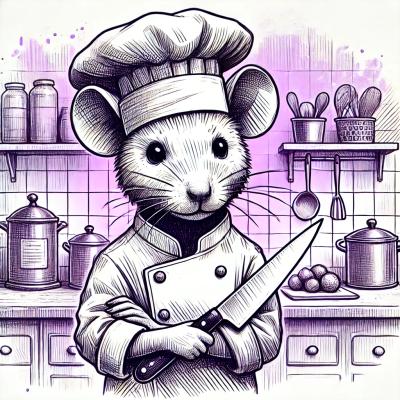
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@microsoft/teamsfx
Advanced tools
TeamsFx aims to reduce the developer tasks of implementing identity and access to cloud resources down to single-line statements with "zero configuration".
Use the library to:
Source code | Package (NPM) | API reference documentation | Samples
TeamsFx SDK is pre-configured in scaffolded project using TeamsFx toolkit or cli. Please check the README to see how to create a Teams App project.
botbuilder
related packages as dependencies, ensure they are of the same version and the version >= 4.9.3
. (Issue - all of the BOTBUILDER packages should be the same version)@microsoft/teamsfx
packageInstall the TeamsFx SDK for TypeScript/JavaScript with npm
:
npm install @microsoft/teamsfx
MicrosoftGraphClient
To create a graph client object to access the Microsoft Graph API, you will need the credential to do authentication. The SDK provides several credential classes to choose that meets various requirements.
Please note that you need to load configuration before using any credentials.
loadConfiguration({
authentication: {
initiateLoginEndpoint: process.env.REACT_APP_START_LOGIN_PAGE_URL,
simpleAuthEndpoint: process.env.REACT_APP_TEAMSFX_ENDPOINT,
clientId: process.env.REACT_APP_CLIENT_ID
}
});
loadConfiguration
. It will load from environment variables by default.loadConfiguration();
Use the snippet below:
Note: You can only use this credential class in browser application like Teams Tab App.
loadConfiguration({
authentication: {
initiateLoginEndpoint: process.env.REACT_APP_START_LOGIN_PAGE_URL,
simpleAuthEndpoint: process.env.REACT_APP_TEAMSFX_ENDPOINT,
clientId: process.env.REACT_APP_CLIENT_ID
}
});
const credential = new TeamsUserCredential();
const graphClient = createMicrosoftGraphClient(credential, ["User.Read"]); // Initializes MS Graph SDK using our MsGraphAuthProvider
const profile = await graphClient.api("/me").get();
It doesn't require the interaction with Teams App user. You can call Microsoft Graph as application. Use the snippet below:
loadConfiguration();
const credential = new M365TenantCredential();
const graphClient = createMicrosoftGraphClient(credential);
const profile = await graphClient.api("/users/{object_id_of_another_people}").get();
There are 3 credential classes that are used to help simplifying authentication. They are located under credential folder.
Credential classes implements TokenCredential
interface that is broadly used in Azure library APIs. They are designed to provide access token for specific scopes.
The credential classes represents different identity under certain scenarios.
TeamsUserCredential
represents Teams current user's identity. Using this credential will request user consent at the first time.
M365TenantCredential
represents Microsoft 365 tenant identity. It is usually used when user is not involved like time-triggered automation job.
OnBehalfOfUserCredential
uses on-behalf-of flow. It needs an access token and you can get a new token for different scope. It's designed to be used in Azure Function or Bot scenarios.
Bot related classes are stored under bot folder.
TeamsBotSsoPrompt
has a good integration with Bot framework. It simplifies the authentication process when you develops bot application.
TeamsFx SDK provides helper functions to ease the configuration for third-party libraries. They are located under core folder.
API will throw ErrorWithCode
if error happens. Each ErrorWithCode
contains error code and error message.
For example, to filter out all errors, you could use the following check:
try {
const credential = new TeamsUserCredential();
const graphClient = createMicrosoftGraphClient(credential, ["User.Read"]); // Initializes MS Graph SDK using our MsGraphAuthProvider
const profile = await graphClient.api("/me").get();
} catch (err) {
// Show login button when specific ErrorWithCode is caught.
if (err instanceof ErrorWithCode && err.code === ErrorCode.UiRequiredError) {
this.setState({
showLoginBtn: true
});
}
}
The following sections provide several code snippets covering some of the most common scenarios, including:
Use TeamsUserCredential
and createMicrosoftGraphClient
.
