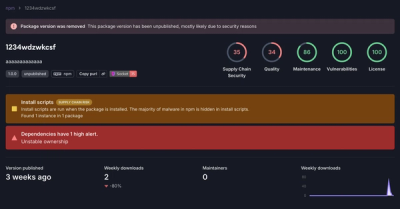
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
@sentry/tracing
Advanced tools
The @sentry/tracing package provides performance monitoring and distributed tracing for applications. It allows developers to track the performance of their applications, understand latency issues, and monitor the flow of requests through various services in a distributed system.
Automatic Instrumentation
Automatically instruments the application to collect performance data. This includes capturing data for outgoing HTTP requests when using the Sentry.Integrations.Http integration.
const Sentry = require('@sentry/node');
const Tracing = require('@sentry/tracing');
Sentry.init({
dsn: 'YOUR_DSN',
tracesSampleRate: 1.0,
integrations: [new Sentry.Integrations.Http({ tracing: true })],
});
Manual Instrumentation
Allows developers to manually create transactions to trace specific operations or workflows within their application.
const transaction = Sentry.startTransaction({
op: 'test',
name: 'My Transaction',
});
// ... your code here ...
transaction.finish();
Performance Monitoring
Integrates with web frameworks like Express to provide performance monitoring for web applications. This includes automatic tracing of requests and responses.
const express = require('express');
const app = express();
const Sentry = require('@sentry/node');
const Tracing = require('@sentry/tracing');
Sentry.init({
dsn: 'YOUR_DSN',
integrations: [
new Sentry.Integrations.Http({ tracing: true }),
new Tracing.Integrations.Express({ app }),
],
tracesSampleRate: 1.0,
});
app.use(Sentry.Handlers.requestHandler());
app.use(Sentry.Handlers.tracingHandler());
// All controllers should live here
app.use(Sentry.Handlers.errorHandler());
app.listen(3000);
Distributed Tracing
Supports distributed tracing by allowing services to pass trace context in HTTP headers, enabling the tracking of requests across service boundaries.
const transaction = Sentry.startTransaction({
op: 'http',
name: 'My HTTP Request',
});
fetch('https://example.com', {
method: 'GET',
headers: {
'Sentry-Trace': transaction.toTraceparent(),
},
})
.then(response => {
// ... process the response ...
})
.finally(() => {
transaction.finish();
});
Zipkin is a distributed tracing system that helps gather timing data needed to troubleshoot latency problems in service architectures. It has features similar to @sentry/tracing but focuses more on the tracing aspect and less on error tracking and performance monitoring.
Jaeger, inspired by Dapper and OpenZipkin, is a distributed tracing system released as open source by Uber Technologies. Like @sentry/tracing, it provides monitoring and troubleshooting of microservices-based distributed systems, including distributed context propagation and transaction monitoring.
Datadog's APM tracing client for Node.js, dd-trace, provides distributed tracing and performance monitoring. It is similar to @sentry/tracing but is tailored to integrate with Datadog's suite of observability tools.
New Relic's APM tool offers application performance monitoring, including transaction tracing. It provides a full-stack observability solution, which includes features for monitoring application health, user experience, and network performance, in addition to distributed tracing.
⚠️ Deprecation Notice: From SDK versions >=
7.47.0
onwards, the@sentry/tracing
package is officially deprecated. This package will be removed in a future major release. More details can be found in the migration docs.
This package was discontinued with version 8.0.0 of the Sentry JavaScript SDKs. Instead, exports from this package are
exported directly from the main SDK packages like @sentry/react
or @sentry/node
. For more information have a look at
the Migration Guide.
This package contains extensions to the @sentry/hub
to enable Sentry AM related functionality. It also provides
integrations for Browser and Node that provide a good experience out of the box.
The tracing integration for JavaScript SDKs has moved from @sentry/apm
to
@sentry/tracing
. While the two packages are similar, some imports and
APIs have changed slightly.
The old package @sentry/apm
is deprecated in favor of @sentry/tracing
. Future support for @sentry/apm
is limited
to bug fixes only.
If you were using the Browser CDN bundle, switch from the old bundle.apm.min.js
to the new tracing bundle:
<script
src="https://browser.sentry-cdn.com/{{ packages.version('sentry.javascript.browser') }}/bundle.tracing.min.js"
integrity="sha384-{{ packages.checksum('sentry.javascript.browser', 'bundle.tracing.min.js', 'sha384-base64') }}"
crossorigin="anonymous"
></script>
And then update Sentry.init
:
Sentry.init({
- integrations: [new Sentry.Integrations.Tracing()]
+ integrations: [new Sentry.Integrations.BrowserTracing()]
});
If you were using automatic instrumentation, update the import statement and update Sentry.init
to use the new
BrowserTracing
integration:
import * as Sentry from "@sentry/browser";
-import { Integrations } from "@sentry/apm";
+import { Integrations } from "@sentry/tracing";
Sentry.init({
integrations: [
- new Integrations.Tracing(),
+ new Integrations.BrowserTracing(),
]
});
If you were using the beforeNavigate
option from the Tracing
integration, the API in BrowserTracing
has changed
slightly. Instead of passing in a location and returning a string representing transaction name, beforeNavigate
now
accepts a transaction context and is expected to return a transaction context. You can now add extra tags or change the
op
based on different parameters. If you want to access the location like before, you can get it from
window.location
.
For example, if you had a function like so that computed a custom transaction name:
import * as Sentry from '@sentry/browser';
import { Integrations } from '@sentry/apm';
Sentry.init({
integrations: [
new Integrations.Tracing({
beforeNavigate: location => {
return getTransactionName(location);
},
}),
],
});
You would now leverage the context to do the same thing:
import * as Sentry from '@sentry/browser';
import { Integrations } from '@sentry/tracing';
Sentry.init({
integrations: [
new Integrations.BrowserTracing({
beforeNavigate: context => {
return {
...context,
// Can even look at context tags or other data to adjust
// transaction name
name: getTransactionName(window.location),
};
},
}),
],
});
For the full diff:
import * as Sentry from "@sentry/browser";
-import { Integrations } from "@sentry/apm";
+import { Integrations } from "@sentry/tracing";
Sentry.init({
integrations: [
- new Integrations.Tracing({
- beforeNavigate: (location) => {
- return getTransactionName(location)
+ new Integrations.BrowserTracing({
+ beforeNavigate: (ctx) => {
+ return {
+ ...ctx,
+ name: getTransactionName(ctx.name, window.location)
+ }
}
}),
]
});
If you were using the Express integration for automatic instrumentation, the only necessary change is to update the import statement:
import * as Sentry from "@sentry/node";
-import { Integrations } from "@sentry/apm";
+import { Integrations } from "@sentry/tracing";
Sentry.init({
integrations: [
new Integrations.Express(),
]
});
FAQs
Sentry Performance Monitoring Package
The npm package @sentry/tracing receives a total of 1,854,837 weekly downloads. As such, @sentry/tracing popularity was classified as popular.
We found that @sentry/tracing demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 11 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.