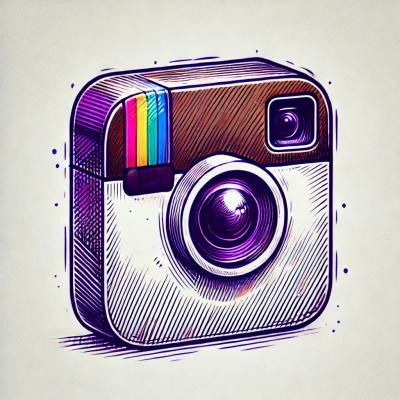
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
@secux/app-ada
Advanced tools
@secux/app-ada
SecuX Hardware Wallet ADA API
import { SecuxADA, AddressType } from "@secux/app-ada";
First, create instance of ITransport
Get shelley address
const path = "m/1852'/1815'/0'";
const address = await device.getAddress(path, AddressType.BASE);
/*
// transfer data to hardware wallet by custom transport layer
const data = SecuxADA.prepareAddress(path);
const response = await device.Exchange(data);
const address = SecuxADA.resolveAddress(response, AddressType.BASE);
*/
const path = "m/1852'/1815'/0'";
const address = await device.getAddress(path, AddressType.REWARD);
Sign transaction
const inputs = [
{
path: "m/1852'/1815'/0'",
txId: "75c7d745c5212a11a0bfc2719c35bcc2f57fda88d7afb2eb3c5f2b02c3e99ccb",
index: 1,
amount: 12663894,
// for custom transport layer, each utxo needs xpublickey.
// xpublickey: "c232950d7c27b78542795ce4cad053e8dfaab7679ba5477563be5c60c1a4d0613fc81fd9bb8f30822c1252c29cc6af147831da44fb86acad6c04fcc95700b92b"
},
{
path: "m/1852'/1815'/0'",
txId: "6552b8f8b8b282542b07d6187fe80daa5b7a60461c97231f45c06fd97f8a3385",
index: 1,
amount: 2330624,
// for custom transport layer, each utxo needs xpublickey.
// xpublickey: "c232950d7c27b78542795ce4cad053e8dfaab7679ba5477563be5c60c1a4d0613fc81fd9bb8f30822c1252c29cc6af147831da44fb86acad6c04fcc95700b92b"
},
];
const output = {
// daedalus or shelley address is accepted.
address: "DdzFFzCqrhsjZHKn8Y9Txr4B9PaEtYcYp8TGa4gQTfJfjvuNLqvB8hPG35WRgK4FjcSYhgK7b2H24jLMeqmPoS3YhJq6bjStsx4BZVnn",
amount: 13000000
};
const { raw_tx } = await device.sign(inputs, output, {
changeAddress: "addr1qyk54vyyc856ngxermdzqhxnlk376ykkupru8rxcyryvg4kxs4un3x4r4rq422kwrtvc8p2a20dzhyr5v0n9lhwy2u6sfjujuz",
});
/*
// transfer data to hardware wallet by custom transport layer.
const { commandData, serialized } = SecuxADA.prepareSign(inputs, output, {
changeAddress: "addr1qyk54vyyc856ngxermdzqhxnlk376ykkupru8rxcyryvg4kxs4un3x4r4rq422kwrtvc8p2a20dzhyr5v0n9lhwy2u6sfjujuz",
});
const response = await device.Exchange(commandData);
const raw_tx = SecuxADA.resloveTransaction(response, serialized);
*/
const input = {
path: "m/1852'/1815'/0'",
utxo: [
{
txId: "75c7d745c5212a11a0bfc2719c35bcc2f57fda88d7afb2eb3c5f2b02c3e99ccb",
index: 1,
amount: 12663894,
}
],
changeAddress: "addr1qyk54vyyc856ngxermdzqhxnlk376ykkupru8rxcyryvg4kxs4un3x4r4rq422kwrtvc8p2a20dzhyr5v0n9lhwy2u6sfjujuz",
// for custom transport layer, each utxo needs xpublickey.
// xpublickey: "c232950d7c27b78542795ce4cad053e8dfaab7679ba5477563be5c60c1a4d0613fc81fd9bb8f30822c1252c29cc6af147831da44fb86acad6c04fcc95700b92b"
};
// pool id (support bech32 encoded)
const pool = "ea595c6f726db925b6832af51795fd8a46e700874c735d204f7c5841";
const { raw_tx } = await device.sign(
input,
pool,
{
// An account needs to have a stake pool registration certificate
// before it can participate in stake delegation between stake pools.
needRegistration: true
}
);
/*
// transfer data to hardware wallet by custom transport layer.
const { commandData, serialized } = SecuxADA.prepareStake(
input,
pool,
{
needRegistration: true
}
);
const response = await device.Exchange(commandData);
const raw_tx = SecuxADA.resolveTransaction(response, serialized);
*/
const withdrawAmount = 150000;
const { raw_tx } = await device.sign(input, withdrawAmount);
/*
// transfer data to hardware wallet by custom transport layer.
const { commandData, serialized } = SecuxADA.prepareStake(input, withdrawAmount);
const response = await device.Exchange(commandData);
const raw_tx = SecuxADA.resolveTransaction(response, serialized);
*/
const { raw_tx } = await device.sign(
input,
{
// With de-registration operation, the balance of reward address must be 0.
withdrawAmount
}
);
/*
// transfer data to hardware wallet by custom transport layer.
const { commandData, serialized } = SecuxADA.prepareUnstake(input, { withdrawAmount });
const response = await device.Exchange(commandData);
const raw_tx = SecuxADA.resolveTransaction(response, serialized);
*/
ADA package for SecuX device
Kind: global class
string
communicationData
string
communicationData
string
prepared
Array.<string>
string
prepared
prepared
prepared
string
Convert bip32-publickey to ADA address.
