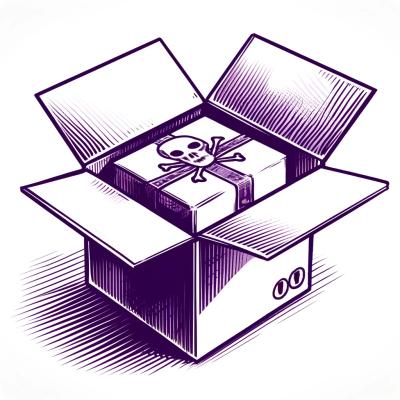
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
@types/argparse
Advanced tools
TypeScript definitions for argparse
The @types/argparse package provides TypeScript type definitions for the argparse package, which is a node.js library for parsing command-line options. It allows developers to add options, arguments, and sub-commands in their command-line applications, making it easier to build tools with clear and helpful usage messages. The types provided by @types/argparse ensure that TypeScript developers can use argparse with the benefits of type checks and autocompletion in their IDEs.
Argument Parsing
This feature allows you to parse command-line arguments. You can define options (like '-v' for verbose output) and then parse the arguments provided to your application. This is useful for creating command-line tools with various options.
{"import { ArgumentParser } from 'argparse';\nconst parser = new ArgumentParser({\n description: 'Argument parser example'\n});\n\nparser.add_argument('-v', '--verbose', {\n help: 'increase output verbosity',\n action: 'store_true'\n});\n\nconst args = parser.parse_args();\nconsole.log(args.verbose);"}
Sub-commands
This feature demonstrates how to use sub-commands in your application, allowing for a more organized and modular command-line interface. Each sub-command can have its own set of arguments.
{"import { ArgumentParser } from 'argparse';\nconst parser = new ArgumentParser({\n description: 'Sub-command example'\n});\n\nconst subparsers = parser.add_subparsers({\n title: 'subcommands',\n dest: 'subcommand_name'\n});\n\nconst start = subparsers.add_parser('start', { add_help: true });\nstart.add_argument('-p', '--port', {\n help: 'Port to start the server on',\n default: 8080\n});\n\nconst args = parser.parse_args();\nconsole.log(args);"}
Commander is a complete solution for node.js command-line interfaces, offering features like automated help generation, type coercion, and sub-commands. It is more high-level compared to argparse and does not require separate type definitions for TypeScript, as it includes its own.
Yargs helps you build interactive command-line tools, by parsing arguments and generating an elegant user interface. It supports a wide range of features like command chaining, argument validation, and context-based help. Yargs is similar to argparse but with a more fluent API and built-in support for TypeScript, eliminating the need for separate type definitions.
npm install --save @types/argparse
This package contains type definitions for argparse (https://github.com/nodeca/argparse).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/argparse.
These definitions were written by Andrew Schurman, Tomasz Łaziuk, Sebastian Silbermann, Kannan Goundan, Halvor Holsten Strand, and Dieter Oberkofler.
FAQs
TypeScript definitions for argparse
The npm package @types/argparse receives a total of 1,841,778 weekly downloads. As such, @types/argparse popularity was classified as popular.
We found that @types/argparse demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.