
New Relic Browser Agent
The New Relic Browser Agent instruments your website and provides observability into the performance and behavior of your application. This NPM Library is an in-progress implementation of the New Relic Browser Agent, accessible through NPM
. Please see the Differences and Features sections to compare how this library differs from the other offerings of the New Relic Browser Agent.
Installation
Using Package Managers
Using npm:
npm install @newrelic/browser-agent
or yarn
yarn add @newrelic/browser-agent
Directly in HTML/JS
Using unpkg
See Using Unpkg for implementation details
ES6 compatible bundle
<script src="https://unpkg.com/@newrelic/browser-agent/dist/bundled/es6/index.js"></script>
ES5 compatible bundle
<script src="https://unpkg.com/@newrelic/browser-agent/dist/bundled/es5/index.js"></script>
Usage Examples
Basic Setup
import NR from '@newrelic/browser-agent'
const options = {
}
const nr = new NR()
nr.start(options).then(() => {
console.log("Browser Agent Initialized!")
})
Notice Errors After Setup
try {
...
} catch (err){
nr.noticeError(err)
}
Setup Using unpkg
<head>
<script src="https://unpkg.com/@newrelic/browser-agent/bundled"></script>
<script>
const { BrowserAgent } = NRBA;
const options = {
}
const agent = new BrowserAgent()
agent.start(options).then(() => {
console.log("Browser Agent Initialized!")
})
</script>
</head>
Notice Errors after Setup
try {
...
} catch (err){
nr.noticeError(err)
}
Instrumenting a Micro Front Ends or Multiple Targets
The New Relic Browser Agent can maintain separate configuration scopes by creating new instances. Separate NR instances can each report their own scoped data to separate New Relic applications.
import { BrowserAgent } from '@newrelic/browser-agent'
const options1 = {
licenseKey: 'abc',
applicationID: '123',
beacon: 'bam.nr-data.net'
}
const agent1 = new BrowserAgent()
agent1.features.errors.enabled = true
agent1.start(options1).then(() => {
console.log("Browser Agent (options1) Initialized!")
})
const options2 = {
licenseKey: 'xyz',
applicationID: '987',
beacon: 'bam.nr-data.net'
}
const agent2 = new BrowserAgent()
agent2.features.errors.auto = false
agent2.start(options2).then(() => {
console.log("Browser Agent (options2) Initialized!")
})
class MyComponent() {
try {
...
} catch(err) {
agent1.noticeError(err)
}
}
class MyComponent() {
try {
...
} catch(err) {
agent2.noticeError(err)
}
}
Configuring your application
The NR interface's start
method accepts an options
object to configure the agent:
const options = {
licenseKey: String
applicationID: String
beacon: String
}
nr.start(options)
Get application ID, license key, beacon
You can find licenseKey
, applicationID
and beacon
values in the New Relic UI's Browser Application Settings page (one.newrelic.com > Browser > (select an app) > Settings > Application settings.)
