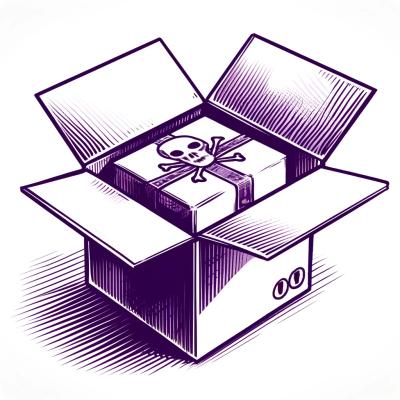
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
@proyecto26/animatable-component
Advanced tools
A WebComponent to use Web Animations API in a declarative way! 💅
<animatable/>
Web Component built with Stencil.js
to use Web Animations API in a declarative way.
You can animate any HTML element easily:
<animatable-component
autoplay
easing="ease-in-out"
duration="800"
delay="300"
animation="tada"
>
<h1>Hello World</h1>
</animatable-component>
To animate things you can use the createAnimatableComponent
utility.
import {
createAnimatableComponent
} from '@proyecto26/animatable-component';
const SendMessageButton = (props) =>(
<ion-fab-button {...props}>
<ion-icon name='send' />
</ion-fab-button>
);
const AnimatableSendMessageButton = createAnimatableComponent(SendMessageButton);
const keyFramesSendMessage: Keyframe[] = [
{
opacity: '0',
transform: 'rotate(0deg)'
},
{
opacity: '1',
transform: 'rotate(360deg)'
}
];
const optionsSendMessage: KeyframeAnimationOptions = {
duration: 500,
easing: 'ease-in-out'
};
...
render() {
return (
<AnimatableSendMessageButton
keyFrames={keyFramesSendMessage}
options={optionsSendMessage}
onFinish={() => alert('Eureka!')}
/>
)
}
...
Do you want to see this web component in action? Visit https://codepen.io/jdnichollsc/full/rNNYBbe yay! 🎉
Property | Attribute | Description | Type | Default |
---|---|---|---|---|
animateId | animate-id | A DOMString with which to reference the animation. | string | undefined |
animation | animation | Name of the animation to get the keyFrames. | "bounce" | "flash" | "jello" | "pulse" | "rotate" | "shake" | "swing" | "rubberBand" | "tada" | "wobble" | "heartBeat" | "bounceIn" | "bounceInUp" | "bounceInDown" | "bounceInRight" | "bounceInLeft" | "bounceOut" | "bounceOutUp" | "bounceOutDown" | "bounceOutRight" | "bounceOutLeft" | "fadeIn" | "fadeInUp" | "fadeInUpBig" | "fadeInDown" | "fadeInDownBig" | "fadeInRight" | "fadeInRightBig" | "fadeInLeft" | "fadeInLeftBig" | "fadeOut" | "fadeOutUp" | "fadeOutUpBig" | "fadeOutDown" | "fadeOutDownBig" | "fadeOutRight" | "fadeOutRightBig" | "fadeOutLeft" | "fadeOutLeftBig" | "flip" | "flipInX" | "flipInY" | "flipOutX" | "flipOutY" | "lightSpeedIn" | "lightSpeedOut" | "rotateIn" | "rotateInClockwise" | "rotateInDownLeft" | "rotateInDownRight" | "rotateInUpLeft" | "rotateInUpRight" | "rotateOut" | "rotateOutClockwise" | "rotateOutDownLeft" | "rotateOutDownRight" | "rotateOutUpLeft" | "rotateOutUpRight" | "slideInUp" | "slideInDown" | "slideInLeft" | "slideInRight" | "slideOutUp" | "slideOutDown" | "slideOutLeft" | "slideOutRight" | "zoomIn" | "zoomInUp" | "zoomInDown" | "zoomInLeft" | "zoomInRight" | "zoomOut" | "zoomOutUp" | "zoomOutDown" | "zoomOutLeft" | "zoomOutRight" | "hinge" | "jackInTheBox" | "rollIn" | "rollOut" | undefined |
autoPlay | autoplay | Start the animation when the component is mounted. | boolean | true |
composite | composite | Determines how values are combined between this animation and other, separate animations that do not specify their own specific composite operation. Defaults to replace . | "accumulate" | "add" | "replace" | undefined |
currentTime | current-time | Sets the current time value of the animation in milliseconds, whether running or paused. | number | undefined |
delay | delay | The number of milliseconds to delay the start of the animation. Defaults to 0. | number | undefined |
direction | direction | Direction of the animation. | "alternate" | "alternate-reverse" | "normal" | "reverse" | undefined |
duration | duration | The number of milliseconds each iteration of the animation takes to complete. Defaults to 0. | number | undefined |
easing | easing | The rate of the animation's change over time. | custom function | "linear" | "ease" | "ease-in" | "ease-out" | "ease-in-out" | "ease-in-cubic" | "ease-out-cubic" | "ease-in-out-cubic" | "ease-in-circ" | "ease-out-circ" | "ease-in-out-circ" | "ease-in-expo" | "ease-out-expo" | "ease-in-out-expo" | "ease-in-quad" | "ease-out-quad" | "ease-in-out-quad" | "ease-in-quart" | "ease-out-quart" | "ease-in-out-quart" | "ease-in-quint" | "ease-out-quint" | "ease-in-out-quint" | "ease-in-sine" | "ease-out-sine" | "ease-in-out-sine" | "ease-in-back" | "ease-out-back" | "ease-in-out-back" | undefined |
endDelay | end-delay | The number of milliseconds to delay after the end of an animation. | number | undefined |
fill | fill | Dictates whether the animation's effects should be reflected by the element(s) prior to playing ("backwards"), retained after the animation has completed playing ("forwards"), or both. Defaults to "none". | "auto" | "backwards" | "both" | "forwards" | "none" | undefined |
iterationComposite | iteration-composite | Determines how values build from iteration to iteration in this animation. | "accumulate" | "replace" | undefined |
iterationStart | iteration-start | Describes at what point in the iteration the animation should start. | number | undefined |
iterations | iterations | The number of times the animation should repeat. Defaults to 1 , and can also take a value of Infinity to make it repeat for as long as the element exists. | number | undefined |
keyFrames | -- | Keyframes of the animation. | Keyframe[] | undefined |
keyFramesData | key-frames-data | Keyframes of the animation in string format. | string | undefined |
options | -- | Default options of the animation. | KeyframeAnimationOptions | undefined |
optionsData | options-data | Default options of the animation in string format. | string | undefined |
playbackRate | playback-rate | Sets the playback rate of the animation. | number | undefined |
startTime | start-time | Sets the scheduled time when an animation's playback should begin. | number | undefined |
Event | Description | Type |
---|---|---|
start | This event is sent when the animation is going to play. | CustomEvent<HTMLElement> |
cancel | This event is sent when the animation is cancelled. | CustomEvent<HTMLElement> |
finish | This event is sent when the animation finishes playing. | CustomEvent<HTMLElement> |
cancel() => Promise<void>
Clears all KeyframeEffects
caused by this animation and aborts its playback.
