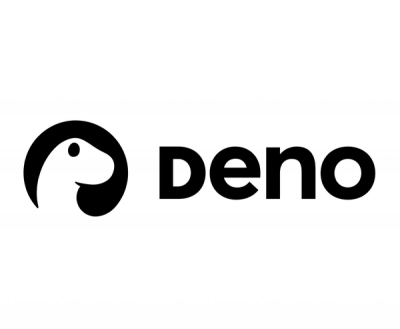
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@tensorflow/tfjs
Advanced tools
@tensorflow/tfjs is an open-source hardware-accelerated JavaScript library for training and deploying machine learning models. It allows you to develop machine learning models in JavaScript and use them in the browser or on Node.js.
Creating and Training Models
This code demonstrates how to create a simple neural network model, compile it, and train it using synthetic data. The model is then used for inference.
const tf = require('@tensorflow/tfjs');
// Define a simple model
const model = tf.sequential();
model.add(tf.layers.dense({units: 100, activation: 'relu', inputShape: [10]}));
model.add(tf.layers.dense({units: 1, activation: 'linear'}));
// Compile the model
model.compile({optimizer: 'sgd', loss: 'meanSquaredError'});
// Generate some synthetic data for training
const xs = tf.randomNormal([100, 10]);
const ys = tf.randomNormal([100, 1]);
// Train the model
model.fit(xs, ys, {epochs: 10}).then(() => {
// Use the model for inference
model.predict(tf.randomNormal([1, 10])).print();
});
Loading Pre-trained Models
This code demonstrates how to load a pre-trained model from a URL and use it for inference on an image. The image is preprocessed to match the input requirements of the model.
const tf = require('@tensorflow/tfjs');
// Load a pre-trained model from a URL
const modelUrl = 'https://storage.googleapis.com/tfjs-models/tfjs/mobilenet_v1_0.25_224/model.json';
tf.loadGraphModel(modelUrl).then(model => {
// Use the model for inference
const img = tf.browser.fromPixels(document.getElementById('image'));
const resizedImg = tf.image.resizeBilinear(img, [224, 224]);
const input = resizedImg.expandDims(0).toFloat().div(tf.scalar(127)).sub(tf.scalar(1));
model.predict(input).print();
});
Tensor Operations
This code demonstrates basic tensor operations such as addition. Tensors are the core data structure in TensorFlow.js, and you can perform various mathematical operations on them.
const tf = require('@tensorflow/tfjs');
// Create tensors
const a = tf.tensor([1, 2, 3, 4]);
const b = tf.tensor([5, 6, 7, 8]);
// Perform tensor operations
const c = a.add(b);
c.print(); // Output: [6, 8, 10, 12]
Brain.js is a JavaScript library for neural networks. It is simpler and more beginner-friendly compared to TensorFlow.js, but it is less powerful and flexible. Brain.js is suitable for smaller projects and simpler neural network tasks.
Synaptic is a JavaScript neural network library for node.js and the browser. It provides a wide range of neural network architectures and is relatively easy to use. However, it does not offer the same level of performance and hardware acceleration as TensorFlow.js.
ml5.js is a high-level library built on top of TensorFlow.js, designed to make machine learning accessible to artists, creative coders, and students. It provides a simplified API for common machine learning tasks, making it easier to use but less flexible for advanced users.
TensorFlow.js is an open-source hardware-accelerated JavaScript library for building, training and serving machine learning models. When running in the browser, it utilizes WebGL acceleration. TensorFlow.js is also convenient and intuitive, modeled after Keras and tf.layers and can load models saved from those libraries.
This repository conveniently contains the logic and scripts to form a version-matched union package, @tensorflowjs/tfjs, from
You can import TensorFlow.js Union directly via yarn or npm.
yarn add @tensorflow/tfjs
or npm install @tensorflow/tfjs
.
See snippets below for examples.
Alternatively you can use a script tag. Here we load it from a CDN.
In this case it will be available as a global variable named tf
.
You can replace also specify which version to load replacing @latest
with a specific
version string (e.g. 0.6.0
).
<script src="https://cdn.jsdelivr.net/npm/tensorflow/tfjs@latest"></script>
<!-- or -->
<script src="https://unpkg.com/tensorflow/tfjs@latest"></script>
Many examples illustrating how to use TensorFlow.js in ES5, ES6 and TypeScript are available from the Examples repository and the TensorFlow.js Tutorials
Let's add a scalar value to a 1D Tensor. TensorFlow.js supports broadcasting the value of scalar over all the elements in the tensor.
import * as tf from '@tensorflow/tfjs'; // If not loading the script as a global
const a = tf.tensor1d([1, 2, 3]);
const b = tf.scalar(2);
const result = a.add(b); // a is not modified, result is a new tensor
result.data().then(data => console.log(data)); // Float32Array([3, 4, 5]
// Alternatively you can use a blocking call to get the data.
// However this might slow your program down if called repeatedly.
console.log(result.dataSync()); // Float32Array([3, 4, 5]
See the core-concepts tutorial for more.
The following example shows how to build a toy model with only one dense
layer
to perform linear regression.
import * as tf from '@tensorflow/tfjs';
// A sequential model is a container which you can add layers to.
const model = tf.sequential();
// Add a dense layer with 1 output unit.
model.add(tf.layers.dense({units: 1, inputShape: [1]}));
// Specify the loss type and optimizer for training.
model.compile({loss: 'meanSquaredError', optimizer: 'SGD'});
// Generate some synthetic data for training.
const xs = tf.tensor2d([[1], [2], [3], [4]], [4, 1]);
const ys = tf.tensor2d([[1], [3], [5], [7]], [4, 1]);
// Train the model.
await model.fit(xs, ys, {epochs: 500});
// Ater the training, perform inference.
const output = model.predict(tf.tensor2d([[5]], [1, 1]));
output.print();
For a deeper dive into building a layers classifier, see the MNIST tutorial
You can also load a model previously trained and saved from elsewhere (e.g., from Python Keras) and use it for inference or transfer learning in the browser. More details in the import-keras tutorial
For example, in Python, save your Keras model using
tensorflowjs,
which can be installed using pip install tensorflowjs
.
import tensorflowjs as tfjs
# ... Create and train your Keras model.
# Save your Keras model in TensorFlow.js format.
tfjs.converter.save_keras_model(model, '/path/to/tfjs_artifacts/')
# Then use your favorite web server to serve the directory at a URL, say
# http://foo.bar/tfjs_artifacts/model.json
To load the model with TensorFlow.js Layers:
import * as tf from '@tensorflow/tfjs';
const model = await tf.loadModel('http://foo.bar/tfjs_artifacts/model.json');
// Now the model is ready for inference, evaluation or re-training.
Again, see the Examples repository and the TensorFlow.js Tutorials for many more examples of how to build models and manipulate tensors.
TensorFlow.js targets environments with WebGL 1.0 or WebGL 2.0. For devices
without the OES_texture_float
extension, we fall back to fixed precision
floats backed by a gl.UNSIGNED_BYTE
texture. For platforms without WebGL,
we provide CPU fallbacks.
TensorFlow.js is a part of the TensorFlow ecosystem. You can import pre-trained TensorFlow SavedModels and Keras models, for execution and retraining.
For more information on the API, follow the links to their Core and Layers repositories below, or visit js.tensorflow.org.
FAQs
An open-source machine learning framework.
The npm package @tensorflow/tfjs receives a total of 121,183 weekly downloads. As such, @tensorflow/tfjs popularity was classified as popular.
We found that @tensorflow/tfjs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 10 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.