Returns: string
- address
Param | Type | Description |
---|---|---|
xpublickey | string | Buffer | ada bip32-publickey |
type | AddressType | |
[option] | AddressOption |
communicationData
Prepare data for address generation.
Returns: communicationData
- data for sending to device
Param | Type | Description |
---|---|---|
pathWith3Depth | string | m/1852'/1815'/... |
string
Resolve address from response data.
Returns: string
- address
Param | Type | Description |
---|---|---|
response | communicationData | data from device |
type | AddressType | |
[option] | AddressOption |
communicationData
Prepare data for bip32-publickey.
Returns: communicationData
- data for sending to device
Param | Type | Description |
---|---|---|
pathWith3Depth | string | m/1852'/1815'/... |
string
Resolve bip32-publickey from response data.
Returns: string
- bip32-publickey (hex string)
Param | Type | Description |
---|---|---|
response | communicationData | data from device |
prepared
Prepare data for signing.
Param | Type |
---|---|
inputs | Array.<txInput> |
output | txOutput |
[option] | signOption |
Array.<string>
Reslove signatures from response data.
Returns: Array.<string>
- signature array of hex string
Param | Type | Description |
---|---|---|
response | communicationData | data from device |
string
Resolve transaction for broadcasting.
Returns: string
- signed transaction (base64 encoded)
Param | Type | Description |
---|---|---|
response | communicationData | data from device |
serialized | communicationData |
prepared
Prepare data for signing.
Param | Type | Description |
---|---|---|
input | stakeInput | |
pool | string | pool hash or id |
[option] | stakeOption |
prepared
Prepare data for signing.
Param | Type |
---|---|
input | stakeInput |
[option] | unstakeOption |
prepared
Prepare data for signing.
Param | Type | Description |
---|---|---|
input | stakeInput | |
amount | number | string | rewards |
[option] | withdrawOption |
enum
Properties
Name | Type | Description |
---|---|---|
BASE | number | 0 |
ENTERPRISE | number | 1 |
POINTER | number | 2 |
REWARD | number | 3 |
BOOTSTRAPv1 | number | 4 |
BOOTSTRAPv2 | number | 5 |
object
Properties
Name | Type |
---|---|
slot | number |
txIndex | number |
certIndex | number |
object
Properties
Name | Type | Description |
---|---|---|
[addressIndex] | number | account index |
[stakeIndex] | number | stake key index |
[pointer] | PointerOption | option for Pointer address |
object
Properties
Name | Type | Description |
---|---|---|
path | string | 3-depth path of CIP-1852 |
xpublickey | string | Buffer | ED25519 publickey from path |
txId | string | referenced transaction hash |
index | number | referenced transaction output index |
amount | number | string | referenced transaction output amount |
[addressIndex] | number | default: 0 |
[stakeIndex] | number | default: 0 |
object
Properties
Name | Type | Description |
---|---|---|
address | string | receiver's address |
amount | number | string | amount of payment |
object
Properties
Name | Type | Description |
---|---|---|
[changeAddress] | string | default: sender's address |
[fee] | number | string | |
[TimeToLive] | number |
object
Properties
Name | Type | Description |
---|---|---|
[stakeIndex] | number | default: 0 |
[needRegistration] | boolean | include registration or not |
[fee] | number | string | |
[TimeToLive] | number |
object
Properties
Name | Type | Description |
---|---|---|
[stakeIndex] | number | default: 0 |
[fee] | number | string | |
[TimeToLive] | number |
object
Properties
Name | Type | Description |
---|---|---|
[stakeIndex] | number | default: 0 |
[withdrawAmount] | boolean | withdraw and de-registration |
[fee] | number | string | |
[TimeToLive] | number |
object
Properties
Name | Type | Description |
---|---|---|
txId | string | referenced transaction hash |
index | number | referenced transaction output index |
amount | number | string | referenced transaction output amount |
[addressIndex] | number | default: 0 |
object
Properties
Name | Type | Description |
---|---|---|
path | string | 3-depth path of CIP-1852 |
utxo | Array.<utxo> | |
changeAddress | string | owner's account |
xpublickey | string | Buffer | cardano bip32-publickey |
[stakeIndex] | number | default: 0 |
object
Properties
Name | Type | Description |
---|---|---|
commandData | communicationData | data for sending to device |
serialized | communicationData |
© 2018-21 SecuX Technology Inc.
authors:
andersonwu@secuxtech.com
FAQs
SecuX Hardware Wallet ADA API
The npm package @secux/app-ada receives a total of 0 weekly downloads. As such, @secux/app-ada popularity was classified as not popular.
We found that @secux/app-ada demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.