loadConfiguration({
authentication: {
initiateLoginEndpoint: process.env.REACT_APP_START_LOGIN_PAGE_URL,
simpleAuthEndpoint: process.env.REACT_APP_TEAMSFX_ENDPOINT,
clientId: process.env.REACT_APP_CLIENT_ID
}
});
const credential: any = new TeamsUserCredential();
const graphClient = createMicrosoftGraphClient(credential, ["User.Read"]);
const profile = await graphClient.api("/me").get();
Use axios
library to make HTTP request to Azure Function.
loadConfiguration({
authentication: {
initiateLoginEndpoint: process.env.REACT_APP_START_LOGIN_PAGE_URL,
simpleAuthEndpoint: process.env.REACT_APP_TEAMSFX_ENDPOINT,
clientId: process.env.REACT_APP_CLIENT_ID
}
});
const credential: any = new TeamsUserCredential();
const token = credential.getToken(""); // Get SSO token for the user
// Call API hosted in Azure Functions on behalf of user
const apiConfig = getResourceConfiguration(ResourceType.API);
const response = await axios.default.get(apiConfig.endpoint + "api/httptrigger1", {
headers: {
authorization: "Bearer " + token
}
});
Use tedious
library to access SQL and leverage DefaultTediousConnectionConfiguration
that manages authentication.
Apart from tedious
, you can also compose connection config of other SQL libraries based on the result of sqlConnectionConfig.getConfig()
.
loadConfiguration();
const sqlConnectConfig = new DefaultTediousConnectionConfiguration();
const config = await sqlConnectConfig.getConfig();
const connection = new Connection(config);
connection.on("connect", (error) => {
if (error) {
console.log(error);
}
});
Add TeamsBotSsoPrompt
to dialog set.
const { ConversationState, MemoryStorage } = require('botbuilder');
const { DialogSet, WaterfallDialog } = require('botbuilder-dialogs');
const { TeamsBotSsoPrompt } = require('@microsoft/teamsfx');
const convoState = new ConversationState(new MemoryStorage());
const dialogState = convoState.createProperty('dialogState');
const dialogs = new DialogSet(dialogState);
loadConfiguration();
dialogs.add(new TeamsBotSsoPrompt('TeamsBotSsoPrompt', {
scopes: ["User.Read"],
}));
dialogs.add(new WaterfallDialog('taskNeedingLogin', [
async (step) => {
return await step.beginDialog('TeamsBotSsoPrompt');
},
async (step) => {
const token = step.result;
if (token) {
// ... continue with task needing access token ...
} else {
await step.context.sendActivity(`Sorry... We couldn't log you in. Try again later.`);
return await step.endDialog();
}
}
]));
You can set custome log level and logger when using this library.
The default log level is info
and SDK will print log information to console.
Set log level using the snippet below:
// Only need the warning and error messages.
setLogLevel(LogLevel.Warn);
Set a custome log function if you want to redirect output:
// Only log error message to Application Insights in bot application.
setLogFunction((level: LogLevel, ...args: any[]) => {
if (level === LogLevel.Error) {
const { format, ...rest } = args;
this.telemetryClient.trackTrace({
message: util.format(format, ...rest),
severityLevel: Severity.Error
});
}
});
Set a custome logger instance:
// context.log send messages to Application Insights in Azure Function
setLogger(context.log);
Please take a look at the Samples project for detailed examples on how to use this library.
This project welcomes contributions and suggestions. Most contributions require you to agree to a Contributor License Agreement (CLA) declaring that you have the right to, and actually do, grant us the rights to use your contribution. For details, visit https://cla.microsoft.com.
When you submit a pull request, a CLA-bot will automatically determine whether you need to provide a CLA and decorate the PR appropriately (e.g., label, comment). Simply follow the instructions provided by the bot. You will only need to do this once across all repos using our CLA.
This project has adopted the Microsoft Open Source Code of Conduct. For more information see the Code of Conduct FAQ or contact opencode@microsoft.com with any additional questions or comments.
If you'd like to contribute to this library, please read the contribution guide to learn more about how to build and test the code.
Security issues and bugs should be reported privately, via email, to the Microsoft Security Response Center (MSRC) secure@microsoft.com. You should receive a response within 24 hours. If for some reason you do not, please follow up via email to ensure we received your original message. Further information, including the MSRC PGP key, can be found in the Security TechCenter.
FAQs
Microsoft Teams Framework for Node.js and browser.
The npm package @microsoft/teamsfx receives a total of 12,527 weekly downloads. As such, @microsoft/teamsfx popularity was classified as popular.
We found that @microsoft/teamsfx demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.