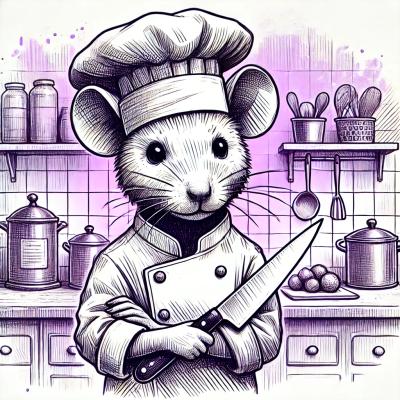
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@pulumi/gcp
Advanced tools
A Pulumi package for creating and managing Google Cloud Platform resources.
@pulumi/gcp is a Pulumi package that allows developers to create, deploy, and manage Google Cloud Platform (GCP) resources using Pulumi's infrastructure as code approach. It provides a wide range of functionalities to manage various GCP services such as Compute Engine, Cloud Storage, BigQuery, and more.
Compute Engine
This feature allows you to create and manage Google Compute Engine instances. The code sample demonstrates how to create a new Compute Engine instance with specific configurations.
const gcp = require('@pulumi/gcp');
const compute = new gcp.compute.Instance('my-instance', {
machineType: 'f1-micro',
zone: 'us-central1-a',
bootDisk: {
initializeParams: {
image: 'debian-cloud/debian-9'
}
},
networkInterfaces: [{
network: 'default',
accessConfigs: [{}]
}]
});
Cloud Storage
This feature allows you to create and manage Google Cloud Storage buckets. The code sample demonstrates how to create a new Cloud Storage bucket with specific configurations.
const gcp = require('@pulumi/gcp');
const bucket = new gcp.storage.Bucket('my-bucket', {
location: 'US',
forceDestroy: true
});
BigQuery
This feature allows you to create and manage Google BigQuery datasets. The code sample demonstrates how to create a new BigQuery dataset with specific configurations.
const gcp = require('@pulumi/gcp');
const dataset = new gcp.bigquery.Dataset('my-dataset', {
datasetId: 'my_dataset',
friendlyName: 'My Dataset',
description: 'A sample dataset'
});
Cloud Functions
This feature allows you to create and manage Google Cloud Functions. The code sample demonstrates how to create a new Cloud Function with specific configurations.
const gcp = require('@pulumi/gcp');
const function = new gcp.cloudfunctions.Function('my-function', {
runtime: 'nodejs10',
entryPoint: 'helloWorld',
sourceArchiveBucket: bucket.name,
sourceArchiveObject: bucketObject.name,
triggerHttp: true
});
@pulumi/aws is a Pulumi package that allows developers to create, deploy, and manage Amazon Web Services (AWS) resources using Pulumi's infrastructure as code approach. It provides similar functionalities to @pulumi/gcp but for AWS services.
@pulumi/azure is a Pulumi package that allows developers to create, deploy, and manage Microsoft Azure resources using Pulumi's infrastructure as code approach. It provides similar functionalities to @pulumi/gcp but for Azure services.
Terraform is an open-source infrastructure as code software tool created by HashiCorp. It allows users to define and provision data center infrastructure using a high-level configuration language. Terraform supports multiple cloud providers, including GCP, AWS, and Azure, making it a versatile alternative to @pulumi/gcp.
FAQs
A Pulumi package for creating and managing Google Cloud Platform resources.
The npm package @pulumi/gcp receives a total of 311,056 weekly downloads. As such, @pulumi/gcp popularity was classified as popular.
We found that @pulumi/gcp demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.