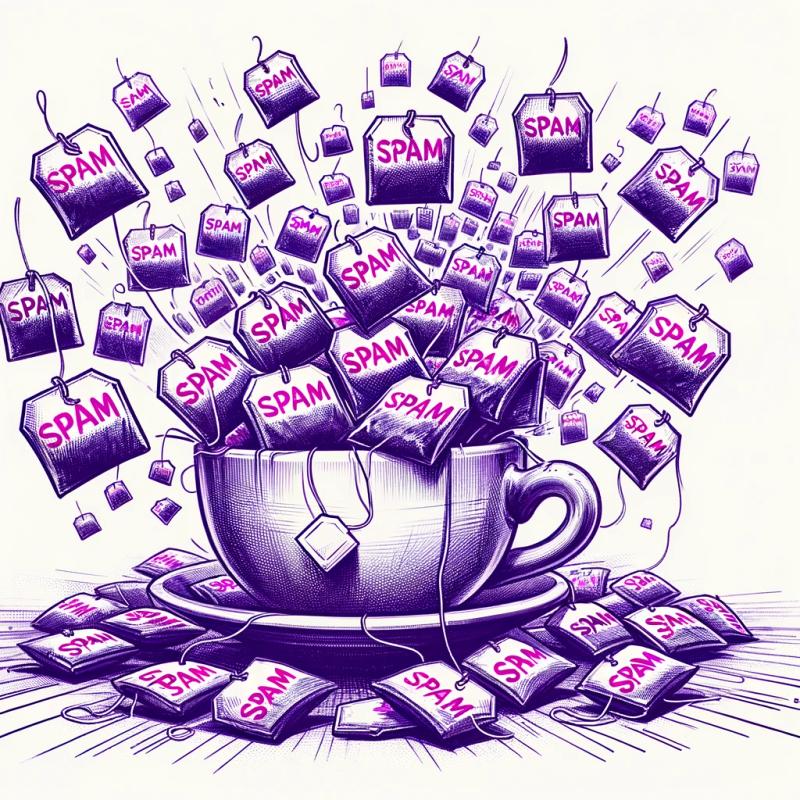
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@ngneat/falso
Advanced tools
Readme
All the Fake Data for All Your Real Needs 🙂
Create massive amounts of fake data in the browser and NodeJS. Tree Shakeable & Fully Typed.
✅ 203 Functions
✅ Tree Shakable
✅ Fully Typed
✅ Factory Functions
✅ Entity Functions
✅ Single and Array Result
🤓 Learn about it on the docs site
Sponsorships aid in the continued development and maintenance of ngneat libraries. Consider asking your company to sponsor ngneat as its core to their business and application development.
Elevate your support by becoming a Gold Sponsor and have your logo prominently featured on our README in the top 5 repositories.
Boost your backing by becoming a Gold Sponsor and enjoy the spotlight with your logo prominently showcased in the top 3 repositories on our README.
Become a bronze sponsor and get your logo on our README on GitHub.
npm i @ngneat/falso
yarn add @ngneat/falso
import { randEmail, randFullName } from '@ngneat/falso';
const user = { email: randEmail(), name: randFullName() };
const emails = randEmail({ length: 10 });
You can specify the length of elements you want to generate. Below is an example of generating 10 emails with length equal or smaller than 20 characters.
const emails = randEmail({ length: 10, maxCharCount: 20 });
You can set your own seed if you want consistent results:
import { rand, seed } from '@ngneat/falso';
seed('some-constant-seed');
// Always returns 2
rand([1, 2, 3, 4, 5]);
// Reset random seed
seed();
npm run c
and choose the right answersFAQs
All the Fake Data for All Your Real Needs
The npm package @ngneat/falso receives a total of 152,888 weekly downloads. As such, @ngneat/falso popularity was classified as popular.
We found that @ngneat/falso demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.