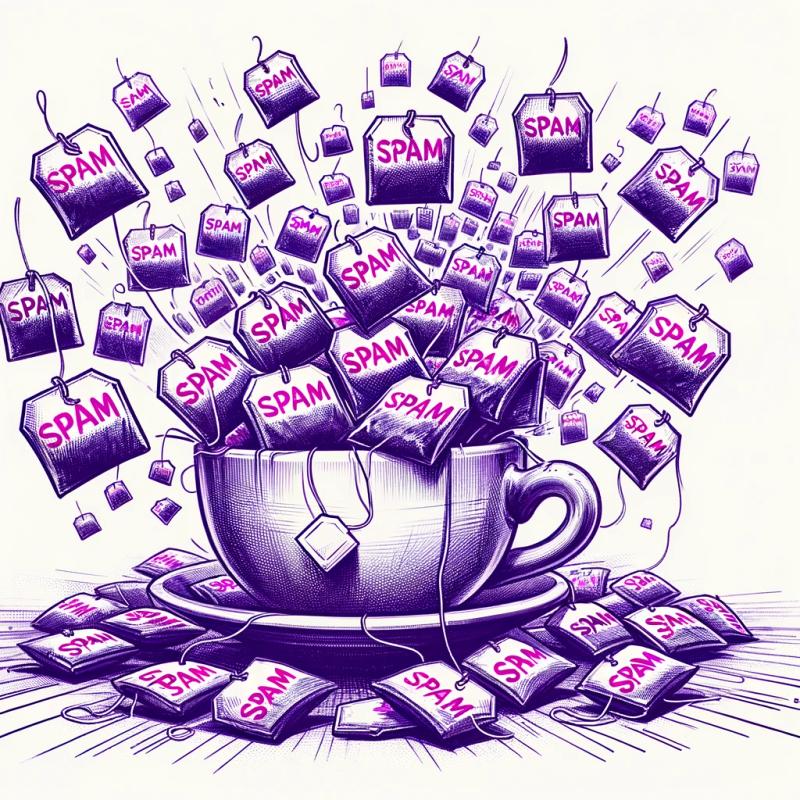
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@nouance/payload-better-fields-plugin
Advanced tools
Readme
This plugin aims to provide you with very specific and improved fields for the admin panel.
We've tried to keep styling as consistent as possible with the existing admin UI, however if there are any issues please report them!
Every field will come with its own usage instructions and structure. These are subject to change!
All fields have had changes to standardise how the field parameters are structured. Field overrides will now be mandatory and come first across fields.
Payload | Better fields |
---|---|
1.x | < 1.0 |
2.x | > 1.0 |
yarn add @nouance/payload-better-fields-plugin
# OR
npm i @nouance/payload-better-fields-plugin
We've tried to re-use the internal components of Payload as much as possible. Where we can't we will re-use the CSS variables used in the core theme to ensure as much compatibility with user customisations.
If you want to further customise the CSS of these components, every component comes with a unique class wrapper in the format of bf<field-name>Wrapper
, eg bfPatternFieldWrapper
, to help you target these inputs consistently.
