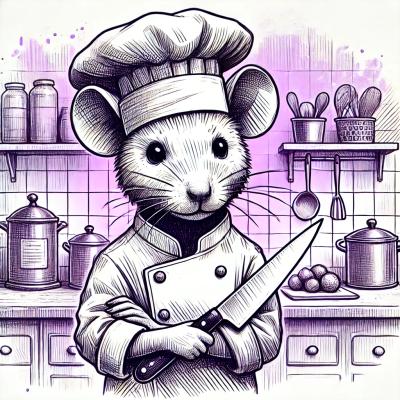
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@types/react-datepicker
Advanced tools
Stub TypeScript definitions entry for react-datepicker, which provides its own types definitions
The @types/react-datepicker package provides TypeScript definitions for the react-datepicker package. This allows developers using TypeScript to integrate the react-datepicker component into their projects with type checking, ensuring that props and options passed to the react-datepicker components are of the correct type. This enhances development experience by providing autocompletion and preventing common type-related errors.
Basic Date Selection
This feature allows users to select a single date. The DatePicker component is imported from react-datepicker, and its selected and onChange props are used to manage the selected date state.
import React, { useState } from 'react';
import DatePicker from 'react-datepicker';
import 'react-datepicker/dist/react-datepicker.css';
function App() {
const [startDate, setStartDate] = useState(new Date());
return (
<DatePicker selected={startDate} onChange={(date) => setStartDate(date)} />
);
}
Date Range Selection
This feature enables users to select a range of dates. The DatePicker component's selectsRange prop is set to true, and the startDate and endDate props are used to manage the range of selected dates.
import React, { useState } from 'react';
import DatePicker from 'react-datepicker';
import 'react-datepicker/dist/react-datepicker.css';
function App() {
const [startDate, setStartDate] = useState(new Date());
const [endDate, setEndDate] = useState(new Date());
return (
<DatePicker
selected={startDate}
onChange={(dates) => {
const [start, end] = dates;
setStartDate(start);
setEndDate(end);
}}
startDate={startDate}
endDate={endDate}
selectsRange
/>
);
}
Custom Date Format
This feature allows the display format of the selected date to be customized using the dateFormat prop.
import React, { useState } from 'react';
import DatePicker from 'react-datepicker';
import 'react-datepicker/dist/react-datepicker.css';
function App() {
const [startDate, setStartDate] = useState(new Date());
return (
<DatePicker
selected={startDate}
onChange={(date) => setStartDate(date)}
dateFormat='yyyy/MM/dd'
/>
);
}
react-dates is an Airbnb-developed date picker for React. It offers a range of features similar to react-datepicker, such as single date and date range selection. However, react-dates provides more built-in styles and customization options, making it a good choice for projects requiring more visually complex calendars.
material-ui-pickers offers date and time selection components that integrate well with Material-UI. It provides a similar functionality to react-datepicker but is designed to be used within Material-UI applications, offering a consistent look and feel with other Material-UI components.
The antd (Ant Design) library includes a DatePicker component that is part of a larger suite of UI components. While it offers similar date picking capabilities, it is designed to be used within the Ant Design ecosystem, providing a consistent design language and extensive customization options.
This is a stub types definition for @types/react-datepicker (https://github.com/Hacker0x01/react-datepicker).
react-datepicker provides its own type definitions, so you don't need @types/react-datepicker installed!
FAQs
Stub TypeScript definitions entry for react-datepicker, which provides its own types definitions
The npm package @types/react-datepicker receives a total of 885,448 weekly downloads. As such, @types/react-datepicker popularity was classified as popular.
We found that @types/react-datepicker demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.