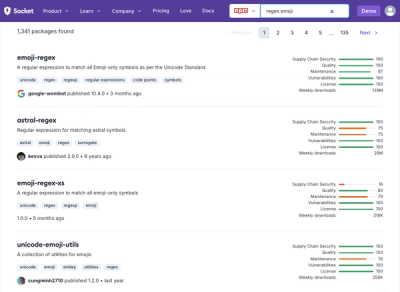
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
@travetto/base
Advanced tools
Install: @travetto/base
npm install @travetto/base
# or
yarn add @travetto/base
Base is the foundation of all Travetto applications. It is intended to be a minimal application set, as well as support for commonly shared functionality. It has support for the following key areas:
The functionality we support for testing and retrieving environment information:
isTrue(key: string): boolean;
- Test whether or not an environment flag is set and is trueisFalse(key: string): boolean;
- Test whether or not an environment flag is set and is falseisSet(key:string): boolean;
- Test whether or not an environment value is set (excludes: null
, ''
, and undefined
)get(key: string, def?: string): string;
- Retrieve an environmental value with a potential defaultgetBoolean(key: string, isValue?: boolean)
- Retrieve an environmental value as a boolean. If isValue is provided, determine if the environment variable matches the specified valuegetInt(key: string, def?: number): number;
- Retrieve an environmental value as a numbergetList(key: string): string[];
- Retrieve an environmental value as a listaddToList(key: string, value: string): string[];
- Add an item to an environment value, ensuring uniquenessGlobalEnv is a non-cached interface to the Env class with specific patterns defined. It provides access to common patterns used at runtime within the framework.
Code: GlobalEnv Shape
export const GlobalEnv = {
/** Get environment name */
get envName(): string;
/** Get debug module expression */
get debug(): string | undefined;
/** Are we in development mode */
get devMode(): boolean;
/** Is the app in dynamic mode? */
get dynamic(): boolean;
/** Get list of resource paths */
get resourcePaths(): string[];
/** Is test */
get test(): boolean;
/** Get node major version */
get nodeVersion(): number;
/** Export as plain object */
toJSON(): Record<string, unknown>;
} as const;
The source for each field is:
envName
- This is derived from process.env.TRV_ENV
with a fallback of process.env.NODE_ENV
devMode
- This is true if process.env.NODE_ENV
is dev* or testdynamic
- This is derived from process.env.TRV_DYNAMIC
. This field reflects certain feature sets used throughout the framework.resourcePaths
- This is a list derived from process.env.TRV_RESOURCES
. This points to a list of folders that the ResourceLoader will search against.test
- This is true if envName
is test
nodeVersion
- This is derived from process.version
, and is used primarily for logging purposes
In addition to reading these values, there is a defined method for setting/updating these values:Code: GlobalEnv Update
export function defineEnv(cfg: EnvInit = {}): void {
const envName = (cfg.envName ?? readEnvName()).toLowerCase();
process.env.NODE_ENV = /^dev|development|test$/.test(envName) ? 'development' : 'production';
process.env.DEBUG = `${envName !== 'test' && (cfg.debug ?? GlobalEnv.debug ?? false)}`;
process.env.TRV_ENV = envName;
process.env.TRV_DYNAMIC = `${cfg.dynamic ?? GlobalEnv.dynamic}`;
}
As you can see this method exists to update/change the process.env
values so that the usage of GlobalEnv reflects these changes. This is primarily used in testing, or custom environment setups (e.g. CLI invocations for specific applications).
The primary access patterns for resources, is to directly request a file, and to resolve that file either via file-system look up or leveraging the Manifest's data for what resources were found at manifesting time.
The FileLoader allows for accessing information about the resources, and subsequently reading the file as text/binary or to access the resource as a Readable
stream. If a file is not found, it will throw an AppError with a category of 'notfound'.
The ResourceLoader extends FileLoader and utilizes the GlobalEnv's resourcePaths
information on where to attempt to find a requested resource.
While the framework is 100 % compatible with standard Error
instances, there are cases in which additional functionality is desired. Within the framework we use AppError (or its derivatives) to represent framework errors. This class is available for use in your own projects. Some of the additional benefits of using this class is enhanced error reporting, as well as better integration with other modules (e.g. the RESTful API module and HTTP status codes).
The AppError takes in a message, and an optional payload and / or error classification. The currently supported error classifications are:
general
- General purpose errorssystem
- Synonym for general
data
- Data format, content, etc are incorrect. Generally correlated to bad input.permission
- Operation failed due to lack of permissionsauth
- Operation failed due to lack of authenticationmissing
- Resource was not found when requestedtimeout
- Operation did not finish in a timely mannerunavailable
- Resource was unresponsiveThis module provides logging functionality, built upon console operations.
