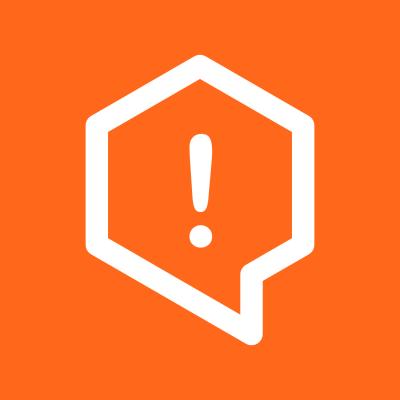
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@types/tmp
Advanced tools
TypeScript definitions for tmp
@types/tmp provides TypeScript type definitions for the 'tmp' npm package, which is used to create temporary files and directories in a Node.js environment.
Create a Temporary File
This feature allows you to create a temporary file. The callback provides the file path, file descriptor, and a cleanup callback to remove the file when done.
const tmp = require('tmp');
tmp.file((err, path, fd, cleanupCallback) => {
if (err) throw err;
console.log('File: ', path);
console.log('Filedescriptor: ', fd);
cleanupCallback();
});
Create a Temporary Directory
This feature allows you to create a temporary directory. The callback provides the directory path and a cleanup callback to remove the directory when done.
const tmp = require('tmp');
tmp.dir((err, path, cleanupCallback) => {
if (err) throw err;
console.log('Dir: ', path);
cleanupCallback();
});
Synchronous File Creation
This feature allows you to create a temporary file synchronously. The method returns an object with the file path and a remove callback.
const tmp = require('tmp');
const tmpobj = tmp.fileSync();
console.log('File: ', tmpobj.name);
tmpobj.removeCallback();
Synchronous Directory Creation
This feature allows you to create a temporary directory synchronously. The method returns an object with the directory path and a remove callback.
const tmp = require('tmp');
const tmpobj = tmp.dirSync();
console.log('Dir: ', tmpobj.name);
tmpobj.removeCallback();
fs-extra is a package that extends the native Node.js 'fs' module with additional methods, including methods for creating temporary files and directories. It provides more general file system utilities compared to 'tmp'.
temp is another package for creating temporary files and directories. It offers similar functionality to 'tmp' but with a different API. It also supports automatic cleanup of temporary files and directories.
npm install --save @types/tmp
This package contains type definitions for tmp (https://github.com/raszi/node-tmp).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/tmp.
These definitions were written by Jared Klopper, Gyusun Yeom, Alan Plum, Carsten Klein, and BendingBender.
FAQs
TypeScript definitions for tmp
The npm package @types/tmp receives a total of 2,539,081 weekly downloads. As such, @types/tmp popularity was classified as popular.
We found that @types/tmp demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.