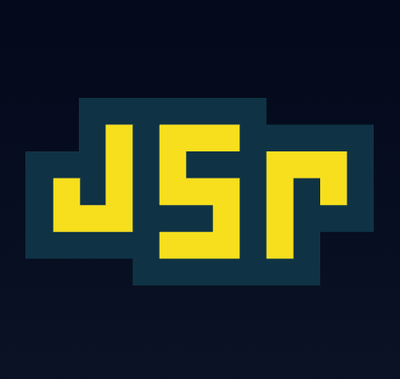
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@react-stately/collections
Advanced tools
@react-stately/collections is a library that provides utilities for managing collections of data in React applications. It offers a set of hooks and components to handle common collection operations such as iteration, filtering, and sorting in a declarative way.
Iterating over collections
This feature allows you to iterate over a collection of items and render them in a list. The `useCollection` hook is used to manage the collection state.
import { useCollection } from '@react-stately/collections';
const items = [
{ id: 1, name: 'Item 1' },
{ id: 2, name: 'Item 2' },
{ id: 3, name: 'Item 3' }
];
function MyComponent() {
const collection = useCollection({ items });
return (
<ul>
{collection.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
}
Filtering collections
This feature allows you to filter a collection of items based on a condition. The `filter` method is used to create a new array with items that match the condition.
import { useCollection } from '@react-stately/collections';
const items = [
{ id: 1, name: 'Item 1' },
{ id: 2, name: 'Item 2' },
{ id: 3, name: 'Item 3' }
];
function MyComponent() {
const collection = useCollection({ items });
const filteredItems = collection.filter(item => item.name.includes('1'));
return (
<ul>
{filteredItems.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
}
Sorting collections
This feature allows you to sort a collection of items based on a comparison function. The `sort` method is used to order the items in the collection.
import { useCollection } from '@react-stately/collections';
const items = [
{ id: 3, name: 'Item 3' },
{ id: 1, name: 'Item 1' },
{ id: 2, name: 'Item 2' }
];
function MyComponent() {
const collection = useCollection({ items });
const sortedItems = collection.sort((a, b) => a.name.localeCompare(b.name));
return (
<ul>
{sortedItems.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
}
react-table is a lightweight, fast, and extendable data grid built for React. It provides hooks for managing table state, including sorting, filtering, and pagination. Compared to @react-stately/collections, react-table is more focused on tabular data and provides more advanced features for table management.
react-virtualized is a library for efficiently rendering large lists and tabular data in React. It provides components for virtualizing rows and columns, which can significantly improve performance for large datasets. While @react-stately/collections focuses on collection management, react-virtualized is specialized in optimizing rendering performance.
react-window is a lightweight library for rendering large lists and tabular data in React. It is similar to react-virtualized but with a smaller API surface and better performance. Like react-virtualized, it focuses on rendering performance, whereas @react-stately/collections provides utilities for managing collections.
This package is part of react-spectrum. See the repo for more details.
FAQs
Spectrum UI components in React
The npm package @react-stately/collections receives a total of 1,231,340 weekly downloads. As such, @react-stately/collections popularity was classified as popular.
We found that @react-stately/collections demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.