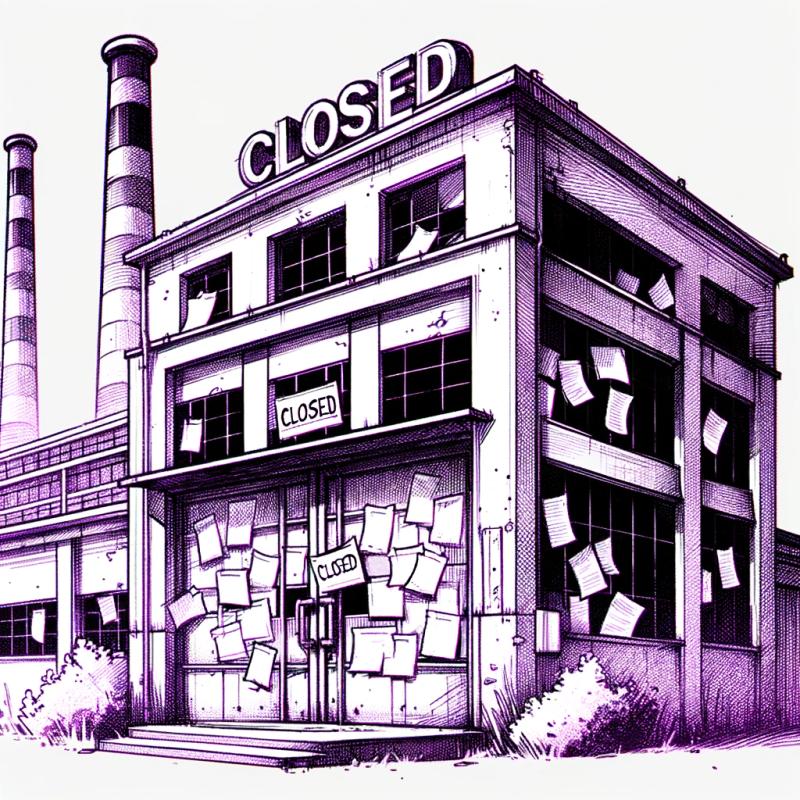
Security News
LDAPjs Open Source Project Decommissioned After Maintainer Receives Abusive Email
LDAPjs, an LDAP Client and Server API for Node.js, was decommissioned after its maintainer received an abusive email from a user, raising concerns about this form of abuse as a potential attack vector.