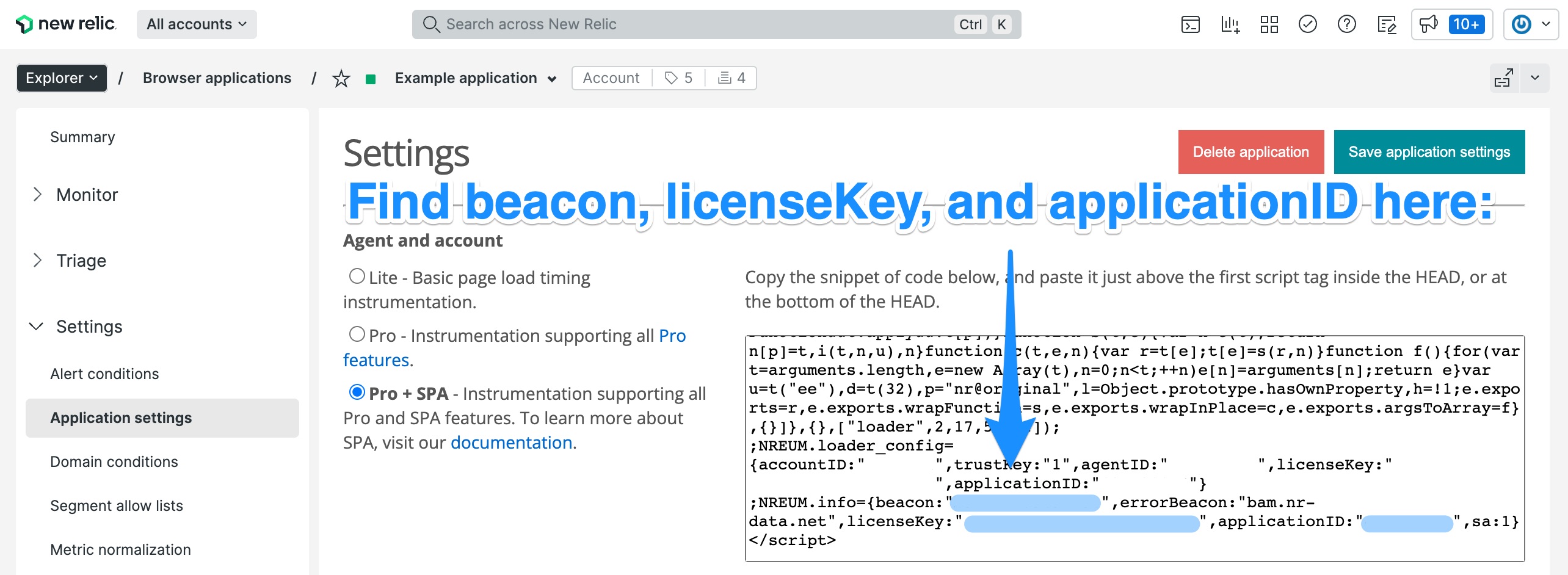
Features
Feature | Subfeature | Default | Description |
---|
errors | enabled | true | Enable's noticeError method |
errors | auto | false | Reports all global errors |
Features must be set before calling the .start() method.
JavaScript Errors
This NPM package can currently capture JavaScript Error reporting in two ways. These errors will be reported to the appId & licenseKey specified in your configuration.
import { BrowserAgent } from '@newrelic/browser-agent'
const browserAgent = new BrowserAgent()
browserAgent.features.errors.enabled = true
browserAgent.features.errors.auto = true
browserAgent.start(options)
Capture JavaScript errors via API
browserAgent.noticeError(new Error())
Set browserAgent.errors.enabled
to true
to report specific errors via the noticeError API.
Automatically capture global JavaScript errors
browserAgent.features.errors.auto = true
Set browserAgent.errors.auto
to true
to report all errors on the page.
Differences
The Browser Agent delivered through NPM will eventually offer parity to its copy/paste and APM counterparts, but during this initial development phase, it should not yet be treated as equivalent. Please see the following table describing the capabilities of each. The availability of these features will change over time.
Feature | APM Injected | Copy/Paste | NPM |
---|
JavaScript Errors | Auto, API | Auto, API | Auto, API |
Page View | Auto | Auto | None |
Page View Timings | Auto | Auto | None |
Ajax Tracking | Auto | Auto | None |
Page Actions | API | API | None |
Session Traces | Auto | Auto | None |
Browser Interactions (SPA) | Auto, API | Auto, API | None |
Multiple Configurable Instances of Browser Agent on One Page | No | No | Yes |
Configurable Code Splitting | No | No | Yes |
IDE Code Completion and Typings | No | No | Yes |
Contributing
We encourage your contributions to improve the Browser agent! Keep in mind that when you submit your pull request, you'll need to sign the CLA via the click-through using CLA-Assistant. You only have to sign the CLA one time per project.
If you have any questions, or to execute our corporate CLA (which is required if your contribution is on behalf of a company), drop us an email at opensource@newrelic.com.
For more details on how best to contribute, see CONTRIBUTING.md
License
The Browser agent is licensed under the Apache 2.0 License.
The Browser agent also uses source code from third-party libraries. Full details on which libraries are used and the terms under which they are licensed can be found in the third-party notices document.