React Flow Smart Edge
Custom Edges for React Flow that never intersect with other nodes.
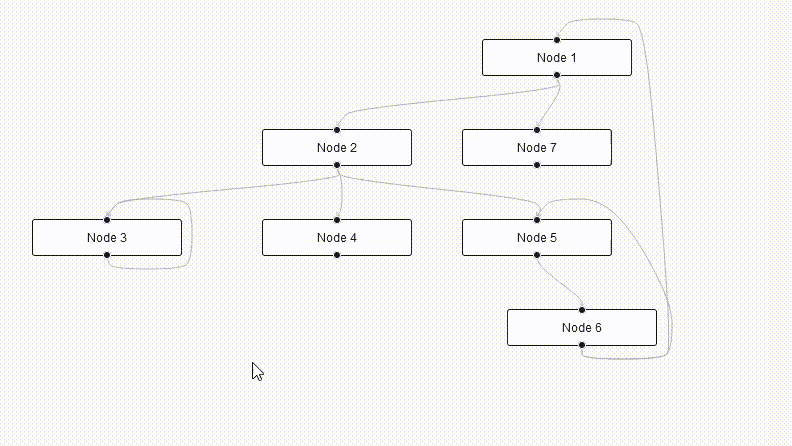
Install
With npm
:
npm install @tisoap/react-flow-smart-edge
With yarn
:
yarn add @tisoap/react-flow-smart-edge
Usage
import React from 'react'
import ReactFlow from 'react-flow-renderer'
import { SmartEdge } from '@tisoap/react-flow-smart-edge'
const nodes = [
{
id: '1',
data: { label: 'Node 1' },
position: { x: 300, y: 100 }
},
{
id: '2',
data: { label: 'Node 2' },
position: { x: 300, y: 200 }
}
]
const edges = [
{
id: 'e21',
source: '2',
target: '1',
type: 'smart'
}
]
const edgeTypes = {
smart: SmartEdge
}
export const Graph = (props) => {
const { children, ...rest } = props
return (
<ReactFlow
defaultNodes={nodes}
defaultEdges={edges}
edgeTypes={edgeTypes}
{...rest}
>
{children}
</ReactFlow>
)
}
Options
The SmartEdge
takes the same options as a React Flow Edge.
You can configure additional advanced options by wrapping your graph with SmartEdgeProvider
and passing an options
object. If an option is not provided it'll assume it's default value. The available options are:
const options = {
debounceTime: 200,
nodePadding: 10,
gridRatio: 10,
lineType: 'curve',
lessCorners: false
}
Usage:
import React from 'react'
import ReactFlow from 'react-flow-renderer'
import { SmartEdge, SmartEdgeProvider } from '@tisoap/react-flow-smart-edge'
import { nodes, edges } from './data'
const edgeTypes = {
smart: SmartEdge
}
const smartOptions = {
debounceTime: 300
}
export const Graph = (props) => {
const { children, ...rest } = props
return (
<SmartEdgeProvider options={smartOptions}>
<ReactFlow
defaultNodes={nodes}
defaultEdges={edges}
edgeTypes={edgeTypes}
{...rest}
>
{children}
</ReactFlow>
</SmartEdgeProvider>
)
}
Storybook
You can see Storybook examples by visiting this page: https://tisoap.github.io/react-flow-smart-edge/
License
This project is MIT licensed.
Support
Liked this project and want to show your support? Buy me a coffee:
