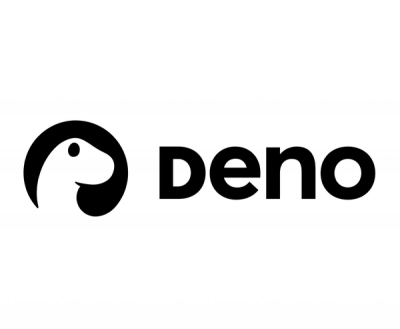
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@storybook/addon-storysource
Advanced tools
View a story’s source code to see how it works and paste into your app
@storybook/addon-storysource is an addon for Storybook that allows you to view the source code of your stories directly within the Storybook interface. This can be particularly useful for documentation purposes and for developers who want to see how a particular story is implemented.
View Story Source Code
This feature allows you to view the source code of your stories directly within the Storybook interface. By using the `withStorySource` decorator, you can add the source code of the story to the Storybook panel.
import { withStorySource } from '@storybook/addon-storysource';
export default {
title: 'Button',
decorators: [withStorySource],
};
export const Primary = () => <button>Primary</button>;
Primary.story = {
parameters: {
storySource: {
source: '<button>Primary</button>',
},
},
};
Automatic Source Code Extraction
This feature automatically extracts the source code of your stories and displays it in the Storybook panel. By setting `injectStoryParameters` to true, you can enable automatic source code extraction for all stories.
import { addParameters } from '@storybook/react';
import { withStorySource } from '@storybook/addon-storysource';
addParameters({
storySource: {
loaderOptions: {
injectStoryParameters: true,
},
},
});
export default {
title: 'Button',
decorators: [withStorySource],
};
export const Secondary = () => <button>Secondary</button>;
@storybook/addon-docs provides a comprehensive documentation addon for Storybook. It allows you to write and view documentation alongside your stories, including the ability to view source code, props tables, and more. Compared to @storybook/addon-storysource, @storybook/addon-docs offers a more extensive set of documentation features.
react-docgen is a CLI and library for extracting information from React component files. It can be used to generate documentation for your components, including prop types and default values. While it does not integrate directly with Storybook, it can be used in conjunction with other tools to provide similar functionality to @storybook/addon-storysource.
This addon is used to show stories source in the addon panel.
First, install the addon
yarn add @storybook/addon-storysource --dev
You can add configuration for this addon by using a preset or by using the addon config with webpack
Add the following to your .storybook/main.js
exports:
export default {
addons: ['@storybook/addon-storysource'],
};
You can pass configurations into the addon-storysource loader in your .storybook/main.js
file, e.g.:
export default {
addons: [
{
name: '@storybook/addon-storysource',
options: {
rule: {
// test: [/\.stories\.jsx?$/], This is default
include: [path.resolve(__dirname, '../src')], // You can specify directories
},
loaderOptions: {
prettierConfig: { printWidth: 80, singleQuote: false },
},
},
},
],
};
To customize the source-loader
, pass loaderOptions
. Valid configurations are documented in the source-loader
README.
Storysource will automatically use the light or dark syntax theme based on your storybook theme. See Theming Storybook for more information.
Storybook 6.0 introduced an unintentional change to source-loader
, in which only the source of the selected story is shown in the addon. To restore the old behavior, pass theinjectStoryParameters: false
option.
If you're using addon-docs
:
export default {
addons: [
{
name: '@storybook/addon-docs',
options: {
sourceLoaderOptions: {
injectStoryParameters: false,
},
},
},
],
};
If not:
export default {
addons: [
{
name: '@storybook/addon-storysource',
options: {
loaderOptions: {
injectStoryParameters: false,
},
},
},
],
};
This bug will be resolved in a future version of the addon.
7.0.0 (March 31, 2023)
Storybook 7.0 is here! 🎉
7.0 contains hundreds more fixes, features, and tweaks. Browse the changelogs matching 7.0.0-alpha.*
, 7.0.0-beta.*
, and 7.0.0-rc.*
for the full list of changes.
See our Migration guide to upgrade from earlier versions of Storybook.
Full announcement and proper release to the latest
npm tag coming soon. 😘
FAQs
View a story’s source code to see how it works and paste into your app
The npm package @storybook/addon-storysource receives a total of 491,864 weekly downloads. As such, @storybook/addon-storysource popularity was classified as popular.
We found that @storybook/addon-storysource demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 12 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.