/kontent-management/_bundles/kontent-management.umd.min.js"></script>
The following code example shows how to create new content item in your Kentico Kontent project.
import { ManagementClient } from '@kentico/kontent-management';
const client = new ManagementClient({
projectId: 'xxx', // id of your Kentico Kontent project
apiKey: 'yyy' // Content management API token
});
client
.addContentItem()
.withData({
name: 'New article',
type: {
codename: 'article' // codename of content type
}
})
.toPromise()
.then(response => {
console.log(response);
});
If you are using UMD
bundles directly in browsers, you can find this library under KontentManagement
global
variable.
<!DOCTYPE html>
<html>
<head>
<title>Kontent management | jsdelivr cdn</title>
<script src="https://cdn.jsdelivr.net/npm/@kentico/kontent-management/_bundles/kontent-management.umd.min.js"></script>
</head>
<body>
<script type="text/javascript">
var KontentManagement = window['KontentManagement'];
var client = new KontentManagement.ManagementClient({
projectId: 'xxx',
// using CM API key in browser is NOT safe. If you need to use SDK in browsers
// you should use proxy server and set authorization header there rather than here
apiKey: 'yyy'
});
client
.addContentItem()
.withData({
name: 'New article',
type: {
codename: 'article'
}
})
.toPromise()
.then(response => {
console.log(response);
});
</script>
</body>
</html>
The ManagementClient
contains several configuration options:
const client = new ManagementClient({
// configuration options
});
Option | Default | Description |
---|---|---|
projectId | N/A | Required - Id of your Kentico Kontent project |
apiKey | N/A | Required - Content management API Token |
baseUrl | https://manage.kontent.ai/v2/projects | Base URL of REST api. Can be useful if you are using custom proxy or for testing purposes |
retryStrategy | undefined | Retry strategy configuration. If not set, default strategy is used. |
httpService | HttpService | Used to inject implementation of IHttpService used to make HTTP request across network. Can also be useful for testing purposes by returning specified responses. |
try {
const client = await new ManagementClient({
projectId: 'x',
apiKey: 'y'
});
await client.viewContentItem().byItemCodename('invalid codename').toPromise();
} catch (err) {
if (err instanceof SharedModels.ContentManagementBaseKontentError) {
// Error message provided by API response and mapped by SDK
const message = err.message;
} else {
// handle generic error however you need
}
}
const client = await new ManagementClient({
projectId: 'x',
apiKey: 'y'
});
// prepare cancel token
const cancelTokenRequest = client.createCancelToken();
client
.listContentItems()
.withCancelToken(cancelTokenRequest)
.toPromise()
.then((x) => {
// will not be executed as request was cancelled before network
// request got a chance to finish
})
.catch((err) => {
// error with your custom message 'Request manually cancelled'
});
// cancel request right away
cancelTokenRequest.cancel('Request manually cancelled');
Following is a sample scenario consisting of:
const client = new ManagementClient({
projectId: 'x',
apiKey: 'y'
});
const languages = await client.listLanguages().toPromise();
const defaultLanguage = languages.data.items.find((m) => m.isDefault);
const movieTaxonomy = await client
.addTaxonomy()
.withData({
name: 'Genre',
codename: 'genre',
terms: [
{
name: 'Comedy',
codename: 'comedy',
terms: []
},
{
name: 'Action',
codename: 'action',
terms: []
},
{
name: 'Anime',
codename: 'anime',
terms: []
}
]
})
.toPromise();
const movieType = await client
.addContentType()
.withData((builder) => {
return {
name: 'Movie',
codename: 'movie',
elements: [
builder.textElement({
name: 'Title',
codename: 'title',
type: 'text',
is_required: true,
maximum_text_length: {
applies_to: 'characters',
value: 50
}
}),
builder.dateTimeElement({
name: 'ReleaseDate',
codename: 'release_date',
type: 'date_time',
is_required: true
}),
builder.richTextElement({
name: 'Description',
codename: 'description',
type: 'rich_text',
is_required: true,
allowed_table_formatting: ['unstyled', 'bold', 'italic'],
allowed_blocks: ['text', 'tables'],
maximum_text_length: {
applies_to: 'words',
value: 500
}
}),
builder.assetElement({
name: 'Cover',
codename: 'cover',
type: 'asset',
allowed_file_types: 'adjustable',
asset_count_limit: {
condition: 'exactly',
value: 1
},
is_required: true
}),
builder.taxonomyElement({
type: 'taxonomy',
codename: 'genre',
is_required: true,
taxonomy_group: {
id: movieTaxonomy.data.id
}
})
]
};
})
.toPromise();
const contentItem = await client
.addContentItem()
.withData({
name: 'Warrior',
type: {
codename: 'movie'
}
})
.toPromise();
const imageAsset = await client
.uploadAssetFromUrl()
.withData({
asset: {
descriptions: [{ description: 'Image poster for warrior', language: { id: defaultLanguage?.id ?? '' } }],
title: 'Warrior cover'
},
binaryFile: {
filename: 'warrior.jpg'
},
fileUrl: 'https://upload.wikimedia.org/wikipedia/en/e/e3/Warrior_Poster.jpg'
})
.toPromise();
const languageVariant = await client
.upsertLanguageVariant()
.byItemId(contentItem.data.id)
.byLanguageId(defaultLanguage?.id ?? '')
.withData((builder) => [
builder.textElement({
element: {
codename: 'title'
},
value: 'Warrior'
}),
builder.dateTimeElement({
element: {
codename: 'release_date'
},
value: '2011-09-09'
}),
builder.richTextElement({
element: {
codename: 'description'
},
value: '<p>Path that puts the fighter on a collision course with his estranged, older brother.</p>'
}),
builder.assetElement({
element: {
codename: 'cover'
},
value: [
{
id: imageAsset.data.id
}
]
}),
builder.taxonomyElement({
element: {
codename: 'genre'
},
value: [
{
codename: 'action'
}
]
})
])
.toPromise();
});
If you want to mock http responses, it is possible to use external implementation of configurable Http Service as a part of the client configuration.
If you have any issues or want to share your feedback, please feel free to create an issue in this GitHub repository.
Contributions are welcomed. If you have an idea of what you would like to implement, let us know and lets discuss details of your PR.
FAQs
Official Kentico kontent Content management API SDK
The npm package @kentico/kontent-management receives a total of 283 weekly downloads. As such, @kentico/kontent-management popularity was classified as not popular.
We found that @kentico/kontent-management demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 12 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.