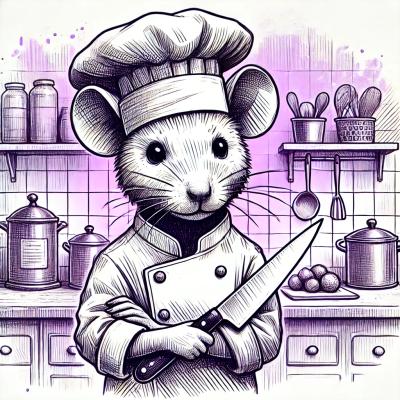
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@timetac/js-client-library
Advanced tools
This library is a thin wrapper facilitating requests to the TimeTac REST API. For more information please visit the API documentation.
Use the package manager yarn or npm to install TimeTac Client Library.
Using yarn:
yarn add @timetac/js-client-library
Using npm:
npm install @timetac/js-client-library
Existing customers can activate API access or request access to a sandbox environment by contacting support@timetac.com. This process usually takes less than two business days and is currently free of charge.
If you are not a customer yet, you may set up a
free trial and contact
support@timetac.com to obtain a client_id
and client_secret
for your
personal demo account.
Finally, if you just want to run a few requests, you may use the public API
playground
sandbox account. This account is reset to its initial state in
regular intervals. Access credentials are available in the react playground's
README.
import Api from "@timetac/js-client-library"
//Only account name is required.
const environment = {
host: 'go.timetac.com',
https?: boolean;
account: <ACCOUNT_NAME>,
version: 3,
account?: string;
accessToken?: string;
refreshToken?: string;
clientId?: string;
clientSecret?: string;
//Callback, called on refresh of the token. object of access token and refresh {accessToken, refreshTOken} token are passed as parameter
onTokenRefreshedCallback: (tokens) => console.log(`${tokens.accessToken} ${tokens.refreshToken}`),
//Callback called when refresh of the token fails.
onTokenRefreshFailed: () => { console.log('Intended action, such as logout')},
//If true, it tries to refresh token on failed request. Default true.
shouldAutoRefreshToken?: boolean;
timeout?: number;
}
const authCredentials = {
grant_type: 'password',
client_id: <CLIENT_ID>,
client_secret: <CLIENT_SECRET>,
username: <USER_NAME>,
password: <PASSWORD>
}
const api = new Api(environment);
async() => {
await api.authentication.login(authCredentials);
api.timeTrackings.read()
.then({ Results }} => {
console.log(Results)
});
api.users.readMe().then({ Results } => {
console.log(Results);
});
api.absenceDays.read(
new RequestParamsBuilder<AbsenceDay>()
.eq('user_id', 1)
.gteq('date', '2020-01-01')
.build()
);
api.absenceDay.read({
user_id: '1',
date: '2020-01-01',
_op__date: 'gteq'
})
}
Pull requests are welcome. For major changes, please open an issue first to discuss what you would like to change.
The shortest, simplest way of running the test suite is the following command:
yarn test
When experiencing issues with cross-origin resource sharing (CORS), you will have to configure a proxy server for development. This can be done, for example, with http-proxy-middleware. An example configuration for a React project can be found in the react playground.
FAQs
TimeTac API JS client library
The npm package @timetac/js-client-library receives a total of 1,648 weekly downloads. As such, @timetac/js-client-library popularity was classified as popular.
We found that @timetac/js-client-library demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.