import { CollectionConfig } from 'payload/types'
import { SlugField } from '@nouance/payload-better-fields-plugin'
const Examples: CollectionConfig = {
slug: 'examples',
admin: {
useAsTitle: 'title',
},
fields: [
{
name: 'title',
type: 'text',
},
...SlugField(
{
name: 'slug',
admin: {
position: 'sidebar',
},
},
{
useFields: ['title', 'subtitle'],
},
),
],
}
The SlugField
accepts the following parameters
overrides
- TextField
required Textfield overrides
config
useFields
- string[]
defaults to ['title']
appendOnDuplication
- boolean
defaults to false
| Adds a validation hook that appends -1
to your slug to avoid conflicts (experimental)
slugify
- Options to be passed to the slugify function
@default
{ lower: true, remove: /[*+~\/\\.()'"!?#\.,:@]/g }
checkbox
enable
- boolean
defaults to true
| Enable or disable the checkbox field
overrides
- CheckboxField
| Checkbox field overrides
Here is a more full example:
...SlugField(
{
name: 'secondSlug',
admin: {
position: 'sidebar',
},
},
{
useFields: ['nested.heading'],
},
{
enable: true,
overrides: {
name: 'secondEdit',
},
},
)
index
and unique
are set to true by default
import { CollectionConfig } from 'payload/types'
import { ComboField } from '@nouance/payload-better-fields-plugin'
const Examples: CollectionConfig = {
slug: 'examples',
admin: {
useAsTitle: 'fullName',
},
fields: [
{
type: 'row',
fields: [
{
name: 'firstName',
type: 'text',
},
{
name: 'lastName',
type: 'text',
},
],
},
...ComboField(['firstName', 'lastName'], { name: 'fullName' }),
],
}
The ComboField
accepts the following parameters
overrides
- TextField
required for name attribute
fieldToUse
- string[]
required
options
separator
- string
defaults to ' '
initial
- string
The starting string value before all fields are concatenated
callback
- (value: string) => string
You can apply a callback to modify each field value if you want to preprocess them
source | react-number-format NumericFormat
import { CollectionConfig } from 'payload/types'
import { NumberField } from '@nouance/payload-better-fields-plugin'
const Examples: CollectionConfig = {
slug: 'examples',
admin: {
useAsTitle: 'fullName',
},
fields: [
{
name: 'title',
type: 'text',
},
...NumberField(
{
name: 'price',
required: true,
},
{
prefix: '$ ',
suffix: ' %',
thousandSeparator: ',',
decimalScale: 2,
fixedDecimalScale: true,
},
),
],
}
The NumberField
accepts the following parameters
overrides
- NumberField
required for name attribute
format
- NumericFormatProps
required, accepts props for NumericFormat
callback
- you can override the internal callback on the value, the value
will be a string so you need to handle the conversion to an int or float yourself via parseFloat// example
callback: (value) => parseFloat(value) + 20,
source | react-number-format PatternFormat
import { CollectionConfig } from 'payload/types'
import { PatternField } from '@nouance/payload-better-fields-plugin'
const Examples: CollectionConfig = {
slug: 'examples',
admin: {
useAsTitle: 'fullName',
},
fields: [
{
name: 'title',
type: 'text',
},
...PatternField(
{
name: 'telephone',
type: 'text',
required: false,
admin: {
placeholder: '% 20',
},
},
{
format: '+1 (###) #### ###',
prefix: '% ',
allowEmptyFormatting: true,
mask: '_',
},
),
],
}
The PatternField
accepts the following parameters
overrides
- TextField
required for name attribute
format
- PatternFormatProps
required, accepts props for PatternFormat
format
required, input for the pattern to be applied
callback
- you can override the internal callback on the value, the value
will be a string so you need to handle the conversion to an int or float yourself via parseFloat
// example
callback: (value) => value + 'ID',
We recommend using a text field in Payload.
import { CollectionConfig } from 'payload/types'
import { RangeField } from '@nouance/payload-better-fields-plugin'
const Examples: CollectionConfig = {
slug: 'examples',
admin: {
useAsTitle: 'title',
},
fields: [
{
name: 'title',
type: 'text',
},
...RangeField(
{
name: 'groups',
},
{ min: 5, max: 200, step: 5 },
),
],
}
export default Examples
The RangeField
accepts the following parameters
overrides
- NumberField
required for name attribute
config
- required
min
- number
defaults to 1
max
- number
defaults to 100
step
- number
defaults to 1
showPreview
- boolean
defaults to false, shows a preview box of the underlying selected value, n/a
for null
markers
- NumberMarker[]
array of markers to be visually set, accepts an optional label
{ value: number, label?: string}[]
import { CollectionConfig } from 'payload/types'
import { ColourTextField } from '@nouance/payload-better-fields-plugin'
const Examples: CollectionConfig = {
slug: 'examples',
admin: {
useAsTitle: 'title',
},
fields: [
{
name: 'title',
type: 'text',
},
...ColourTextField({
name: 'colour',
}),
],
}
export default Examples
The ColourTextField
accepts the following parameters
overrides
- TextField
required for name attributesource | react-phone-number-input
