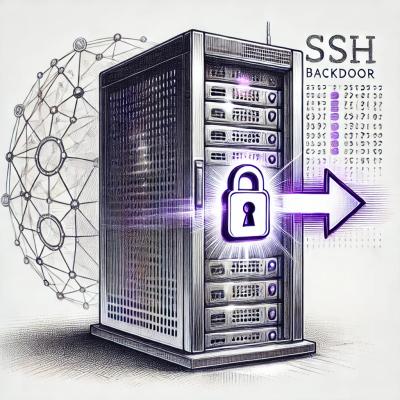
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@nestjs/graphql
Advanced tools
@nestjs/graphql is a module for NestJS that provides integration with GraphQL. It allows developers to build GraphQL APIs using the NestJS framework, leveraging its powerful features like dependency injection, modular architecture, and decorators.
Schema First Approach
In the schema-first approach, you define your GraphQL schema using the SDL (Schema Definition Language) and then implement the resolvers in your code. This approach is useful for teams that prefer to start with the schema design.
const { GraphQLModule } = require('@nestjs/graphql');
const { join } = require('path');
@Module({
imports: [
GraphQLModule.forRoot({
typePaths: ['./**/*.graphql'],
}),
],
})
export class AppModule {}
Code First Approach
In the code-first approach, you define your GraphQL schema using TypeScript decorators and classes. This approach is useful for teams that prefer to start with the implementation and generate the schema automatically.
const { GraphQLModule } = require('@nestjs/graphql');
const { join } = require('path');
@ObjectType()
class User {
@Field()
id: number;
@Field()
name: string;
}
@Resolver(of => User)
class UserResolver {
@Query(returns => User)
getUser() {
return { id: 1, name: 'John Doe' };
}
}
@Module({
imports: [
GraphQLModule.forRoot({
autoSchemaFile: join(process.cwd(), 'src/schema.gql'),
}),
],
providers: [UserResolver],
})
export class AppModule {}
Resolvers
Resolvers are used to define the logic for fetching data for your GraphQL queries. In this example, a simple resolver is created to return a 'Hello World!' string.
const { Resolver, Query } = require('@nestjs/graphql');
@Resolver('User')
class UserResolver {
@Query(() => String)
sayHello() {
return 'Hello World!';
}
}
@Module({
providers: [UserResolver],
})
export class AppModule {}
Mutations
Mutations are used to modify data on the server. In this example, a mutation is created to handle the creation of a new user.
const { Resolver, Mutation, Args } = require('@nestjs/graphql');
@Resolver('User')
class UserResolver {
@Mutation(() => Boolean)
createUser(@Args('name') name: string) {
// Logic to create a user
return true;
}
}
@Module({
providers: [UserResolver],
})
export class AppModule {}
Apollo Server is a community-driven, open-source GraphQL server that works with any GraphQL schema. It is highly flexible and can be used with various Node.js frameworks. Compared to @nestjs/graphql, Apollo Server is more general-purpose and not tied to a specific framework like NestJS.
GraphQL Yoga is a fully-featured GraphQL server with focus on easy setup, performance, and great developer experience. It is built on top of Express and Apollo Server. While @nestjs/graphql is tightly integrated with NestJS, GraphQL Yoga offers a more standalone solution.
TypeGraphQL is a library that allows you to create GraphQL APIs using TypeScript classes and decorators. It is similar to the code-first approach in @nestjs/graphql but is framework-agnostic, meaning it can be used with any Node.js framework or even standalone.
A progressive Node.js framework for building efficient and scalable server-side applications.
GraphQL is a powerful query language for APIs and a runtime for fulfilling those queries with your existing data. It's an elegant approach that solves many problems typically found with REST APIs. For background, we suggest reading this comparison between GraphQL and REST. GraphQL combined with TypeScript helps you develop better type safety with your GraphQL queries, giving you end-to-end typing.
Nest is an MIT-licensed open source project. It can grow thanks to the sponsors and support by the amazing backers. If you'd like to join them, please read more here.
Nest is MIT licensed.
FAQs
Nest - modern, fast, powerful node.js web framework (@graphql)
The npm package @nestjs/graphql receives a total of 617,766 weekly downloads. As such, @nestjs/graphql popularity was classified as popular.
We found that @nestjs/graphql demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.