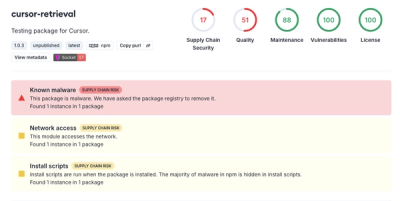
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
@strapi/helper-plugin
Advanced tools
Helper for Strapi plugins development
@strapi/helper-plugin is a utility package designed to assist in the development of Strapi plugins. It provides a set of tools and components that streamline the process of creating and managing plugins within the Strapi ecosystem.
API Requests
This feature allows you to make API requests easily within your Strapi plugin. The `request` function simplifies the process of sending HTTP requests and handling responses.
import { request } from '@strapi/helper-plugin';
const fetchData = async () => {
try {
const data = await request('/some-endpoint', { method: 'GET' });
console.log(data);
} catch (error) {
console.error('Error fetching data:', error);
}
};
fetchData();
Notification System
The notification system allows you to display notifications within your plugin. The `useNotification` hook provides a simple way to trigger notifications with different types and messages.
import { useNotification } from '@strapi/helper-plugin';
const MyComponent = () => {
const toggleNotification = useNotification();
const handleClick = () => {
toggleNotification({ type: 'success', message: 'Operation successful!' });
};
return <button onClick={handleClick}>Click me</button>;
};
Form Handling
Form handling is made easier with the `useFormik` hook from @strapi/helper-plugin. It helps manage form state, validation, and submission.
import { useFormik } from '@strapi/helper-plugin';
const MyForm = () => {
const formik = useFormik({
initialValues: { name: '' },
onSubmit: values => {
console.log('Form data:', values);
},
});
return (
<form onSubmit={formik.handleSubmit}>
<input
type="text"
name="name"
value={formik.values.name}
onChange={formik.handleChange}
/>
<button type="submit">Submit</button>
</form>
);
};
Formik is a popular library for building and managing forms in React. It provides a comprehensive set of tools for handling form state, validation, and submission. Compared to @strapi/helper-plugin, Formik is more general-purpose and can be used in any React application, not just Strapi plugins.
Axios is a widely-used HTTP client for making API requests in JavaScript. It offers a simple and intuitive API for sending HTTP requests and handling responses. While @strapi/helper-plugin's `request` function is tailored for Strapi, Axios can be used in any JavaScript project.
React-Toastify is a library for displaying notifications in React applications. It provides a flexible and customizable notification system. Compared to @strapi/helper-plugin's notification system, React-Toastify offers more customization options and can be used in any React project.
[!WARNING] This package is only compatible with Strapi v4 version
The most recent versions of Strapi packages can be found at https://github.com/strapi/strapi
Helper to develop Strapi plugins.
Please read our Contributing Guide before submitting a Pull Request to the project.
FAQs
Helper for Strapi plugins development
The npm package @strapi/helper-plugin receives a total of 123,710 weekly downloads. As such, @strapi/helper-plugin popularity was classified as popular.
We found that @strapi/helper-plugin demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.