Type: Promise<void>
finish() => Promise<void>
Sets the current playback time to the end of the animation corresponding to the current playback direction.
Type: Promise<void>
getCurrentTime() => Promise<number>
Returns the current time value of the animation in milliseconds, whether running or paused.
Type: Promise<number>
getPending() => Promise<boolean>
Indicates whether the animation is currently waiting for an asynchronous operation such as initiating playback or pausing a running animation.
Type: Promise<boolean>
getPlayState() => Promise<AnimationPlayState>
Returns an enumerated value describing the playback state of an animation.
Type: Promise<AnimationPlayState>
getPlaybackRate() => Promise<number>
Returns the playback rate of the animation.
Type: Promise<number>
getStartTime() => Promise<number>
Returns the scheduled time when an animation's playback should begin.
Type: Promise<number>
pause() => Promise<void>
Suspends playback of the animation.
Type: Promise<void>
play() => Promise<void>
Starts or resumes playing of an animation.
Type: Promise<void>
reverse() => Promise<void>
Reverses the playback direction, meaning the animation ends at its beginning.
Type: Promise<void>
<script src='https://unpkg.com/animatable-component@0.1.1/dist/animatable-component.js'></script>
in the head of your index.htmlnpm install @proyecto26/animatable-component --save
<script src='node_modules/@proyecto26/animatable-component/dist/animatable-component.js'></script>
in the head of your index.htmlnpm install @proyecto26/animatable-component --save
import @proyecto26/animatable-component;
Using animatable-component
component within an Angular project:
Including the CUSTOM_ELEMENTS_SCHEMA
in the module allows the use of Web Components in the HTML files. Here is an example of adding it to AppModule
:
import { BrowserModule } from '@angular/platform-browser';
import { CUSTOM_ELEMENTS_SCHEMA, NgModule } from '@angular/core';
import { AppComponent } from './app.component';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule],
bootstrap: [AppComponent],
schemas: [CUSTOM_ELEMENTS_SCHEMA]
})
export class AppModule {}
The CUSTOM_ELEMENTS_SCHEMA
needs to be included in any module that uses Animatable.
Animatable component includes a function used to load itself in the application window object. That function is called defineCustomElements()
and needs to be executed once during the bootstrapping of your application. One convenient place to add it is in the main.ts
file as follows:
import { enableProdMode } from '@angular/core';
import { platformBrowserDynamic } from '@angular/platform-browser-dynamic';
import { defineCustomElements as defineAnimatable } from '@proyecto26/animatable-component/loader';
import { AppModule } from './app/app.module';
import { environment } from './environments/environment';
if (environment.production) {
enableProdMode();
}
platformBrowserDynamic().bootstrapModule(AppModule)
.catch(err => console.log(err));
defineAnimatable(window);
import React from 'react'
import ReactDOM from 'react-dom'
import { defineCustomElements as defineAnimatable } from '@proyecto26/animatable-component/loader'
import App from './App';
ReactDOM.render(<App />, document.getElementById('root'));
defineAnimatable(window);
In order to use the animatable-component
Web Component inside of a Vue application, it should be modified to define the custom elements and to inform the Vue compiler which elements to ignore during compilation. This can all be done within the main.js
file as follows:
import Vue from 'vue';
import { defineCustomElements as defineAnimatable } from '@proyecto26/animatable-component/loader'
import App from './App.vue';
Vue.config.productionTip = false;
Vue.config.ignoredElements = [/animatable-\w*/];
// Bind the custom element to the window object
defineAnimatable(window);
new Vue({
render: h => h(App)
}).$mount('#app');
I believe in Unicorns 🦄 Support me, if you do too.
To report a security vulnerability, please use the Tidelift security contact. Tidelift will coordinate the fix and disclosure.
Made with ❤️
FAQs
Animate once, use Everywhere! 💫
The npm package @proyecto26/animatable-component receives a total of 785 weekly downloads. As such, @proyecto26/animatable-component popularity was classified as not popular.
We found that @proyecto26/animatable-component demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.