Rematch Loading
Adds automated loading indicators for effects to Rematch. Inspired by dva-loading.
Example
See an example below using a loading indicator within a button.
import React from 'react'
import { connect } from 'react-redux'
import AwesomeLoadingButton from './components/KindaCoolLoadingButton'
const LoginButton = (props) => (
<AwesomeLoadingButton onClick={props.submit} loading={props.loading}>
Login
</AwesomeLoadingButton>
)
const mapState = (state) => ({
loading: state.loading.effects.login.submit,
loading: state.loading.models.login,
})
const mapDispatch = (dispatch) => ({
submit: () => dispatch.login.submit()
})
export default connect(mapState, mapDispatch)(LoginButton)
Demo
See a demo
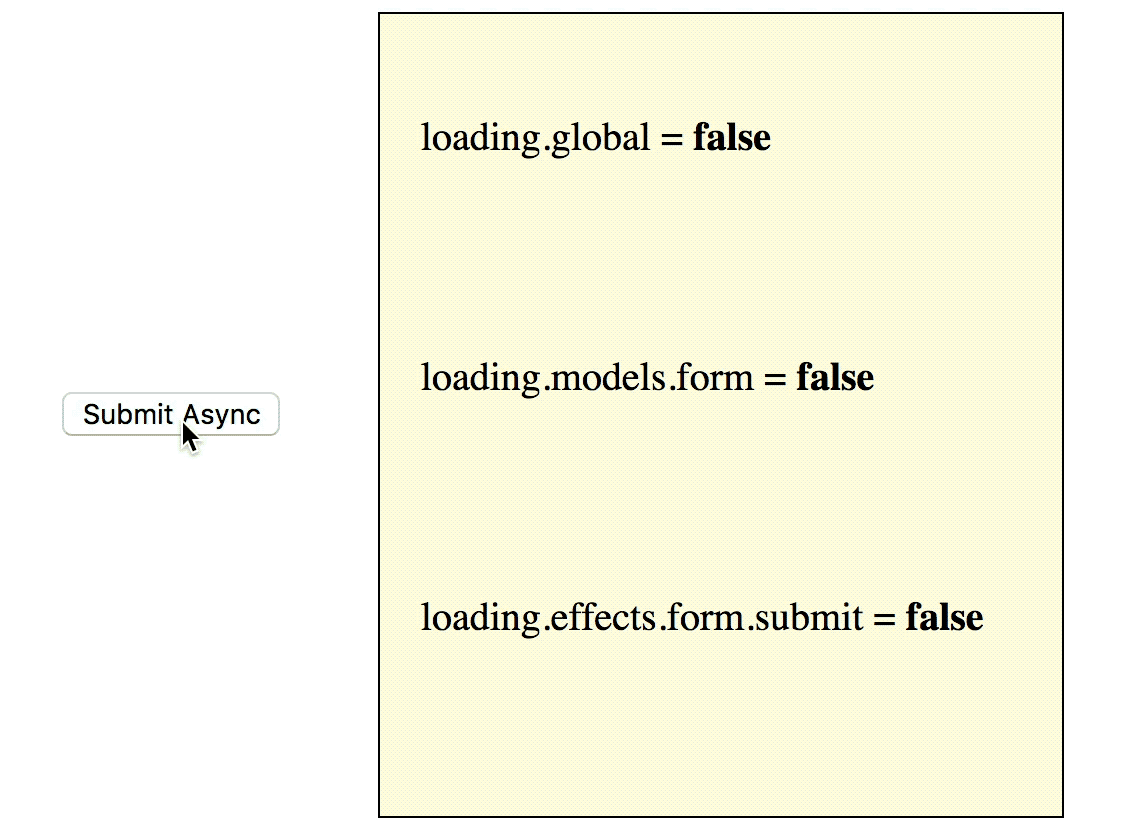
Setup
Install @rematch/loading
as a dependency.
npm install @rematch/loading
Configure loading.
import { init } from '@rematch/core'
import createLoadingPlugin from '@rematch/loading'
const options = {}
const loading = createLoadingPlugin(options)
init({
plugins: [loading]
})
Options
asNumber
{ asNumber: true }
The loading state values are a "counter", returns a number (eg. `store.getState().loading.global === 5`).
Defaults to `false`, returns a boolean (eg. `store.getState().loading.global === true`)
### name
```js
{ name: 'load' }
In which case, loading can be accessed from state.load.global
.
Defaults to the name of loading
(eg. state.loading.global
).
whitelist
A shortlist of actions. Named with "modelName" / "actionName".
{ whitelist: ['count/addOne'] })
blacklist
A shortlist of actions to exclude from loading indicators.
{ blacklist: ['count/addOne'] })