The supported operations are:
console.error
which logs at the ERROR
levelconsole.warn
which logs at the WARN
levelconsole.info
which logs at the INFO
levelconsole.debug
which logs at the DEBUG
levelconsole.log
which logs at the INFO
levelNote: All other console methods are excluded, specifically trace
, inspect
, dir
, time
/timeEnd
All of the logging instrumentation occurs at transpilation time. All console.*
methods are replaced with a call to a globally defined variable that delegates to the ConsoleManager. This module, hooks into the ConsoleManager and receives all logging events from all files compiled by the Travetto.
A sample of the instrumentation would be:
Code: Sample logging at various levels
export function work() {
console.debug('Start Work');
try {
1 / 0;
} catch (err) {
console.error('Divide by zero', { error: err });
}
console.debug('End Work');
}
Code: Sample After Transpilation
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
exports.work = void 0;
const tslib_1 = require("tslib");
const ᚕ_c = tslib_1.__importStar(require("@travetto/base/src/console.js"));
var ᚕf = "@travetto/base/doc/transpile.js";
function work() {
ᚕ_c.log({ level: "debug", source: ᚕf, line: 2, scope: "work", args: ['Start Work'] });
try {
1 / 0;
}
catch (err) {
ᚕ_c.log({ level: "error", source: ᚕf, line: 7, scope: "work", args: ['Divide by zero', { error: err }] });
}
ᚕ_c.log({ level: "debug", source: ᚕf, line: 9, scope: "work", args: ['End Work'] });
}
exports.work = work;
The debug
messages can be filtered using the patterns from the debug. You can specify wild cards to only DEBUG
specific modules, folders or files. You can specify multiple, and you can also add negations to exclude specific packages.
Terminal: Sample environment flags
# Debug
$ DEBUG=-@travetto/model npx trv run app
$ DEBUG=-@travetto/registry npx trv run app
$ DEBUG=@travetto/rest npx trv run app
$ DEBUG=@travetto/*,-@travetto/model npx trv run app
Additionally, the logging framework will merge debug into the output stream, and supports the standard usage
Terminal: Sample environment flags for standard usage
# Debug
$ DEBUG=express:*,@travetto/rest npx trv run rest
The StreamUtil class provides basic stream utilities for use within the framework:
toBuffer(src: Readable | Buffer | string): Promise<Buffer>
for converting a stream/buffer/filepath to a Buffer.toReadable(src: Readable | Buffer | string):Promise<Readable>
for converting a stream/buffer/filepath to a ReadablewriteToFile(src: Readable, out: string):Promise<void>
will stream a readable into a file path, and wait for completion.waitForCompletion(src: Readable, finish:()=>Promise<any>)
will ensure the stream remains open until the promise finish produces is satisfied.Simple functions for providing a minimal facsimile to lodash, but without all the weight. Currently ObjectUtil includes:
isPrimitive(el)
determines if el
is a string
, boolean
, number
or RegExp
isPlainObject(obj)
determines if the obj is a simple objectisFunction(o)
determines if o
is a simple Function
isClass(o)
determines if o
is a class constructorisSimple(a)
determines if a
is a simple valueisPromise(a)
determines if a
is a promiseData utilities for binding values, and type conversion. Currently DataUtil includes:
deepAssign(a, b, mode ?)
which allows for deep assignment of b
onto a
, the mode
determines how aggressive the assignment is, and how flexible it is. mode
can have any of the following values:
loose
, which is the default is the most lenient. It will not error out, and overwrites will always happencoerce
, will attempt to force values from b
to fit the types of a
, and if it can't it will error outstrict
, will error out if the types do not matchcoerceType(input: unknown, type: Class<unknown>, strict = true)
which allows for converting an input type into a specified type
instance, or throw an error if the types are incompatible.
shallowClone<T = unknown>(a: T): T
will shallowly clone a field
filterByKeys<T>(obj: T, exclude: (string | RegExp)[]): T
will filter a given object, and return a plain object (if applicable) with fields excluded using the values in the exclude
input
Common utilities used throughout the framework. Currently Util includes:
uuid(len: number)
generates a simple uuid for use within the application.allowDenyMatcher(rules[])
builds a matching function that leverages the rules as an allow/deny list, where order of the rules matters. Negative rules are prefixed by '!'.naiveHash(text: string)
produces a fast, and simplistic hash. No guarantees are made, but performs more than adequately for framework purposes.makeTemplate<T extends string>(wrap: (key: T, val: TemplatePrim) => string)
produces a template function tied to the distinct string values that key
supports.resolvablePromise()
produces a Promise
instance with the resolve
and reject
methods attached to the instance. This is extremely useful for integrating promises into async iterations, or any other situation in which the promise creation and the execution flow don't always match up.Code: Sample makeTemplate Usage
const tpl = makeTemplate((name: 'age'|'name', val) => `**${name}: ${val}**`);
tpl`{{age:20}} {{name: 'bob'}}</>;
// produces
'**age: 20** **name: bob**'
TimeUtil contains general helper methods, created to assist with time-based inputs via environment variables, command line interfaces, and other string-heavy based input.
