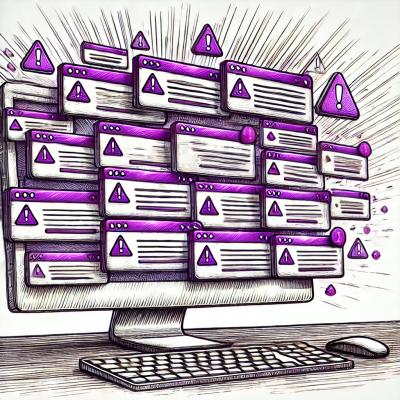
Security News
Combatting Alert Fatigue by Prioritizing Malicious Intent
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
@material/menu-surface
Advanced tools
@material/menu-surface is a package from Material Design Components (MDC) that provides a foundation for creating menu surfaces. These are surfaces that can be used to display a list of choices, such as a dropdown menu or a context menu.
Basic Menu Surface
This code demonstrates how to create a basic menu surface and open it using the MDCMenuSurface class.
import {MDCMenuSurface} from '@material/menu-surface';
const menuSurfaceElement = document.querySelector('.mdc-menu-surface');
const menuSurface = new MDCMenuSurface(menuSurfaceElement);
menuSurface.open();
Anchor Menu Surface
This code shows how to anchor a menu surface to a specific element, making it appear relative to that element.
import {MDCMenuSurface} from '@material/menu-surface';
const menuSurfaceElement = document.querySelector('.mdc-menu-surface');
const menuSurface = new MDCMenuSurface(menuSurfaceElement);
const anchorElement = document.querySelector('.mdc-menu-surface--anchor');
menuSurface.setAnchorElement(anchorElement);
menuSurface.open();
Menu Surface with Event Listeners
This code demonstrates how to add event listeners to a menu surface to handle open and close events.
import {MDCMenuSurface} from '@material/menu-surface';
const menuSurfaceElement = document.querySelector('.mdc-menu-surface');
const menuSurface = new MDCMenuSurface(menuSurfaceElement);
menuSurface.listen('MDCMenuSurface:opened', () => {
console.log('Menu surface opened');
});
menuSurface.listen('MDCMenuSurface:closed', () => {
console.log('Menu surface closed');
});
menuSurface.open();
react-select is a flexible and beautiful Select Input control for ReactJS with multiselect, autocomplete, async and creatable support. It provides a more comprehensive solution for dropdowns and select inputs compared to @material/menu-surface, which is more focused on the menu surface itself.
rc-menu is a React component for creating menus. It offers a wide range of customization options and is highly flexible, making it a good alternative to @material/menu-surface for React applications.
semantic-ui-react is the official React integration for Semantic UI. It includes a variety of UI components, including menus, which are more feature-rich and integrated with the overall Semantic UI framework compared to @material/menu-surface.
The MDC Menu Surface component is a reusable surface that appears above the content of the page and can be positioned adjacent to an element. Menu Surfaces require JavaScript to properly position themselves when opening.
npm install @material/menu-surface
<div class="mdc-menu-surface">
...
</div>
@import "@material/menu-surface/mdc-menu-surface";
import {MDCMenuSurface} from '@material/menu-surface';
const menuSurface = new MDCMenuSurface(document.querySelector('.mdc-menu-surface'));
See Importing the JS component for more information on how to import JavaScript.
The menu surface can be positioned to automatically anchor to a parent element when opened.
<div id="toolbar" class="toolbar mdc-menu-surface--anchor">
<div class="mdc-menu-surface">
...
</div>
</div>
The menu surface can be positioned to automatically anchor to another element, by wrapping the other element with the anchor class.
<div class="mdc-menu-surface--anchor">
<button id="menu-surface-button">Open Menu Surface</button>
<div class="mdc-menu-surface">
...
</div>
</div>
The menu surface can use fixed positioning when being displayed.
<div class="mdc-menu-surface mdc-menu-surface--fixed">
...
</div>
Or in JS:
// ...
menuSurface.setFixedPosition(true);
The menu surface can use absolute positioning when being displayed. This requires that the element containing the
menu(body
if using hoistMenyToBody()
) has the position: relative
style.
<div class="mdc-menu-surface">
...
</div>
// ...
menuSurface.hoistMenuToBody(); // Not required if the menu-surface is already positioned on the body.
menuSurface.setAbsolutePosition(100, 100);
CSS Class | Description |
---|---|
mdc-menu-surface | Mandatory. |
mdc-menu-surface--animating-open | Indicates the menu surface is currently animating open. This class is removed once the animation completes. |
mdc-menu-surface--open | Indicates the menu surface is currently open, or is currently animating open. |
mdc-menu-surface--animating-closed | Indicates the menu surface is currently animating closed. This class is removed once the animation completes. |
mdc-menu-surface--anchor | Used to indicate which element the menu should be anchored to. |
mdc-menu-surface--fixed | Used to indicate that the menu is using fixed positioning. |
Mixin | Description |
---|---|
mdc-menu-surface-ink-color($color) | Sets the color property of the mdc-menu-surface . |
mdc-menu-surface-fill-color($color) | Sets the background-color property of the mdc-menu-surface . |
mdc-menu-surface-corner-radius($radius) | Sets the border-radius property of the mdc-menu-surface . |
MDCMenuSurface
Properties and MethodsProperty | Value Type | Description |
---|---|---|
open | Boolean | Proxies to the foundation's isOpen /(open , close ) methods. |
quickOpen | Boolean | Proxies to the foundation's setQuickOpen() method. |
Method Signature | Description |
---|---|
setAnchorCorner(Corner) => void | Proxies to the foundation's setAnchorCorner(Corner) method. |
setAnchorMargin(AnchorMargin) => void | Proxies to the foundation's setAnchorMargin(AnchorMargin) method. |
setFixedPosition(isFixed: boolean) => void | Adds the mdc-menu-surface--fixed class to the mdc-menu-surface element. Proxies to the foundation's setIsHoisted() and setFixedPosition() methods. |
setAbsolutePosition(x: number, y: number) => void | Proxies to the foundation's setAbsolutePosition(x, y) method. Used to set the absolute x/y position of the menu on the page. Should only be used when the menu is hoisted to the body. |
setMenuSurfaceAnchorElement(element: Element) => void | Changes the element used as an anchor for menu-surface positioning logic. Should be used with conjunction with hoistMenuToBody() . |
hoistMenuToBody() => void | Removes the menu-surface element from the DOM and appends it to the body element. Should be used to overcome overflow: hidden issues. |
setIsHoisted() => void | Proxies to the foundation's setIsHoisted method. |
getDefaultFoundation() => MDCMenuSurfaceFoundation | Returns the foundation. |
Event Name | Data | Description |
---|---|---|
MDCMenuSurface:closed | none | Event emitted after the menu surface is closed. |
MDCMenuSurface:opened | none | Event emitted after the menu surface is opened. |
If you are using a JavaScript framework, such as React or Angular, you can create a Menu Surface for your framework. Depending on your needs, you can use the Simple Approach: Wrapping MDC Web Vanilla Components, or the Advanced Approach: Using Foundations and Adapters. Please follow the instructions here.
MDCMenuSurfaceAdapter
Method Signature | Description |
---|---|
addClass(className: string) => void | Adds a class to the root element. |
removeClass(className: string) => void | Removes a class from the root element. |
hasClass(className: string) => boolean | Returns a boolean indicating whether the root element has a given class. |
hasAnchor: () => boolean | Returns whether the menu surface has an anchor for positioning. |
notifyClose() => void | Dispatches an event notifying listeners that the menu surface has been closed. |
notifyOpen() => void | Dispatches an event notifying listeners that the menu surface has been opened. |
isElementInContainer(el: Element) => Boolean | Returns true if the el Element is inside the mdc-menu-surface container. |
isRtl() => boolean | Returns boolean indicating whether the current environment is RTL. |
setTransformOrigin(value: string) => void | Sets the transform origin for the menu surface element. |
isFocused() => boolean | Returns a boolean value indicating whether the root element of the menu surface is focused. |
saveFocus() => void | Stores the currently focused element on the document, for restoring with restoreFocus . |
restoreFocus() => void | Restores the previously saved focus state, by making the previously focused element the active focus again. |
isFirstElementFocused() => boolean | Returns a boolean value indicating if the first focusable element of the menu-surface is focused. |
isLastElementFocused() => boolean | Returns a boolean value indicating if the last focusable element of the menu-surface is focused. |
focusFirstElement() => void | Focuses the first focusable element of the menu-surface. |
focusLastElement() => void | Focuses the last focusable element of the menu-surface. |
getInnerDimensions() => {width: number, height: number} | Returns an object with the items container width and height. |
getAnchorDimensions() => {width: number, height: number, top: number, right: number, bottom: number, left: number} | Returns an object with the dimensions and position of the anchor (same semantics as DOMRect ). |
getBodyDimensions() => {width: number, height: number} | Returns an object with width and height of the body, in pixels. |
getWindowDimensions() => {width: number, height: number} | Returns an object with width and height of the viewport, in pixels. |
getWindowScroll() => {x: number, y: number} | Returns an object with the amount the body has been scrolled on the x and y axis. |
setPosition(position: {top: string, right: string, bottom: string, left: string}) => void | Sets the position of the menu surface element. |
setMaxHeight(value: string) => void | Sets max-height style for the menu surface element. |
MDCMenuSurfaceFoundation
Method Signature | Description |
---|---|
setAnchorCorner(corder: Corner) => void | Sets the corner that the menu surface will be anchored to. See constants.js |
setAnchorMargin(margin: AnchorMargin) => void | Sets the distance from the anchor point that the menu surface should be shown. |
setIsHoisted(isHoisted: boolean) => void | Sets whether the menu surface has been hoisted to the body so that the offsets are calculated relative to the page and not the anchor. |
setFixedPosition(isFixed: boolean) => void | Sets whether the menu surface is using fixed positioning. |
setAbsolutePosition(x: number, y: numnber) => void | Sets the absolute x/y position of the menu. Should only be used when the menu is hoisted or using fixed positioning. |
handleBodyClick(event: Event) => void | Method used as the callback function for the click event. |
handleKeydown(event: Event) => void | Method used as the callback function for the keydown events. |
open() => void | Opens the menu surface. |
close() => void | Closes the menu. |
isOpen() => boolean | Returns a boolean indicating whether the menu surface is open. |
setQuickOpen(quickOpen: boolean) => void | Sets whether the menu surface should open and close without animation when the open /close methods are called. |
FAQs
The Material Components for the web menu surface component
The npm package @material/menu-surface receives a total of 698,182 weekly downloads. As such, @material/menu-surface popularity was classified as popular.
We found that @material/menu-surface demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 15 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.