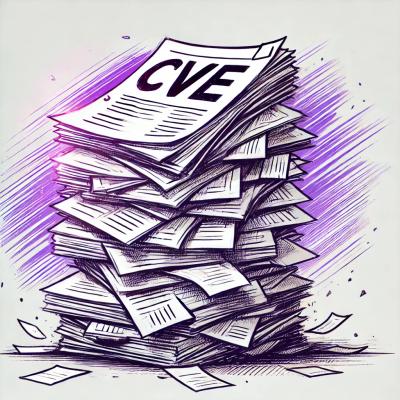
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
@polkadot/util-crypto
Advanced tools
@polkadot/util-crypto is a utility library for cryptographic functions, specifically designed for use with the Polkadot and Substrate ecosystems. It provides a wide range of cryptographic utilities including hashing, key generation, signing, and verification.
Hashing
This feature allows you to create a Blake2 hash of a given input. The code sample demonstrates how to hash the string 'hello world' using the Blake2 algorithm.
const { blake2AsHex } = require('@polkadot/util-crypto');
const data = 'hello world';
const hash = blake2AsHex(data);
console.log(hash);
Key Generation
This feature allows you to generate a mnemonic phrase for key generation. The code sample demonstrates how to generate a new mnemonic phrase.
const { mnemonicGenerate } = require('@polkadot/util-crypto');
const mnemonic = mnemonicGenerate();
console.log(mnemonic);
Signing
This feature allows you to verify a digital signature. The code sample demonstrates how to verify a signature for a given message and public key.
const { signatureVerify } = require('@polkadot/util-crypto');
const message = 'hello world';
const signature = '0x...'; // Replace with actual signature
const publicKey = '0x...'; // Replace with actual public key
const isValid = signatureVerify(message, signature, publicKey).isValid;
console.log(isValid);
Encryption
This feature allows you to encrypt and decrypt messages using the NaCl library. The code sample demonstrates how to encrypt and then decrypt a message.
const { naclEncrypt, naclDecrypt } = require('@polkadot/util-crypto');
const message = new Uint8Array([1, 2, 3, 4, 5]);
const secret = new Uint8Array(32); // Replace with actual secret key
const { encrypted, nonce } = naclEncrypt(message, secret);
const decrypted = naclDecrypt(encrypted, nonce, secret);
console.log(decrypted);
crypto-js is a widely-used library that provides standard and secure cryptographic algorithms for JavaScript. It offers functionalities like hashing, encryption, and decryption. Compared to @polkadot/util-crypto, crypto-js is more general-purpose and not specifically tailored for the Polkadot ecosystem.
tweetnacl is a cryptographic library that provides high-level API for encryption, decryption, signing, and verifying signatures. It is known for its simplicity and security. While tweetnacl offers similar functionalities, it does not provide the same level of integration with the Polkadot ecosystem as @polkadot/util-crypto.
Stanford JavaScript Crypto Library (sjcl) is a library for cryptography in JavaScript. It provides a variety of cryptographic primitives including hashing, encryption, and key generation. Like crypto-js, sjcl is a general-purpose library and does not offer specific utilities for the Polkadot ecosystem.
Various useful cyrpto utility functions that are used across all projects in the @polkadot namespace. It provides utility functions with additional safety checks, allowing not only for consistent coding, but also reducing the general boilerplate.
Installation -
yarn add @polkadot/util-crypto
Functions can be imported as follows:
import { mnemonicGenerate } from '@polkadot/util-crypto';
FAQs
A collection of useful crypto utilities for @polkadot
The npm package @polkadot/util-crypto receives a total of 90,728 weekly downloads. As such, @polkadot/util-crypto popularity was classified as popular.
We found that @polkadot/util-crypto demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.