React Flow Smart Edge
Special Edge for React Flow that never intersects with other nodes.
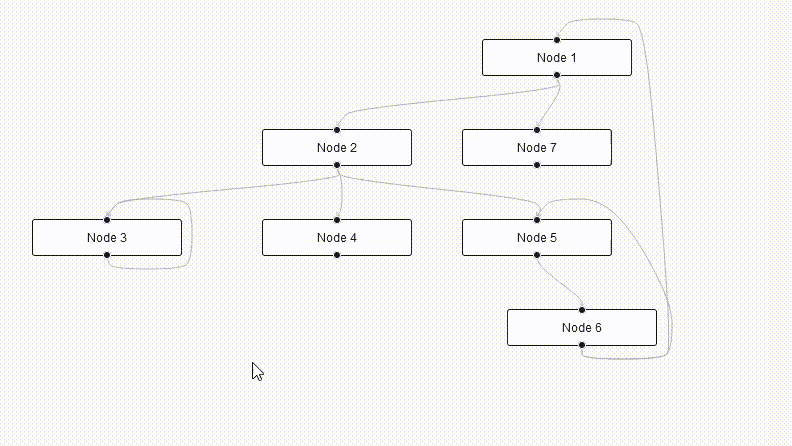
Install
npm install @tisoap/react-flow-smart-edge
Usage
import React from 'react';
import ReactFlow from 'react-flow-renderer';
import { SmartEdge } from '@tisoap/react-flow-smart-edge';
const elements = [
{
id: '1',
data: { label: 'Node 1' },
position: { x: 300, y: 100 },
},
{
id: '2',
data: { label: 'Node 2' },
position: { x: 300, y: 200 },
},
{
id: 'e21',
source: '2',
target: '1',
type: 'smart',
},
];
export const Graph = (props) => {
const { children, ...rest } = props;
return (
<ReactFlow
elements={elements}
edgeTypes={{
smart: SmartEdge,
}}
{...rest}
>
{children}
</ReactFlow>
);
};
Options
The SmartEdge
takes the same options as a React Flow Edge.
You can configure additional options wrapping your graph with SmartEdgeProvider
and passing an options value
. The available options are:
const options = {
debounceTime: 100,
};
Usage:
import React from 'react';
import ReactFlow from 'react-flow-renderer';
import { SmartEdge, SmartEdgeProvider } from '@tisoap/react-flow-smart-edge';
import elements from './elements';
export const Graph = (props) => {
const { children, ...rest } = props;
return (
<SmartEdgeProvider value={{ debounceTime: 300 }}>
<ReactFlow
elements={elements}
edgeTypes={{
smart: SmartEdge,
}}
{...rest}
>
{children}
</ReactFlow>
</SmartEdgeProvider>
);
};
Example
There is a minimum example in this repository example
folder. Clone this repository and run yarn; cd example; yarn; yarn start
.
you can also see the Storybook examples or interact with the stories yourself by cloning this repository and running yarn; yarn storybook
.
License
This project is MIT licensed.