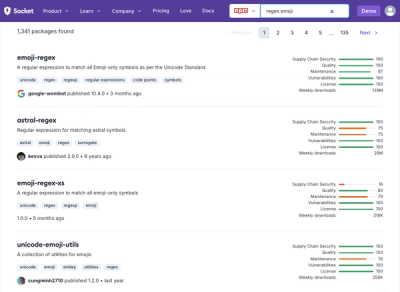
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
@mapbox/point-geometry
Advanced tools
@mapbox/point-geometry is a lightweight library for handling 2D point geometry. It provides a simple API for creating and manipulating points, performing geometric operations, and calculating distances and angles between points.
Creating Points
This feature allows you to create a new point with x and y coordinates. The code sample demonstrates how to create a point at coordinates (10, 20).
const Point = require('@mapbox/point-geometry');
const point = new Point(10, 20);
console.log(point);
Adding Points
This feature allows you to add two points together. The code sample demonstrates how to add point1 (10, 20) and point2 (5, 5) to get a new point (15, 25).
const Point = require('@mapbox/point-geometry');
const point1 = new Point(10, 20);
const point2 = new Point(5, 5);
const result = point1.add(point2);
console.log(result);
Subtracting Points
This feature allows you to subtract one point from another. The code sample demonstrates how to subtract point2 (5, 5) from point1 (10, 20) to get a new point (5, 15).
const Point = require('@mapbox/point-geometry');
const point1 = new Point(10, 20);
const point2 = new Point(5, 5);
const result = point1.sub(point2);
console.log(result);
Calculating Distance
This feature allows you to calculate the Euclidean distance between two points. The code sample demonstrates how to calculate the distance between point1 (10, 20) and point2 (13, 24).
const Point = require('@mapbox/point-geometry');
const point1 = new Point(10, 20);
const point2 = new Point(13, 24);
const distance = point1.dist(point2);
console.log(distance);
Rotating Points
This feature allows you to rotate a point around the origin by a given angle. The code sample demonstrates how to rotate a point (10, 20) by 45 degrees.
const Point = require('@mapbox/point-geometry');
const point = new Point(10, 20);
const angle = Math.PI / 4; // 45 degrees in radians
const rotatedPoint = point.rotate(angle);
console.log(rotatedPoint);
point-cluster is a library for clustering points on a 2D plane. It provides functionality for grouping points that are close to each other. Unlike @mapbox/point-geometry, which focuses on individual point operations, point-cluster is more about managing collections of points.
point-in-polygon is a library for checking if a point is inside a polygon. It provides a simple API for performing point-in-polygon tests. While @mapbox/point-geometry focuses on point operations, point-in-polygon is specialized for spatial queries involving polygons.
turf is a comprehensive geospatial analysis library that includes a wide range of functions for handling points, lines, and polygons. It offers more advanced geospatial operations compared to @mapbox/point-geometry, which is more lightweight and focused on basic point geometry.
a point geometry with transforms
Point(x, y)
A standalone point geometry with useful accessor, comparison, and modification methods.
parameter | type | description |
---|---|---|
x | Number | the x-coordinate. this could be longitude or screen pixels, or any other sort of unit. |
y | Number | the y-coordinate. this could be latitude or screen pixels, or any other sort of unit. |
var point = new Point(-77, 38);
clone
Clone this point, returning a new point that can be modified without affecting the old one.
Returns Point
, the clone
add(p)
Add this point's x & y coordinates to another point, yielding a new point.
parameter | type | description |
---|---|---|
p | Point | the other point |
Returns Point
, output point
sub(p)
Subtract this point's x & y coordinates to from point, yielding a new point.
parameter | type | description |
---|---|---|
p | Point | the other point |
Returns Point
, output point
multByPoint(p)
Multiply this point's x & y coordinates by point, yielding a new point.
parameter | type | description |
---|---|---|
p | Point | the other point |
Returns Point
, output point
divByPoint(p)
Divide this point's x & y coordinates by point, yielding a new point.
parameter | type | description |
---|---|---|
p | Point | the other point |
Returns Point
, output point
mult(k)
Multiply this point's x & y coordinates by a factor, yielding a new point.
parameter | type | description |
---|---|---|
k | Point | factor |
Returns Point
, output point
div(k)
Divide this point's x & y coordinates by a factor, yielding a new point.
parameter | type | description |
---|---|---|
k | Point | factor |
Returns Point
, output point
rotate(a)
Rotate this point around the 0, 0 origin by an angle a, given in radians
parameter | type | description |
---|---|---|
a | Number | angle to rotate around, in radians |
Returns Point
, output point
rotateAround(a, p)
Rotate this point around p point by an angle a, given in radians
parameter | type | description |
---|---|---|
a | Number | angle to rotate around, in radians |
p | Point | Point to rotate around |
Returns Point
, output point
matMult(m)
Multiply this point by a 4x1 transformation matrix
parameter | type | description |
---|---|---|
m | Array.<Number> | transformation matrix |
Returns Point
, output point
unit
Calculate this point but as a unit vector from 0, 0, meaning that the distance from the resulting point to the 0, 0 coordinate will be equal to 1 and the angle from the resulting point to the 0, 0 coordinate will be the same as before.
Returns Point
, unit vector point
perp
Compute a perpendicular point, where the new y coordinate is the old x coordinate and the new x coordinate is the old y coordinate multiplied by -1
Returns Point
, perpendicular point
round
Return a version of this point with the x & y coordinates rounded to integers.
Returns Point
, rounded point
mag
Return the magitude of this point: this is the Euclidean distance from the 0, 0 coordinate to this point's x and y coordinates.
Returns Number
, magnitude
equals(other)
Judge whether this point is equal to another point, returning true or false.
parameter | type | description |
---|---|---|
other | Point | the other point |
Returns boolean
, whether the points are equal
dist(p)
Calculate the distance from this point to another point
parameter | type | description |
---|---|---|
p | Point | the other point |
Returns Number
, distance
distSqr(p)
Calculate the distance from this point to another point, without the square root step. Useful if you're comparing relative distances.
parameter | type | description |
---|---|---|
p | Point | the other point |
Returns Number
, distance
angle
Get the angle from the 0, 0 coordinate to this point, in radians coordinates.
Returns Number
, angle
angleTo(b)
Get the angle from this point to another point, in radians
parameter | type | description |
---|---|---|
b | Point | the other point |
Returns Number
, angle
angleWith(b)
Get the angle between this point and another point, in radians
parameter | type | description |
---|---|---|
b | Point | the other point |
Returns Number
, angle
angleWithSep(x, y)
Find the angle of the two vectors, solving the formula for the cross product a x b = |a||b|sin(θ) for θ.
parameter | type | description |
---|---|---|
x | Number | the x-coordinate |
y | Number | the y-coordinate |
Returns Number
, the angle in radians
convert(a)
Construct a point from an array if necessary, otherwise if the input is already a Point, or an unknown type, return it unchanged
parameter | type | description |
---|---|---|
a | Array.<Number>,Point | any kind of input value |
// this
var point = Point.convert([0, 1]);
// is equivalent to
var point = new Point(0, 1);
Returns Point
, constructed point, or passed-through value.
Requires nodejs.
$ npm install point-geometry
$ npm test
FAQs
a point geometry with transforms
The npm package @mapbox/point-geometry receives a total of 1,385,389 weekly downloads. As such, @mapbox/point-geometry popularity was classified as popular.
We found that @mapbox/point-geometry demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 28 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.