import { CollectionConfig } from 'payload/types'
import { TelephoneField } from '@nouance/payload-better-fields-plugin'
const Examples: CollectionConfig = {
slug: 'examples',
admin: {
useAsTitle: 'title',
},
fields: [
{
name: 'title',
type: 'text',
},
...TelephoneField({
name: 'telephone',
admin: {
placeholder: '+1 2133 734 253',
},
}),
],
}
export default Examples
The TelephoneField
accepts the following parameters
overrides
- TextField
required for name
attribute
config
- Config
optional, allows for overriding attributes in the phone field
international
defaults to true
| Forces international formatting
defaultCountry
accepts a 2-letter country code eg. 'US'
| If defaultCountry is specified then the phone number can be input both in "international" format and "national" format
country
accepts a 2-letter country code eg. 'US'
| If country is specified then the phone number can only be input in "national" (not "international") format
initialValueFormat
If an initial value is passed, and initialValueFormat is "national", then the initial value is formatted in national format
withCountryCallingCode
If country is specified and international property is true then the phone number can only be input in "international" format for that country
countryCallingCodeEditable
smartCaret
When the user attempts to insert a digit somewhere in the middle of a phone number, the caret position is moved right before the next available digit skipping any punctuation in between
useNationalFormatForDefaultCountryValue
When defaultCountry is defined and the initial value corresponds to defaultCountry, then the value will be formatted as a national phone number by default
countrySelectProps
unicodeFlags
defaults to false
| Set to true
to render Unicode flag icons instead of SVG imagesimport { CollectionConfig } from 'payload/types'
import { AlertBoxField } from '@nouance/payload-better-fields-plugin'
const Examples: CollectionConfig = {
slug: 'examples',
admin: {
useAsTitle: 'title',
},
fields: [
{
name: 'title',
type: 'text',
},
...AlertBoxField(
{
name: 'alert',
},
{
type: 'info',
message: 'Please be aware that the title is required for the mobile app.',
},
),
],
}
export default Examples
The AlertBoxField
accepts the following parameters
overrides
- UIField
required for name
attribute
config
- Config
required
type
- a selection of info
| alert
| error
which come with different styles
message
- required string
className
- optional string to help you style individual alerts better
icon
- optional, default is enabled
enable
- boolean, turn off the icon
Element
- React component to override the provided icon
If you want to make this field appear conditionally, you should use the field's admin conditional config as provided by Payload.
import { CollectionConfig } from 'payload/types'
import { ColourPickerField } from '@nouance/payload-better-fields-plugin'
const Examples: CollectionConfig = {
slug: 'examples',
admin: {
useAsTitle: 'title',
},
fields: [
{
name: 'title',
type: 'text',
},
...ColourPickerField(
{
name: 'backgroundColour',
required: true,
},
{
type: 'hslA',
},
),
],
}
export default Examples
The ColourPickerField
accepts the following parameters
overrides
- TextField
required for name
attribute
config
- Config
required
type
- defaults to hex
, a selection of hex
| hexA
| rgb
| rgbA
| hsl
| hslA
expanded
- optional boolean, controls if the selector is visible by default
showPreview
- optional boolean
The goal of this field is to help with management of timezones.
import { CollectionConfig } from 'payload/types'
import { DateField } from '@nouance/payload-better-fields-plugin'
const Examples: CollectionConfig = {
slug: 'examples',
admin: {
useAsTitle: 'title',
},
fields: [
{
name: 'title',
type: 'text',
},
...DateField({
name: 'date',
admin: {
date: {
pickerAppearance: 'dayAndTime',
},
},
}),
],
}
export default Examples
The DateField
accepts the following parameters
overrides
- DateField
required for name
attribute
timezone
- Timezone
optional
enabled
- defaults to true
timezones
- optional list of timezones to customise available options
overrides
- SelectField
required for name
attribute
For development purposes, there is a full working example of how this plugin might be used in the dev directory of this repo.
git clone git@github.com:NouanceLabs/payload-better-fields-plugin.git \
cd payload-better-fields-plugin && yarn \
cd demo && yarn \
cp .env.example .env \
vim .env \ # add your payload details
yarn dev
Thanks to the following people for contributing code to this plugin
FAQs
A Payload plugin that aims to provide improved fields for the admin panel
The npm package @nouance/payload-better-fields-plugin receives a total of 762 weekly downloads. As such, @nouance/payload-better-fields-plugin popularity was classified as not popular.
We found that @nouance/payload-better-fields-plugin demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.