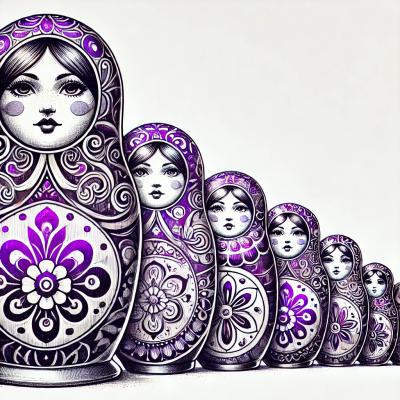
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
@testing-library/react-hooks
Advanced tools
Simple and complete React hooks testing utilities that encourage good testing practices.
The @testing-library/react-hooks package is designed to facilitate the testing of custom React hooks. It provides a simple and complete set of utilities that work well with the React Testing Library ecosystem. With this package, you can render hooks in isolation without having to deal with components, and you can test their behavior, state changes, and side effects.
Rendering hooks and testing their initial state
This feature allows you to render a custom hook and assert its initial state. The `renderHook` function is used to render the hook, and the `result` object is used to access the hook's return values.
import { renderHook } from '@testing-library/react-hooks';
function useCustomHook() {
const [value, setValue] = useState(0);
return { value, setValue };
}
test('should start with initial value', () => {
const { result } = renderHook(() => useCustomHook());
expect(result.current.value).toBe(0);
});
Testing hook updates
This feature allows you to test the updates of a hook's state. The `act` function is used to wrap any code that triggers updates to the hook.
import { renderHook, act } from '@testing-library/react-hooks';
function useCounter() {
const [count, setCount] = useState(0);
return { count, increment: () => setCount(c => c + 1) };
}
test('should increment counter', () => {
const { result } = renderHook(() => useCounter());
act(() => {
result.current.increment();
});
expect(result.current.count).toBe(1);
});
Testing asynchronous hooks
This feature is for testing hooks that have asynchronous operations, such as data fetching. The `waitForNextUpdate` function is used to wait for the hook to update after the asynchronous operation.
import { renderHook } from '@testing-library/react-hooks';
function useFetch(url) {
const [data, setData] = useState(null);
useEffect(() => {
fetch(url).then(response => response.json()).then(setData);
}, [url]);
return data;
}
test('should fetch data', async () => {
const { result, waitForNextUpdate } = renderHook(() => useFetch('https://api.example.com/data'));
await waitForNextUpdate();
expect(result.current).not.toBeNull();
});
Enzyme is a JavaScript testing utility for React that makes it easier to assert, manipulate, and traverse your React Components' output. It does not provide a dedicated API for testing hooks in isolation, which is a key difference from @testing-library/react-hooks.
React Test Renderer is an official React package that provides a renderer that can be used to render React components to pure JavaScript objects, without depending on the DOM or a native mobile environment. It is more general-purpose compared to @testing-library/react-hooks, which is specifically tailored for testing React hooks.
Simple and complete React hooks testing utilities that encourage good testing practices.
You're writing an awesome custom hook and you want to test it, but as soon as you call it you see the following error:
Invariant Violation: Hooks can only be called inside the body of a function component.
You don't really want to write a component solely for testing this hook and have to work out how you were going to trigger all the various ways the hook can be updated, especially given the complexities of how you've wired the whole thing together.
The react-hooks-testing-library
allows you to create a simple test harness for React hooks that
handles running them within the body of a function component, as well as providing various useful
utility functions for updating the inputs and retrieving the outputs of your amazing custom hook.
This library aims to provide a testing experience as close as possible to natively using your hook
from within a real component.
Using this library, you do not have to concern yourself with how to construct, render or interact with the react component in order to test your hook. You can just use the hook directly and assert the results.
useCounter.js
import { useState, useCallback } from 'react'
function useCounter() {
const [count, setCount] = useState(0)
const increment = useCallback(() => setCount((x) => x + 1), [])
return { count, increment }
}
export default useCounter
useCounter.test.js
import { renderHook, act } from '@testing-library/react-hooks'
import useCounter from './useCounter'
test('should increment counter', () => {
const { result } = renderHook(() => useCounter())
act(() => {
result.current.increment()
})
expect(result.current.count).toBe(1)
})
More advanced usage can be found in the documentation.
npm install --save-dev @testing-library/react-hooks
react-hooks-testing-library
does not come bundled with a version of
react
or
react-test-renderer
to allow you to install
the specific version you want to test against. Generally, the installed versions for react
and
react-test-renderer
should have matching versions:
npm install react@^16.9.0
npm install --save-dev react-test-renderer@^16.9.0
NOTE: The minimum supported version of
react
andreact-test-renderer
is^16.9.0
.
See the API reference.
Thanks goes to these wonderful people (emoji key):
This project follows the all-contributors specification. Contributions of any kind welcome!
Looking to contribute? Look for the Good First Issue label.
Please file an issue for bugs, missing documentation, or unexpected behavior.
Please file an issue to suggest new features. Vote on feature requests by adding a 👍. This helps maintainers prioritize what to work on.
For questions related to using the library, you can raise issue here, or visit a support community:
MIT
FAQs
Simple and complete React hooks testing utilities that encourage good testing practices.
We found that @testing-library/react-hooks demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 13 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.