Code: Time Utilities
export class TimeUtil {
/**
* Test to see if a string is valid for relative time
* @param val
*/
static isTimeSpan(val: string): val is TimeSpan;
/**
* Returns time units convert to ms
* @param amount Number of units to extend
* @param unit Time unit to extend ('ms', 's', 'm', 'h', 'd', 'w', 'y')
*/
static timeToMs(amount: number | TimeSpan, unit?: TimeUnit): number;
/**
* Returns a new date with `amount` units into the future
* @param amount Number of units to extend
* @param unit Time unit to extend ('ms', 's', 'm', 'h', 'd', 'w', 'y')
*/
static timeFromNow(amount: number | TimeSpan, unit: TimeUnit = 'ms'): Date;
/**
* Wait for 'amount' units of time
*/
static wait(amount: number | TimeSpan, unit: TimeUnit = 'ms'): Promise<void>;
/**
* Get environment variable as time
* @param key env key
* @param def backup value if not valid or found
*/
static getEnvTime(key: string, def?: number | TimeSpan): number;
/**
* Pretty print a delta between now and `time`, with auto-detection of largest unit
*/
static prettyDeltaSinceTime(time: number, unit?: TimeUnit): string;
/**
* Pretty print a delta, with auto-detection of largest unit
* @param delta The number of milliseconds in the delta
*/
static prettyDelta(delta: number, unit?: TimeUnit): string;
}
Just like child_process, the ExecUtil exposes spawn
and fork
. These are generally wrappers around the underlying functionality. In addition to the base functionality, each of those functions is converted to a Promise
structure, that throws an error on an non-zero return status.
A simple example would be:
Code: Running a directory listing via ls
import { ExecUtil } from '@travetto/base';
export async function executeListing() {
const { result } = ExecUtil.spawn('ls');
const final = await result;
console.log('Listing', { lines: final.stdout.split('\n') });
}
As you can see, the call returns not only the child process information, but the Promise
to wait for. Additionally, some common patterns are provided for the default construction of the child process. In addition to the standard options for running child processes, the module allows for the following execution options:
Code: Execution Options
export interface ExecutionOptions extends SpawnOptions {
/**
* Should an error be caught and returned as a result. Determines whether an exit code > 0 throws
* an Error, or if it merely marks the process as completed, marking the result as invalid.
*/
catchAsResult?: boolean;
/**
* Should the environment be isolated, or inherit from process.env
*/
isolatedEnv?: boolean;
/**
* Built in timeout for any execution. The number of milliseconds the process can run before
* terminating and throwing an error
*/
timeout?: number;
/**
* Determines how to treat the stdout/stderr data.
* - 'text' will assume the output streams are textual, and will convert to unicode data.
* - 'text-stream' makes the same assumptions as 'text', but will only fire events, and will
* not persist any data. This is really useful for long running programs.
* - 'binary' treats all stdout/stderr data as raw buffers, and will not perform any transformations.
* - 'raw' avoids touching stdout/stderr altogether, and leaves it up to the caller to decide.
*/
outputMode?: 'raw' | 'binary' | 'text' | 'text-stream';
/**
* On stderr line. Requires 'outputMode' to be either 'text' or 'text-stream'
*/
onStdErrorLine?: (line: string) => void;
/**
* On stdout line. Requires 'outputMode' to be either 'text' or 'text-stream'
*/
onStdOutLine?: (line: string) => void;
/**
* The stdin source for the execution
*/
stdin?: string | Buffer | Readable;
}
Another key lifecycle is the process of shutting down. The framework provides centralized functionality for running operations on shutdown. Primarily used by the framework for cleanup operations, this provides a clean interface for registering shutdown handlers. The code overrides process.exit
to properly handle SIGKILL
and SIGINT
, with a default threshold of 3 seconds. In the advent of a SIGTERM
signal, the code exits immediately without any cleanup.
As a registered shutdown handler, you can do.
Code: Registering a shutdown handler
import { ShutdownManager } from '@travetto/base';
export function registerShutdownHandler() {
ShutdownManager.onShutdown('handler-name', async () => {
// Do important work, the framework will wait until all async
// operations are completed before finishing shutdown
});
}
FAQs
Environment config and common utilities for travetto applications.
The npm package @travetto/base receives a total of 380 weekly downloads. As such, @travetto/base popularity was classified as not popular.
We found that @travetto/base demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.